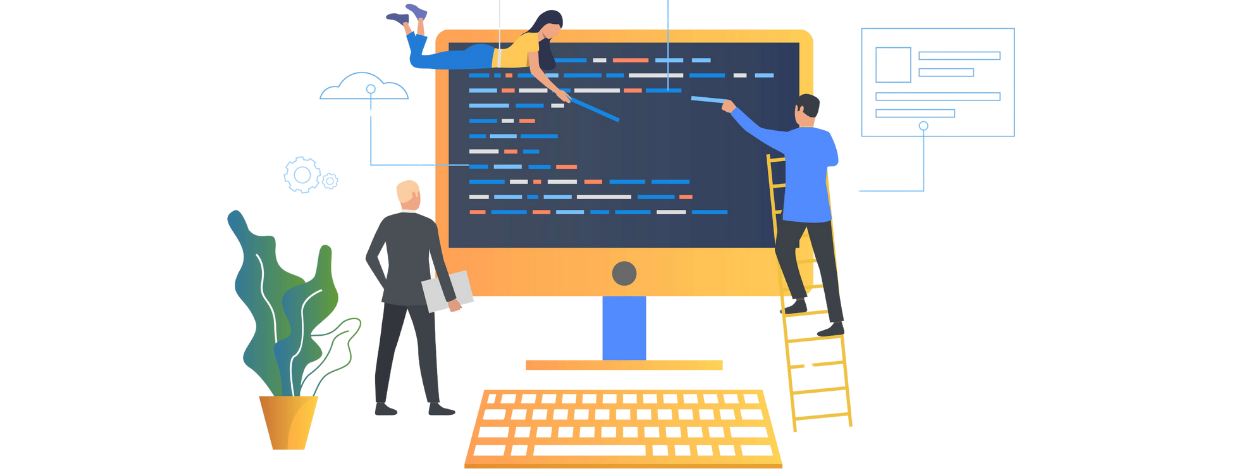
- 14th Feb 2024
- 00:05 am
Pandas is a powerful Python library that helps you organize and analyze data. It uses DataFrames, which are like spreadsheets with rows and columns, to store and manipulate information. But what about when you don't have any data yet? That's where empty DataFrames come in.
Think of an empty DataFrame as a blank canvas, waiting to be filled in by information. The point is, creating and working with those "blank slates" is important for several reasons.
- Starting Point: You can build your analysis step-by-step, adding data as you gather it. This keeps your code organized and helps you track your progress.
- Placeholder: Sometimes, you need a temporary structure to hold information before finalizing it. An empty DataFrame acts as a placeholder, ensuring your code has the right shape even without actual data.
- Conditional Processing: Imagine analyzing different conditions in your data. Empty DataFrames can be used as intermediate results, making your code cleaner and easier to understand.
- Error Handling: Dealing with errors and missing data? Empty DataFrames can help flag or signal issues, making debugging smoother.
So, mastering empty DataFrames unlocks a range of possibilities, both for organizing your data and handling various situations during analysis. Let's delve into the different ways to create and work with them efficiently!
Creating Empty DataFrames
In the world of data analysis, pandas holds a special place, thanks to its versatile DataFrames. But what if you need a DataFrame before the data arrives? Worry not, for pandas offers ways to create empty DataFrames – your blank canvas waiting to be filled. Let's explore:
- The Basic Builder: DataFrame()
Start with the DataFrame() constructor, the foundation of DataFrame creation. Simply call it without any arguments:
empty_df = pd.DataFrame()
print(empty_df)
This gives you an empty DataFrame with no rows or columns.
- Shaping Your Canvas: Dimensions
Define the size of your empty DataFrame using rows and columns:
small_df = pd.DataFrame(columns=['Name', 'Age'], index=[0, 1])
print(small_df)
Here, you create a DataFrame with two columns and two rows, even though they're empty.
- Leveraging Empty Lists and NumPy Arrays:
Use empty lists for columns and NumPy arrays filled with zeros for data:
data = np.zeros((3, 2))
empty_df = pd.DataFrame(data, columns=['City', 'Country'])
print(empty_df)
This creates a DataFrame with three rows, two columns, and all values set to zero.
- Repurposing Existing DataFrames:
Utilize the empty property of an existing DataFrame:
existing_df = pd.DataFrame({'Name': ['Alice', 'Bob']})
empty_df = existing_df.empty
print(empty_df)
This checks if the original DataFrame is empty and returns True if it is.
- Adding Color to Your Canvas: Data Types, Labels, and More:
Specify data types for columns to ensure proper handling:
empty_df = pd.DataFrame(columns=['Date', 'Amount'], dtype={'Date': str, 'Amount': float})
print(empty_df)
Set custom column names and index labels for clarity:
empty_df = pd.DataFrame(index=[1, 2, 3], columns=['Product', 'Sales'])
print(empty_df)
- Beyond the Basics: Advanced Techniques
- Create custom index objects for complex indexing needs.
- Utilize data masks to specify patterns for filling empty values.
Remember, these are just the starting points. As your data analysis skills evolve, you can delve deeper into these advanced techniques.
By mastering these methods, you'll be well-equipped to create empty DataFrames tailored to your specific needs, setting the stage for insightful data analysis in pandas.
Working with Empty DataFrames
While empty DataFrames in pandas might seem like barren landscapes, they offer surprising utility in data analysis. Let's explore how to effectively work with them:
1. Is It Empty? The empty Property
Before venturing into operations, use the empty property to confirm its emptiness:
empty_df = pd.DataFrame()
if empty_df.empty:
print("This DataFrame is indeed empty!")
This simple check prevents unexpected errors down the line.
2. Building From the Void: Adding Data
- Adding Rows: Use the loc or index assignment methods to append rows:
empty_df.loc[0] = {'Name': 'Alice', 'Age': 30}
print(empty_df)
- Adding Columns: Utilize similar methods to insert new columns:
empty_df['City'] = 'New York'
print(empty_df)
Remember, adding data to an empty DataFrame makes it no longer empty.
3. Merging DataFrames: The Power of Concatenation
Concatenate empty DataFrames with others using concat:
df1 = pd.DataFrame({'A': [1, 2]})
df2 = pd.DataFrame({'B': [3, 4]})
combined_df = pd.concat([df1, empty_df, df2], axis=1)
print(combined_df)
This demonstrates how empty DataFrames can act as placeholders while merging.
4. Exploring the Depths: Aggregate Functions
Even on empty DataFrames, functions like count() and size() have meaning:
print(empty_df.count()) # Prints 0, indicating no non-null values
print(empty_df.size) # Prints 0, indicating no values at all
Understanding these behaviors can be helpful for debugging or conditional checks.
5. Taming the Void: Handling Potential Errors
- Prevent Division by Zero: Use conditional statements to avoid errors when dividing:
if not empty_df.empty:
result = empty_df['Value'] / empty_df['Denominator']
print(result)
else:
print("Cannot divide when DataFrame is empty")
- Address fillna() Issues: Employ other methods like mode() or custom logic when filling empty DataFrames.
6. Best Practices: Working Wisely with Empty DataFrames
- Clarity is Key: Use meaningful variable names and comments to explain empty DataFrames' purpose.
- Validation Matters: Check for emptiness before applying operations that might raise errors.
- Embrace Conditionals: Employ conditional statements to handle empty DataFrames gracefully within your code.
Advanced Techniques with Empty DataFrames
Empty DataFrames in pandas might seem simple, but they offer surprising depth for advanced data wrangling. Let's explore some techniques that push the boundaries:
1. Filling the Void: Strategic fillna()
While basic fillna() fills empty values with a single value, consider these:
- Forward Fill: Impute missing values with the previous non-empty value:
df = pd.DataFrame({'A': [1, None, 3]})
df.fillna(method='ffill', inplace=True)
print(df)
- Custom Functions: Use a function to define complex filling logic:
def fill_with_mean(col):
return col.fillna(col.mean())
df['B'] = [4, None, 6]
df.fillna(fill_with_mean, inplace=True)
print(df)
2. Empty Frames, Missing Data:
Filtering with dropna(): Remove rows with any missing values:
df_filtered = df.dropna()
print(df_filtered)
Imputation Strategies: Use empty DataFrames as references for imputation. For example, fill missing values in one DataFrame with the mean of another DataFrame with no missing values.
3. Hierarchical Depths: Empty Levels
Create hierarchical DataFrames with empty levels using MultiIndex.from_tuples():
index = pd.MultiIndex.from_tuples([('A', 1), ('A', 2), ('B', None)],
names=('Level1', 'Level2'))
df_hierarchical = pd.DataFrame(index=index, columns=['Value'])
print(df_hierarchical)
This demonstrates representing missing categories within the hierarchy.
4. Placeholders for the Future:
- Conditional Loading: Load data based on conditions, using an empty DataFrame as a placeholder until the data is available:
if data_available:
df = pd.read_csv('data.csv')
else:
df = pd.DataFrame()
- Deferred Calculations: Assign empty DataFrames to variables for later calculations based on conditions or future data acquisition.
5. Code Examples and Applications:
These are just starting points. Explore advanced techniques like interpolate() for more sophisticated missing data handling, or use empty DataFrames to validate custom data cleaning pipelines. Remember, the key is to understand the context and choose the appropriate technique for your specific data analysis needs.
Applications of Pandas Empty Dataframe
While empty DataFrames in pandas might seem like empty canvases, they're more than just placeholders. Understanding them unlocks valuable applications in data analysis:
- Building With Structure: Imagine starting a new painting. You wouldn't just throw colors around; you'd first sketch the outline. Similarly, in data analysis, you can create an empty DataFrame with defined columns and data types even before the actual data arrives. This ensures your code structure is clear and ready to be filled with information later.
- Missing Data? No Problem: Sometimes, your data comes with gaps. Treating missing values as empty allows you to leverage empty DataFrame techniques. For example, you can filter out entire rows where all values are empty, or aggregate statistics only on non-empty values, providing a clearer picture of your data.
- Conditional Clarity: Imagine writing an "if-else" statement in your code. Wouldn't it be easier to use an empty DataFrame as an intermediate result than handling complex value-based checks? This improves code readability and maintainability, especially when dealing with multiple conditions.
- Error Handling Made Easy: Debugging can be messy. Employing empty DataFrames as flags or placeholders during error handling simplifies the process. For example, if data loading fails, you can create an empty DataFrame to signal the error and prevent subsequent operations, making troubleshooting more efficient.
- Testing and Simulations: Before launching your analysis on real data, you can populate empty DataFrames with simulated values. This is ideal for unit testing your code or creating mock scenarios to validate your analysis approach, ensuring its robustness before dealing with the actual data.
- Beyond the Basics: The applications extend further. You can use empty DataFrames for custom data validation, ensuring specific criteria are met before proceeding. They can also serve as building blocks for reusable data pipelines, streamlining your analysis workflows.