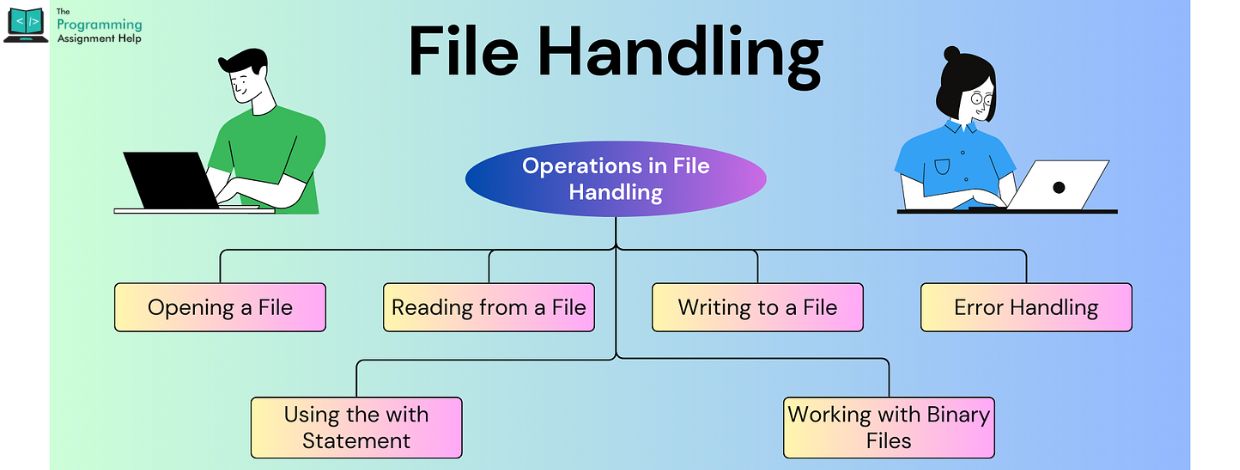
- 24th Sep 2024
- 08:48 am
- Riana Hen
File handling is a vital part of programming that lets us interact with files directly from our code. Whether you’re writing new content, reading data, managing existing files, understanding how to handle files is essential. In Python, file handling is both straightforward and powerful, making it accessible for beginners and useful for seasoned developers. In this blog, we’ll explore how to open, write, and overwrite files in Python—skills that are fundamental in many programming projects. Let’s dive in and discover how you can effectively manage files in your Python applications!
Opening a File in Python
Before working with a file in Python, you need to open it first. Python’s `open()` function makes this super simple. Here’s how the basic syntax looks:
python
file = open("filename.txt", "mode")
- "filename.txt" is the name of the file you want to open.
- "mode" refers to how you want to interact with the file, such as reading, writing, or appending.
Some common modes are:
- "r" – Read mode: Opens the file for reading (default).
- "w" – Write mode: Opens the file for writing (creates a new file if it doesn't exist or truncates the file if it does).
- "a" – Append mode: Opens the file to add new content at the end.
Writing to a File
Once a file is open, you can write content to it. Let's see how this works using "w" mode, which allows you to write new content.
python
file = open("example.txt", "w")
file.write("Hello, world!")
file.close()
In the above code:
The file is opened in write mode. If the file doesn’t exist, Python creates it for you.
The write() method adds text to the file.
Don’t forget to close the file using close() to save the changes.
Overwriting a File
When you open a file in "w" mode, any existing content in the file will be overwritten. This is important to remember, as it means old data will be lost. For example:
python
file = open("example.txt", "w")
file.write("This text will replace the old content.")
file.close()
Now, the file contains only the new text, and anything previously written is gone.
Need Help with Python?
If you need help with Python programming or assignments, The Programming Assignment Help is an excellent resource. They provide expert advice, tutorials, and solutions to help you handle even the most difficult coding problems. File handling may seem tricky at first, but with practice, you'll see how intuitive Python makes the process. Keep coding, and don’t hesitate to reach out for help when you need it!