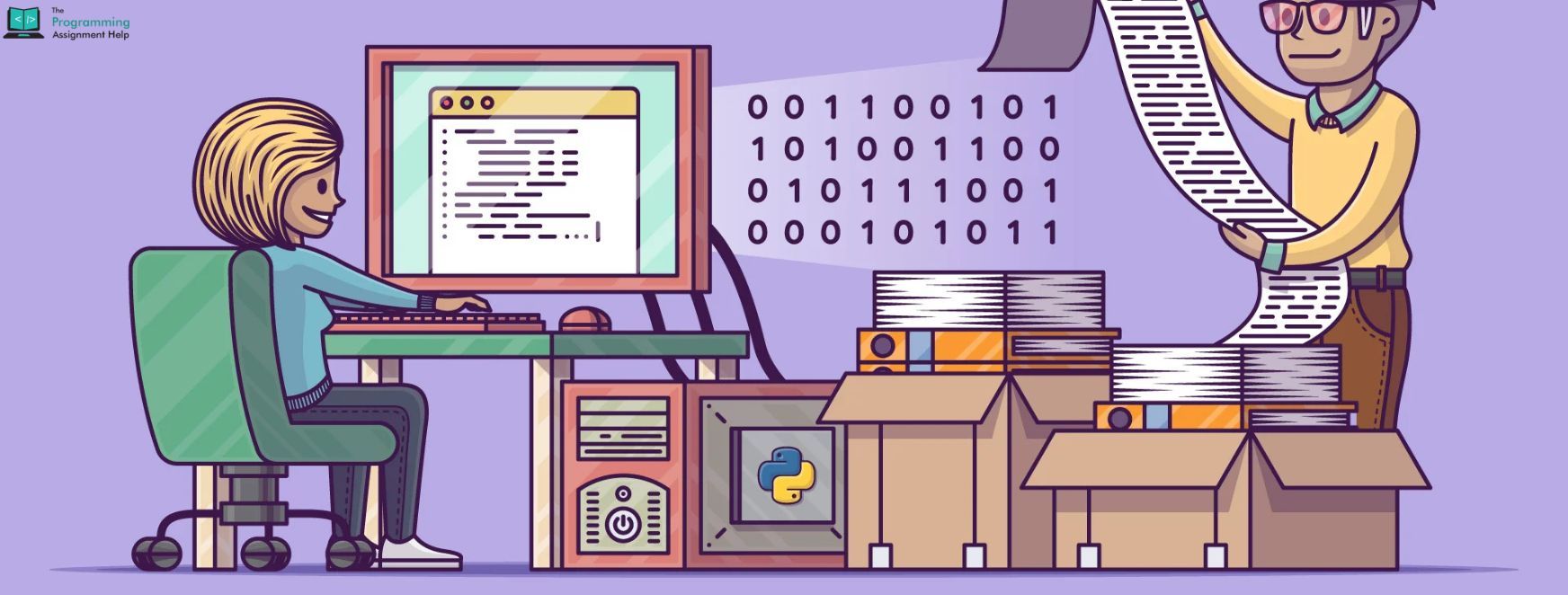
- 15th Oct 2024
- 10:37 am
- Andrew
Mastering file reading in Python is crucial for anyone handling data, text files, or logs. Whether you're working with large datasets or extracting information from a smaller file, efficient file reading can greatly enhance your code's performance and your ability to manage diverse file formats. Python provides multiple methods for reading files line by line, offering flexibility depending on the file size and available memory.
Why Read a File Line by Line?
When dealing with small files, loading the entire file into memory at once might seem straightforward. However, with larger files—sometimes spanning gigabytes—this approach can result in performance issues or even system crashes. Reading files line by line helps prevent these problems by minimizing memory usage, allowing for efficient data processing without overwhelming system resources.
Key Benefits of Reading Files Line by Line:
- Memory Efficiency: By processing only one line at a time, this method prevents loading massive files into memory.
- Streamlined Processing: Line-by-line reading is especially useful for tasks where each line represents a unit of information, like logs or CSV data.
- Flexible Filtering: You can scan the file line by line and easily skip or extract specific lines based on conditions.
Practical Use Cases for Line-by-Line File Reading:
- Log File Analysis: Server logs can be massive, and each line usually represents a separate event or request. Instead of reading the entire log into memory, you can efficiently process one line at a time, extracting important data or filtering out irrelevant entries.
- Large Dataset Processing: In data science and machine learning, datasets can often contain millions of lines. Line-by-line processing ensures you won’t run into memory overload while handling large amounts of data, like CSV files or JSON objects.
- Searching for Keywords: If you're interested in specific lines containing certain keywords or patterns, reading a file line by line allows you to scan and process efficiently without handling the entire file at once.
Efficient File Handling
Python simplifies file handling, and reading files line by line is highly efficient. It uses generators under the hood, meaning the file's content isn’t stored all at once in memory. Each line is loaded, processed, and then discarded, ensuring that memory usage remains minimal. Some key aspects of
Python’s file handling include:
- Context Manager (with statement): Python’s with statement ensures that files are automatically closed after processing, even if an error occurs. This prevents memory leaks and other resource issues.
- Reduced Memory Footprint: By reading files in small chunks (one line at a time), you ensure that your program’s memory consumption stays manageable, even with very large files.
Choosing the Right Method
The method you choose for reading files depends on your needs:
- For large files: Reading line by line is often the best choice, ensuring you don’t run out of memory.
- For smaller files: It may be okay to read the entire file at once or load it into a data structure if memory isn’t a concern.
Conclusion
Reading files line by line in Python is an effective way to process large datasets while keeping memory usage low. Whether you're analyzing server logs, handling massive datasets, or working with text files, mastering this method allows you to manage file handling in a more scalable and efficient manner. By utilizing Python's built-in features and tools, you can ensure that your code runs efficiently, even when dealing with very large files, maintaining both performance and resource management.
If you need assistance with understanding file handling in Python or any other programming tasks, The Programming Assignment Help offers expert guidance. Whether you’re stuck on a coding challenge or need support with your programming projects, their team is ready to provide the help you need to succeed.