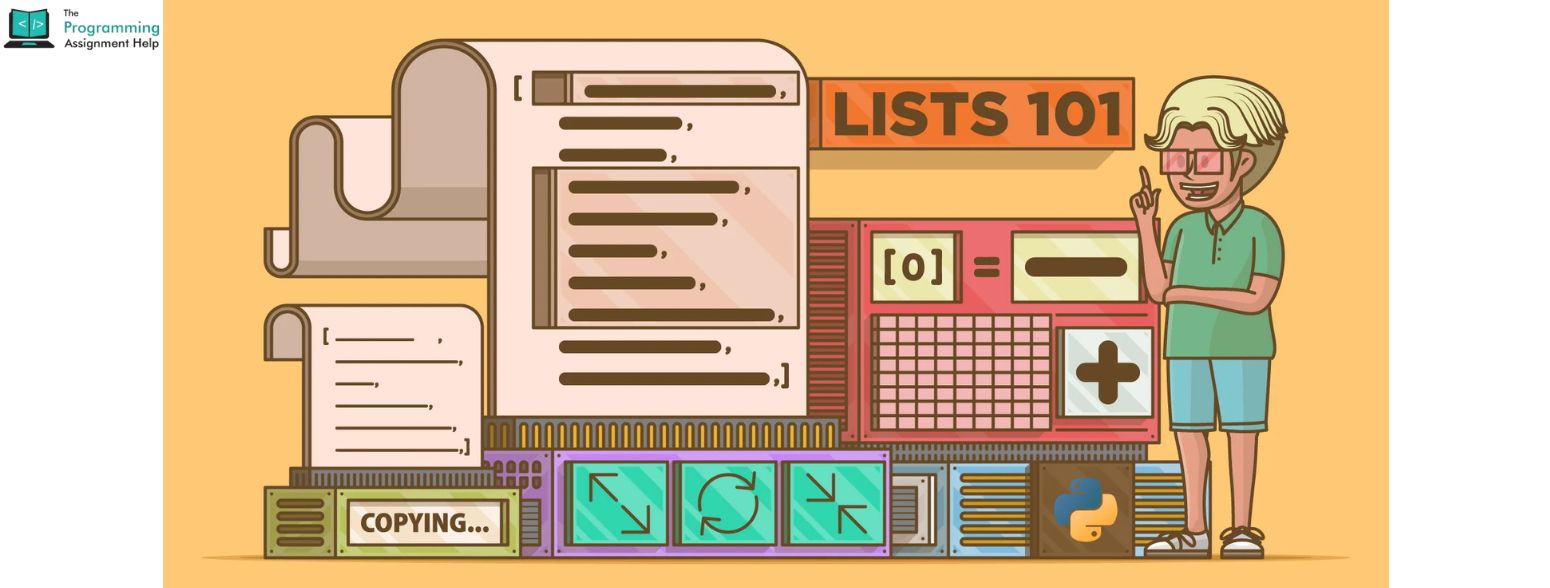
- 29th Oct 2024
- 17:27 pm
- Nia Sharma
Printing lists in Python is a fundamental task that can help visualize data, debug code, or share results. Whether you’re a beginner or an experienced developer, mastering different ways to print lists gives you flexibility and clarity in displaying your output. Here are five practical methods to easily print lists in Python.
1. Using the print() Function Directly
The easiest and most straightforward method to print a list in Python is by using the print()
function. When you pass a list to print()
, it displays the elements in their original format, complete with square brackets and commas. This approach is perfect for basic tasks and offers a quick way to view the contents of a list.
Example:
python
my_list = [1, 2, 3, 4, 5]
print(my_list)
2. Printing Each Item Individually with a for Loop
If you want to print each item on a new line or format them differently, a for loop is a great option. By iterating over each element in the list, you can customize how they are displayed. This method is helpful when you need clean and organized output, especially for long lists or for data that requires readability.
Example:
python
for item in my_list:
print(item)
3. Using join() for Formatted Output
When working with lists of strings, the join() method is particularly useful. It allows you to combine list elements into a single string with a specified separator, such as a comma or space. This method is perfect when you need a clean, inline format without extra punctuation.
Example:
python
string_list = ["apple", "banana", "cherry"]
print(", ".join(string_list))
4. List Comprehension for Compact Printing
List comprehensions are a concise way to perform operations within a list. By using a list comprehension with print(), you can modify and print each element in a single line. This is helpful for quick debugging or when you need to apply transformations before displaying the list.
Example:
python
print([str(item) + "!" for item in my_list])
5. Printing with * Unpacking Operator
The * operator, also known as unpacking, allows you to print a list’s elements without brackets or commas. By adding * before the list, you can display each item separated by a space, making it ideal for creating cleaner output without extra punctuation.
Example:
python
print(*my_list)
Conclusion
These five methods provide flexibility for printing lists in Python, each with its own strengths depending on the situation. Whether you want a simple printout, a structured format, or inline display, mastering these techniques makes data display more efficient and readable. For more help with Python programming, including list handling and data manipulation, The Programming Assignment Help offers expert guidance tailored to your coding needs.