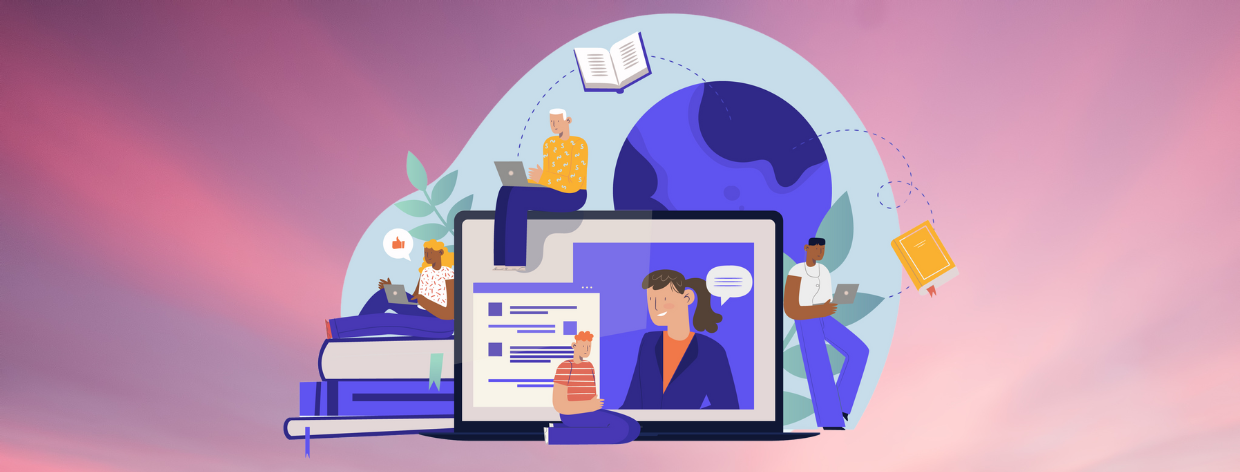
- 15th Mar 2024
- 16:15 pm
- Admin
Modern applications rely heavily on databases for persistent data storage and retrieval. These digital warehouses hold the lifeblood of your application, from user information to complex transactional records. But how do you, as a Java developer, interact with these vital data sources? Enter JDBC, the Java Database Connectivity API.
JDBC (Java Database Connectivity) simplifies connecting Java programs to databases like MySQL or Oracle. It acts as a translator, letting you use the same code regardless of the specific database. This makes development easier and code more portable. This bridge offers several key benefits:
- Portability: JDBC lets you write database code once and use it with various databases with minor changes. It handles the specifics, so you don't need to learn each database's language.
- Flexibility: JDBC lets you control your database: ask questions (queries), update info, and add/remove data. This flexibility makes JDBC a powerful tool for Java database applications.
- Standardization: Using JDBC keeps your database code clean and easy to manage. It works with different Java environments, so you don't need to rewrite everything if you switch systems.
With JDBC at your disposal, you unlock the potential to build robust and scalable database-driven applications in Java. Conquer database interactions, Master JDBC fundamentals and unleash the power of databases in your Java applications with our expert Java Assignment Help and Java Homework Help and conquer Java coding challenges!
JDBC Architecture and Components
JDBC leverages a well-defined layered architecture that promotes sturdy separation of concerns between the Java applications logic and the underlying database machine. Here's a breakdown of the important things additives:
JDBC Driver: This acts as the bridge between your Java code and the database. It translates JDBC API calls into specific protocols understood by the underlying database system. There are different types of drivers, each with its own implementation approach:
- Type 1 Driver (JDBC-ODBC Bridge): Early JDBC drivers sometimes used a separate ODBC layer to connect to databases. This approach is less common today.
- Type 2 Driver (Native Driver): This driver comes directly from the database vendor and uses a platform-specific native library to interact with the database. (Limited portability)
- Type 4 Driver (Pure Java Driver): This driver is written entirely in Java and doesn't require any platform-specific libraries. It's the most common and portable type of driver.
DriverManager: JDBC uses a "driver manager" to find the correct helper program (driver) for the specific database you want to connect to. Your program tells the manager the database address and login details, and the manager finds the matching driver to make the connection.
Connection: This object represents a session with a specific database. It allows you to create statements, execute queries, and manipulate data within that database.
Statement: This object is used to send SQL statements to the database. There are three main types of statements:
- Statement: Provides a basic way to execute simple SQL queries.
- PreparedStatement: Offers a more secure approach for executing dynamic queries with parameter binding, preventing SQL injection vulnerabilities.
- CallableStatement: Used for invoking stored procedures within the database.
- ResultSet: The ResultSet holds your query's data like a table. Loop through it, row by row, to access values (getTextString, getInt).
Establishing a JDBC Connection
This guide will show you how to connect your Java programs to databases using JDBC (Java Database Connectivity). JDBC acts like a translator, allowing your Java code to talk to various databases.
Load the JDBC Driver:
Traditionally, JDBC connections were established by loading the JDBC driver class using Class.forName("driverClassName"). This approach explicitly registers the driver with the DriverManager.
(Alternative for JDBC 4+): With JDBC 4 and above, driver registration often happens automatically when you include the JDBC driver library in your classpath. However, it's still recommended to explicitly load the driver class for better control and to avoid potential issues in certain environments.
1. Establish the Connection:
After loading the correct JDBC driver, use the DriverManager.getConnection(url, username, password) method to establish a connection with the target database. This method takes three key pieces of information:
- url: This is a string specifying the connection details in a specific format. It typically includes the database vendor, hostname, port number, and database name (e.g., jdbc:mysql://localhost:3306/mydatabase).
- username: The username for accessing the database.
- password: The corresponding password for the provided username.
Example:
String url = "jdbc:mysql://localhost:3306/mydatabase";
String username = "root";
String password = "password";
Connection connection = DriverManager.getConnection(url, username, password);
2. Verify the Connection (Optional):
You can check if the connection was successful using connection.isValid(timeout) (available since JDBC 4.1). This method returns true if the connection is valid within the specified timeout period (in seconds).
Optimizing Connections with Connection Pooling:
While establishing connections is straightforward, repeatedly creating and closing connections in your application can be inefficient. This is where connection pooling comes in.
- Connection Pooling: JDBC connection pools manage pre-made database connections. Your program can borrow connections instead of creating new ones each time. This makes things faster and saves resources.
Benefits of Connection Pooling:
- Improved Performance: Reduces overhead associated with creating and closing connections frequently.
- Scalability: Allows your application to handle more concurrent database requests efficiently.
- Resource Management: Simplifies connection management and reduces the risk of connection leaks.
Java connection pools like DBCP or HikariCP recycle database connections. This cuts down on creating new connections every time, making things faster for frequent database calls.
Working with Statements and ResultSets
Having established a connection, it's time to interact with your database! JDBC provides different types of statements to execute queries and manipulate data. Let's explore these options and how to retrieve results.
Statement Types:
- Statement: This is the most basic type of statement. It allows you to execute simple SQL queries as strings.
- PreparedStatement: PreparedStatements protect your Java database code from hacks (SQL injection) by separating data from queries. They use placeholders (?) for values, which are plugged in later. This is especially important for user input to prevent security issues.
- CallableStatement: CallableStatements lets your Java program call pre-written routines (stored procedures) on the database. This is handy for complex tasks or re-usable code within the database.
Example: Statement for Simple Queries
Here's how to create and execute a Statement object for a simple query that retrieves all users from a table:
Statement statement = connection.createStatement();
String query = "SELECT * FROM users";
ResultSet resultSet = statement.executeQuery(query);
// Process the results (explained later)
statement.close();
PreparedStatement for Dynamic Queries:
PreparedStatements offer a safer and more efficient approach for dynamic queries. Here's an example with parameter binding:
String query = "SELECT * FROM users WHERE username = ?";
PreparedStatement preparedStatement = connection.prepareStatement(query);
preparedStatement.setString(1, "john_doe"); // Set the value for the first placeholder (?)
ResultSet resultSet = preparedStatement.executeQuery();
// Process the results (explained later)
preparedStatement.close();
Understanding ResultSets:
The ResultSet acts like your search results, with rows as records and columns as database fields. You can loop through them and access data using methods like getString() or getInt() depending on the data type. This lets you efficiently process results in your Java program.
- getString(columnIndex): Retrieves a String value from the column at the specified index.
- getInt(columnIndex): Retrieves an integer value from the column at the specified index.
- getDouble(columnIndex): Retrieves a double-precision floating-point value from the column at the specified index.
Similar getter methods exist for other data types commonly supported by relational databases.
Iterating Through the ResultSet:
while (resultSet.next()) {
int id = resultSet.getInt(1); // Assuming ID is the first column
String username = resultSet.getString(2); // Assuming username is the second column
// Process the retrieved data (e.g., print, store in a list)
}
resultSet.close();
Exception Handling in JDBC
JDBC applications interact with external resources like database connections and network communication. This introduces the possibility of errors and unexpected situations. Proper exception handling is crucial for ensuring your application reacts gracefully to issues and avoids crashing unexpectedly.
JDBC throws exceptions to signal errors encountered during database operations. The most common exception you'll encounter is SQLException and its subclasses, which provide specific details about the encountered problem. These subclasses include:
- SQLNonTransientException: It means your database query has a major issue (like typos or bad data). You can't fix it by retrying - gotta fix the code!
- SQLTransientException: Indicates your database connection had a temporary glitch (like network issues). Retrying the operation might fix it, so don't panic!
Enhancing Exception Handling with Try-with-Resources:
Java's try-with-resources block makes handling database connections and resources in JDBC a breeze. It automatically closes things like connections, statements, and results when the block finishes, even if errors occur. This keeps your code clean and prevents memory leaks.
Here's an example showing how try-with-resources simplifies exception handling:
try (Connection connection = DriverManager.getConnection(url, username, password)) {
Statement statement = connection.createStatement();
String query = "SELECT * FROM users";
ResultSet resultSet = statement.executeQuery(query);
// Process results (omitted)
} catch (SQLException e) {
// Handle the exception appropriately (e.g., log the error, inform the user)
}
Exploring Advanced JDBC Topics
We've explored connecting to databases, running queries, and working with results. But JDBC has even more tricks up its sleeve! Let's take a peek at some advanced features for more complex database interactions:
- Connection Pooling Frameworks: We mentioned connection pools before. They pre-create database connections for your program to borrow, making things faster and saving resources. Popular choices include DBCP and HikariCP.
- Transactions and Transaction Management: JDBC lets you group database actions together (transactions). You can commit all changes if everything works, or undo everything (rollback) if something fails. This keeps your data consistent.
- Database Metadata: JDBC provides access to database metadata, which is information about the database schema itself. You can use this information to dynamically construct queries or discover available data types and table structures within the database.
- Batch Updates: For efficient data manipulation involving large datasets, JDBC allows batch updates. JDBC lets you group several update, insert, or delete commands together (batching). This speeds things up compared to doing them one by one, making your code more efficient.
Conclusion:
This JDBC guide has given you the basics to connect to databases, run queries, and manage data in your Java programs. You're now equipped to start working with real databases in your applications! Remember, JDBC unlocks a vast potential for building sophisticated database-driven systems. Explore advanced topics like connection pooling, transactions, and batch updates to further refine your database interactions.
For further learning, consider resources like the official JDBC documentation, tutorials on JDBC frameworks, and online courses dedicated to database programming in Java. With continued exploration, you'll be well on your way to unlocking the full potential of databases in your Java projects!
About the Author
Mr. Daniel Lee
Qualification: Master's degree in Software Engineering
Expertise: Java web development maestro, crafting dynamic and interactive web applications.
Research Focus: Mr. Lee explores the intersection of Java and modern web frameworks like Spring MVC and JavaFX. He investigates techniques for building Single-Page Applications (SPAs) and real-time web experiences with Java.
Experience: Leads development teams in creating feature-rich web applications, utilizing Java frameworks to deliver efficient and scalable web solutions.
Mr. Lee's mastery of Java web development empowers him to design and build engaging and interactive web applications at the forefront of modern web technologies.