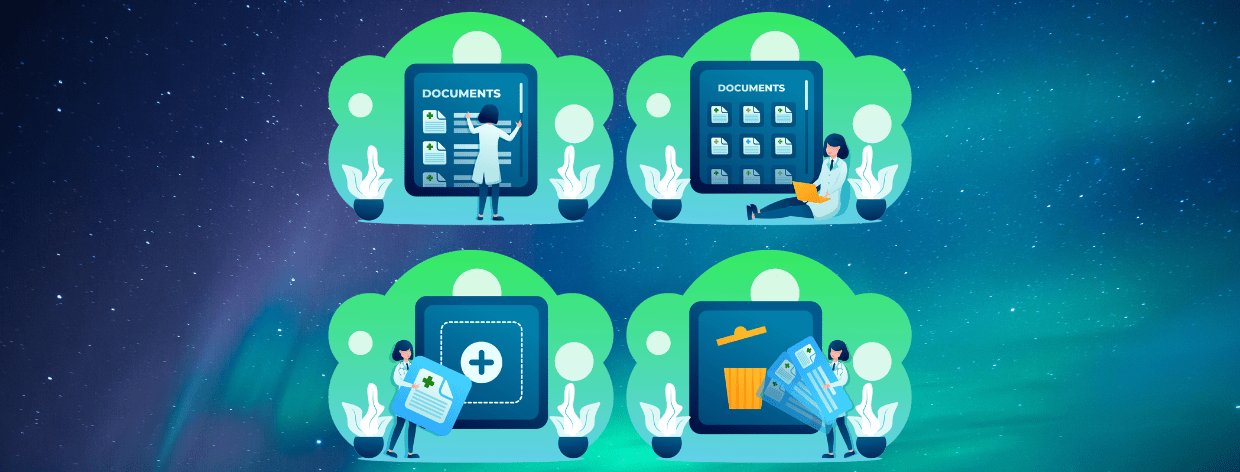
- 26th Feb 2024
- 16:24 pm
In programming, managing files efficiently is key. Deleting unwanted or outdated files is often necessary for various tasks, fostering clean data practices and optimizing storage space. Python, a versatile programming language, empowers you with several robust methods to delete files, each tailored to specific scenarios.
We delves into the essential methods available in Python for deleting files, equipping you with the knowledge to confidently manage your file system and effectively maintain your applications. Whether you need to remove temporary data, clear up logs, or comply with data regulations, understanding these methods will equip you to handle file deletion tasks elegantly and efficiently.
In Python programming, manipulating files is crucial. One essential task is file deletion, which removes unwanted or outdated files from the storage system. This action has diverse applications, ranging from:
- Data Cleaning: Removing temporary files generated during processing frees up storage space and maintains a tidy codebase.
- Log Management: Clearing outdated logs helps optimize storage and avoid overwhelming log files.
- Compliance: Adhering to data regulations might require deleting sensitive information that has reached its retention period.
Understanding the Process:
Python does a simple ask-to-the-operating-system file delete action, which effectively asks the operating system to delete that specific file from the storage device and asks for it not to appear in any file contents on the disk, hence freeing the occupied space. Great care and due safeguards should be ensured before taking this action since it cannot be reversed.
Exploring the Methods:
Python offers several methods for deleting files, each catering to different needs and scenarios:
- os.remove: This is the fundamental method for deleting individual files.
- os.unlink: Similar to os.remove, it can be used for various file system objects, including named pipes and symbolic links.
- shutil.rmtree: This method comes into play when you need to delete entire directories and their contents recursively.
- send2trash: This library offers an alternative approach by sending files to the trash bin, allowing potential recovery if needed.
- pathlib.Path.unlink: The pathlib module provides an object-oriented approach to deleting files, offering cross-platform compatibility.
By understanding these methods and their nuances, you can effectively manage file deletion tasks in your Python projects, ensuring efficient data management and maintaining data integrity.
Conquer file deletion in Python with confidence! Master the methods, explore applications, and tackle your assignments with ease. Our experts offer comprehensive Python Assignment Help and Python Homework Help. Get started today and unlock the power of efficient file management!
Methods for Deleting Files:
Python offers various methods for deleting files, catering to different needs and scenarios. Here's an exploration of five prominent methods:
-
os.remove(filename):
The os.remove function, imported from the os module, is the most fundamental method for deleting files. It takes a single argument, which is the path to the file you want to delete. Here's an example:
import os
file_path = "myfile.txt"
try:
os.remove(file_path)
print(f"File '{file_path}' deleted successfully.")
except FileNotFoundError:
print(f"Error: File '{file_path}' not found.")
except PermissionError:
print(f"Error: Insufficient permissions to delete '{file_path}'.")
This code attempts to delete the file "myfile.txt". If successful, it prints a confirmation message. However, it's essential to handle potential exceptions like FileNotFoundError if the file doesn't exist and PermissionError if the user lacks the necessary permissions to delete the file.
-
os.unlink(filename):
It is quite similar to os.remove function since this makes use of deleting files. However, with a slight difference because whereas os.remove is specially reserved to remove files and cannot be used in the place of os.unlink, the reverse can be done. It not only supports removing any kind of filesystem object but also named pipes and symbolic links. An example of using the file method:
import os
file_path = "myfile.txt"
try:
os.unlink(file_path)
print(f"File '{file_path}' deleted successfully.")
except (FileNotFoundError, PermissionError) as e:
print(f"Error: {e}")
The above code is combined to catch either FileNotFoundError or PermissionError for brevity in error handling.
-
shutil.rmtree(path, ignore_errors=False, ignore_dangling_symlinks=False):
When dealing with directories, deleting individual files becomes cumbersome. The shutil.rmtree function, imported from the shutil module, comes to the rescue. It recursively deletes the entire directory and all its contents, including subdirectories and files. Here's an example:
import shutil
directory_path = "my_directory"
try:
shutil.rmtree(directory_path)
print(f"Directory '{directory_path}' and its contents deleted successfully.")
except OSError as e:
print(f"Error: {e}")
This code attempts to delete the directory "my_directory" and its contents. However, be cautious with shutil.rmtree as it's an irreversible operation. It's essential to handle exceptions like OSError in case of permission issues or other errors during the deletion process.
-
send2trash.send2trash(filename):
The send2trash module, available through third-party libraries like Trash-cli, offers an alternative approach to deleting files. Instead of permanent deletion, it sends the file to the system's trash bin, allowing for potential recovery if necessary. Here's an example:
from send2trash import send2trash
file_path = "myfile.txt"
try:
send2trash(file_path)
print(f"File '{file_path}' sent to trash successfully.")
except OSError as e:
print(f"Error: {e}")
This code really uses send2trash to send the file named "myfile.txt" to trash. Take into consideration that everything sent there might be recovered before being permanently deleted from that place.
-
pathlib.Path(path).unlink():
The pathlib module offers an object-oriented way to manage file paths. The Path class allows you to work with file paths in a unified manner, regardless of the operating system. The method unlink of a Path object deletes the file linked at that path. An instance may be found below:
from pathlib import Path
file_path = Path("myfile.txt")
try:
file_path.unlink()
print(f"File '{file_path}' deleted successfully.")
except (FileNotFoundError, PermissionError) as e:
print(f"Error: {e}")
This code demonstrates using pathlib for deleting the file "myfile.txt". Compared to os.remove or os.unlink, this approach offers advantages like automatic path handling and cross-platform compatibility.
Exception Handling:
As discussed earlier, handling potential exceptions when deleting files is crucial to avoid unexpected errors and program crashes. Common exceptions you might encounter include:
- FileNotFoundError: Raised if the specified file doesn't exist.
- PermissionError: Occurs if you lack the necessary permissions to delete the file.
- OSError: A broader category encompassing various file system errors that can occur during deletion.
Here's a more comprehensive example demonstrating exception handling for os.remove:
import os
file_path = "myfile.txt"
try:
os.remove(file_path)
print(f"File '{file_path}' deleted successfully.")
except FileNotFoundError:
print(f"Error: File '{file_path}' not found.")
except PermissionError:
print(f"Error: Insufficient permissions to delete '{file_path}'.")
except OSError as e:
print(f"Error: An unexpected error occurred: {e}")
This example includes a general OSError exception handler to catch any other unforeseen errors that might occur during the deletion process.
Best Practices and Considerations:
Confirmation Prompts:
Before deleting critical files, it's often recommended to seek confirmation from the user to prevent accidental deletion. You can achieve this using the input function to prompt the user for confirmation:
import os
file_path = "important_data.txt"
confirmation = input(f"Are you sure you want to delete '{file_path}'? (y/n): ")
if confirmation.lower() == "y":
try:
os.remove(file_path)
print(f"File '{file_path}' deleted successfully.")
except (FileNotFoundError, PermissionError) as e:
print(f"Error: {e}")
else:
print("File deletion cancelled.")
This code prompts the user for confirmation before proceeding with the deletion.
Applications of Deleting Files in Python
Deleting files in Python is a simple housekeeping task. It finds applications across various domains, each contributing to efficient and effective programming practices. Let's delve into a few key applications:
Data Cleaning and Optimization:
- Temporary Files: Python programs often create temporary files during computation. Deleting these after use frees up valuable storage space and improves overall system performance.
- Log Management: Log files, while crucial for debugging and monitoring, can accumulate over time, consuming significant storage resources. Regularly deleting outdated logs helps maintain a clean and manageable log system.
- Cache Management: Caching mechanisms can become outdated or irrelevant over time. Deleting outdated cache entries ensures your applications utilize the latest and most accurate data.
Data Management and Compliance:
- Archiving and Retention: Deletion of data at the retention period or when it is no more needed complies with the rules and regulations of the data archiving, without the risk of possible breaches.
- Error Handling and Recovery: It shall be applicable for critical work where corrupted or incomplete data needs to be deleted to maintain a clean, propagated-free error system.
- User Management: If an account is deleted, then it can completely remove all user data in order to adhere to data privacy regulations and respecting the privacy of users when he or she requests that it do so.
Software Development and Testing:
- Test Data Cleanup: During automated testing, deleting temporary test data after execution keeps the testing environment clean and avoids confusion with actual application data.
- Version Control: When managing code versions in a version control system, deleting unnecessary or outdated branches helps maintain a clear and organized repository.
- Deployment Management: During application deployment, cleaning up temporary installation files or deployment scripts ensures a clean state for the deployed application.