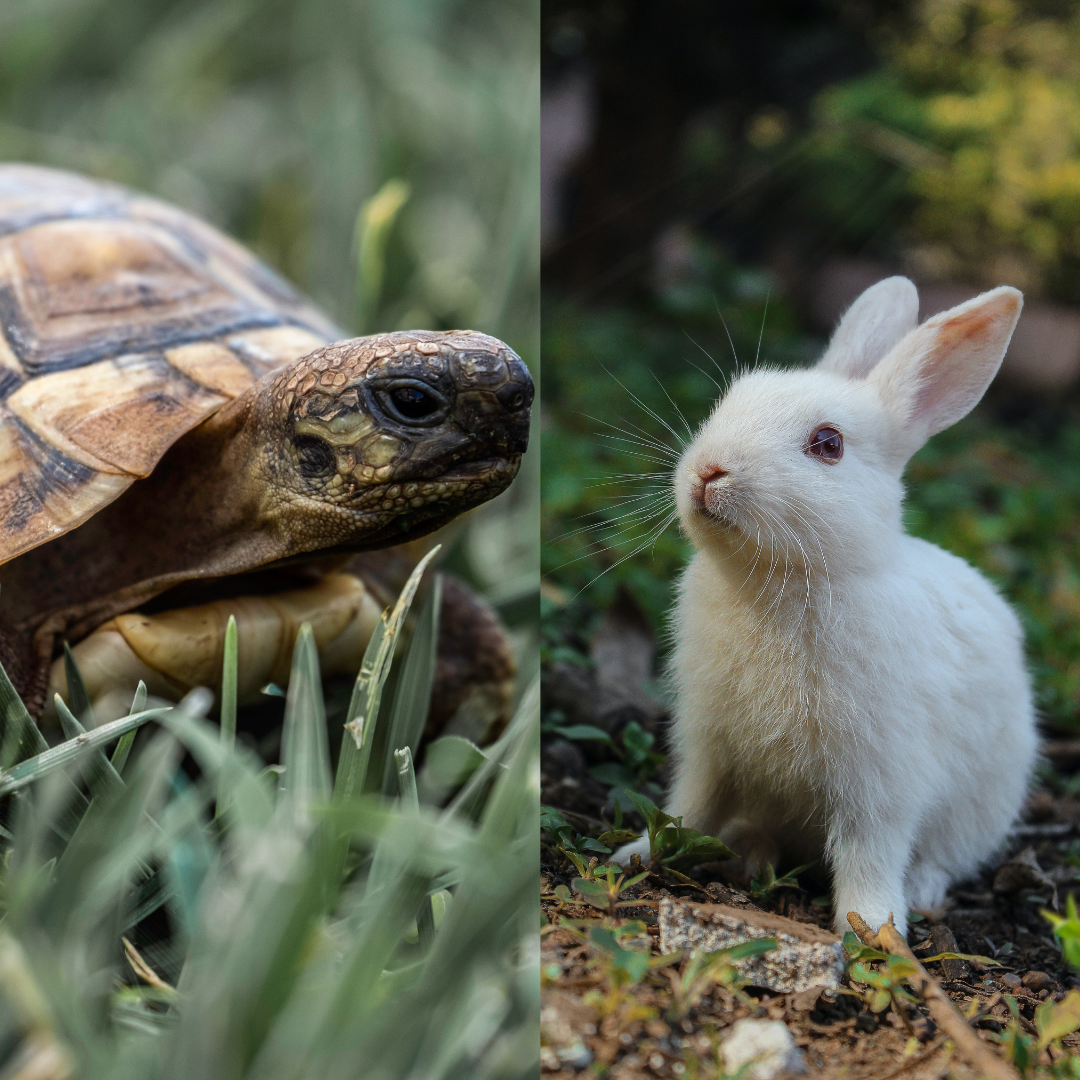
- 6th Apr 2023
- 07:19 am
- Admin
Python Homework Help
Python Homework Question
Python Logical Puzzles, Games, and Algorithms: Tortoise vs Hare
Python Homework Solution
Here's a Python program that simulates the classic race between the tortoise and the hare using random numbers:
import random
# Set up starting positions and finish line
tortoise_pos = 0
hare_pos = 0
finish_line = 70
# Define the moves for the tortoise and hare
def tortoise_move(pos):
"""Calculate the tortoise's next move."""
if random.randint(1, 10) <= 5:
pos += 3
else:
pos -= 6
if pos < 0:
pos = 0
return pos
def hare_move(pos):
"""Calculate the hare's next move."""
move = random.randint(1, 10)
if move <= 2:
pass # No move
elif move <= 4:
pos += 9
elif move <= 5:
pos -= 12
if pos < 0:
pos = 0
elif move <= 8:
pos += 1
else:
pos -= 2
if pos < 0:
pos = 0
return pos
# Loop until one of the animals reaches the finish line
while True:
# Move the animals
tortoise_pos = tortoise_move(tortoise_pos)
hare_pos = hare_move(hare_pos)
# Check if the race is over
if tortoise_pos >= finish_line or hare_pos >= finish_line:
break
# Print the current positions of the animals
print("Tortoise: " + "-" * tortoise_pos + "T")
print("Hare: " + "-" * hare_pos + "H")
# Determine the winner and print the result
if tortoise_pos > hare_pos:
print("TORTOISE WINS!!!")
elif hare_pos > tortoise_pos:
print("Hare wins. Yuch.")
else:
print("It's a tie.")
The program sets up the starting positions of the tortoise and hare, as well as the finish line. It then defines functions to calculate the next move for each animal. The function for the tortoise moves it forward 3 or backward 6, while the function for the hare has a more complicated set of moves based on random numbers.
The program then enters a loop that repeatedly moves the animals until one of them crosses the finish line. After each move, it prints the current positions of the animals. When the race is over, the program determines the winner and prints the result.