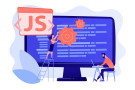
- 29th Nov 2023
- 14:34 pm
In the vast realm of programming, the term "higher-order functions" signifies a profound paradigm shift. These functions, distinguished by their ability to accept or return other functions, unlock new dimensions of expressiveness and modularity. Understanding the essence of higher-order functions is pivotal in unraveling the intricacies of modern, functional programming in JavaScript.
What is a Higher Order Function?
A higher-order function in JavaScript is not just a mere construct; it's a transformative element that bestows unparalleled flexibility to your code. It goes beyond the conventional role of functions by either accepting other functions as arguments or returning them as results. This distinctive capability allows developers to encapsulate and abstract behaviors, fostering modular and reusable code.
By comprehending the nature of higher-order functions, programmers can harness the power of functional programming paradigms, enhancing code clarity and promoting a more declarative style of development.
Characteristics of Higher-Order Functions
Characteristics of higher-order functions lie at the core of their transformative nature. These functions can accept other functions as parameters, enabling the creation of dynamic and adaptable code. Additionally, higher-order functions can return functions as results, allowing developers to encapsulate behaviors within reusable units. This capability empowers programmers to implement powerful abstractions, promoting cleaner and more concise code. The flexibility bestowed by these characteristics is a cornerstone of functional programming, contributing to enhanced code modularity and maintainability.
Functions as Parameters
One of the defining features of higher-order functions is their capability to accept other functions as arguments. This allows developers to pass behavior as a parameter, promoting code reusability and flexibility. Consider a scenario where a sorting algorithm can accept a comparison function, allowing the same sorting logic to be applied to various data types.
function sortBy(arr, compareFunction) {
// Sorting logic using the provided compare function
}
const numbers = [3, 1, 4, 1, 5, 9, 2];
const ascendingOrder = (a, b) => a - b;
const descendingOrder = (a, b) => b - a;
sortBy(numbers, ascendingOrder); // Sort in ascending order
sortBy(numbers, descendingOrder); // Sort in descending order
This flexibility showcases the power of higher-order functions in tailoring behavior based on specific requirements.
Functions Returning Functions
In addition to accepting functions as parameters, higher-order functions can also return functions as their results. This opens the door to dynamic function creation, allowing developers to generate functions tailored to specific use cases.
A classic example is a function factory that produces functions for calculating powered values:
function powerOf(exponent) {
return function(base) {
return Math.pow(base, exponent);
};
}
const square = powerOf(2);
const cube = powerOf(3);
console.log(square(4)); // Output: 16
console.log(cube(2)); // Output: 8
Here, the powerOf function generates new functions based on the provided exponent, showcasing the dynamic nature of higher-order functions.
Closure and Higher Order Functions
The concept of closure, the ability of a function to remember and access variables from its lexical scope even after that scope has finished executing, synergizes seamlessly with higher-order functions. Closures within higher-order functions create a powerful combination, allowing functions to retain and utilize variables from their surrounding environment.
function outerFunction(outerValue) {
return function(innerValue) {
return outerValue + innerValue;
};
}
const addFive = outerFunction(5);
console.log(addFive(3)); // Output: 8
In this example, the inner function retains access to the outerValue variable, even though outerFunction has completed its execution. This encapsulation is a fundamental aspect of higher-order functions.
Built-in Higher-Order Functions in JavaScript
JavaScript incorporates a collection of inherent higher-order functions crucial to functional programming. Functions like 'forEach,' 'map,' 'filter,' and 'reduce' exemplify the principles of higher-order functions, operating on arrays to support versatile programming approaches.
- forEach
The ‘forEach’ function navigates through each element in an array, applying a specified function to each one.
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((number) => {
console.log(number * 2); // Output: 2, 4, 6, 8, 10
});
- map
The ‘map’ function transforms each element of an array based on a provided function, producing a new array.
const doubledNumbers = numbers.map((number) => number * 2);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
- filter
The ‘filter’ function creates a new array containing elements that satisfy a specified condition.
const evenNumbers = numbers.filter((number) => number % 2 === 0);
console.log(evenNumbers); // Output: [2, 4]
- reduce
The ‘reduce’ function accumulates the elements of an array into a single value through a provided function.
const sum = numbers.reduce((accumulator, current) => accumulator + current, 0);
console.log(sum); // Output: 15
These higher-order functions simplify common operations, fostering a declarative and expressive coding style.
Functional Programming Paradigm
The embrace of higher-order functions aligns with the principles of functional programming, emphasizing immutability and the use of pure functions. This paradigm promotes immutable data and pure functions, emphasizing the transformation of data through function composition. Leveraging higher-order functions empowers the development of sophisticated and succinct code, fostering a declarative methodology in tackling problems. This approach taps into the capabilities of higher-order functions to attain abstraction, modularity, and the articulation of intricate logic by composing more straightforward, reusable functions.
Benefits of Higher Order Functions
- Conciseness and Expressiveness: Higher-order functions streamline complex logic, offering a more concise and expressive coding style.
- Code Reusability: They facilitate the reuse of functions, reducing redundancy and promoting a modular coding structure.
- Maintainability: Adopting the functional approach elevates code maintainability, as it compartmentalizes logic into smaller, more manageable components.
- Readability: Higher-order functions play a pivotal role in enhancing code readability, facilitating a better understanding for developers, and promoting collaborative efforts on projects.
- Functional Programming Principles: Aligning with functional programming principles, these functions empower developers to employ powerful tools like map, filter, and reduce.
- Elegant Problem Solving: Higher-order functions enable elegant solutions to intricate problems, contributing to the creation of more sophisticated and efficient code.
- Scalability: By promoting a modular and reusable design, higher-order functions support scalability, allowing for smoother project growth.
- Adaptability: The functional nature of higher-order functions makes code adaptable, facilitating easier modifications as project requirements evolve.
Real-World Applications
The real-world applications of higher-order functions span various domains.
Front-End Development:
- Event Handling: Higher-order functions are extensively used to manage events in user interfaces, enabling dynamic and responsive interactions.
- Asynchronous Operations: They play a crucial role in handling asynchronous tasks, such as fetching data from APIs or processing user input without blocking the main thread.
Back-End Development:
- Data Transformation: Higher-order functions are employed to process and transform data efficiently, promoting modularity and maintainability.
- Middleware Functions: In server-side development, they are utilized as middleware to enhance the functionality of server routes and endpoints.
Functional Programming:
- Code Abstraction: Higher-order functions contribute to code abstraction, allowing developers to write concise and expressive code by encapsulating common patterns.
- Reusability: The ability to pass functions as parameters and return them as results enhances code reusability, reducing redundancy.
Concurrency and Parallelism:
- Concurrency Control: Higher-order functions are leveraged in managing concurrent tasks, ensuring effective control over parallel processes.
- Parallel Computing: They play a role in parallel computing, where tasks are divided and executed simultaneously for improved performance.