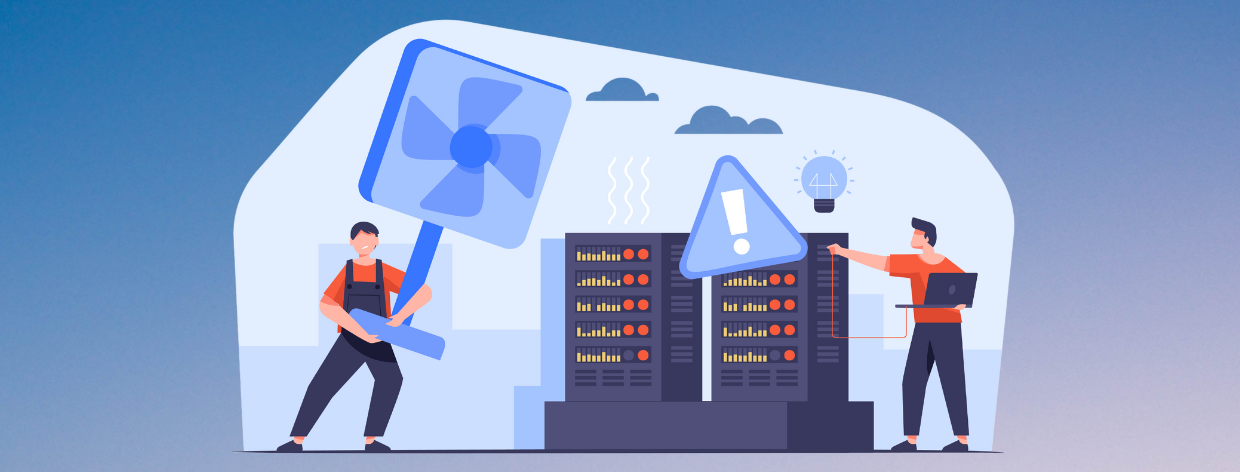
- 13th Mar 2024
- 22:52 pm
- Admin
In the world of Java programming, unexpected events can disrupt the smooth execution of your code. These unforeseen circumstances, known as exceptions, can arise due to various reasons: user input errors, file access issues, network problems, or internal logic flaws. Left unhandled, exceptions can lead to program crashes, leaving users confused and frustrated.
Errors happen. Java's try-catch-finally structure helps manage them gracefully. The try block holds error-prone code, while catch blocks define actions for specific exceptions. Finally, the finally block executes code regardless of errors, often for cleanup tasks. This approach prevents crashes, maintains program flow, and protects data integrity, leading to more reliable and user-friendly software.
The try block serves as the foundation, where you place the code susceptible to exceptions. If an exception occurs during execution within the try block, control is transferred to the catch block(s) designed to handle that specific type of exception. Finally, the finally block, if present, guarantees execution regardless of how the try block exits (normally or due to an exception). Let's delve deeper into each component to understand their roles and functionalities in detail.
Struggling with Java exception handling in your assignments or homework? Master the try-catch-finally construct and conquer those errors! Dive into our comprehensive Java Assignment Help and Java Homework Help service and conquer your coding challenges!
The Try Block
The try block forms the core of Java's exception-handling mechanism. It serves as a designated zone where you place code segments that have the potential to throw exceptions during execution. These could be operations involving:
- File access
- User input
- Network communication
- Any other scenario prone to unforeseen errors
Execution Flow within the Try Block:
- Code within the try block executes normally as long as no exceptions are encountered.
- The program proceeds sequentially, line by line, until it reaches the end of the try block or an exception disrupts the normal flow.
Importance of Encapsulating Exception-Prone Code:
Placing potentially error-prone code within the try block allows the program to anticipate and manage exceptions gracefully. By isolating these sections, you create a controlled environment with several benefits:
- Anticipates exceptions: The try block flags potential trouble spots in your code.
- Controlled response: You can define how the program reacts to exceptions within the try block.
- Prevents program crashes: By handling exceptions, you avoid abrupt program termination due to errors.
For instance, imagine a code snippet reading data from a file. This operation could potentially throw an IOException if the file doesn't exist or is inaccessible:
try {
// Code that reads data from a file (potentially throws IOException)
} catch (IOException e) {
// Code to handle the IOException (e.g., display error message)
}
By using exception handling, you make your program more resilient. It can handle unexpected situations gracefully, preventing crashes and providing a better experience for the user. This allows your program to continue running smoothly even when file reading encounters problems.
Limitations of the Try Block:
It's important to note that the try block itself doesn't handle exceptions. Its primary role is to identify potentially risky code segments. However, it has one significant limitation: unchecked exceptions. These runtime errors, like NullPointerException or ArrayIndexOutOfBoundsException, bypass the try-catch mechanism entirely and can still lead to program termination if not handled elsewhere in your code.
The Catch Block
The catch block acts as the hero in Java's exception-handling drama. It's where you define how to handle exceptions that erupt within the corresponding try block. Here's how it all plays out:
The Role of the Catch Block:
Imagine an exception erupts during code execution within the try block. The program flow redirects to the first catch block that can handle the specific type of exception thrown. This catch block then contains the code responsible for dealing with the exception in a meaningful way.
Catching and Handling Exceptions:
- Catching: A catch block is defined using the catch keyword followed by parentheses containing the exception type it can handle.
- Handling: The code within the catch block addresses the exception. This might involve displaying an error message, logging the error for debugging purposes, attempting to recover from the error, or gracefully terminating the program.
Multiple Catch Blocks and Exception Hierarchies:
You can define multiple catch blocks after a single try block. The order of these catch blocks is crucial. Java scans them from top to bottom, looking for the first catch block that matches the type of exception thrown. Here are two common approaches:
- Specific Exception Types: Define a separate catch block for each specific exception type you anticipate. This provides granular control over how different exceptions are handled.
try {
// Code that might throw exceptions
} catch (IOException e) {
// Handle IOException
} catch (NumberFormatException e) {
// Handle NumberFormatException
}
- Parent-Child Exception Hierarchies: Leverage Java's exception hierarchy. You can use a catch block that handles a parent exception type, effectively catching all its child exceptions as well.
try {
// Code that might throw exceptions
} catch (Exception e) {
// Handle all Exception types (including subclasses like IOException)
}
Exception Chaining with Throwable:
Java exceptions help handle errors. While the Throwable class lets you catch any type of exception, it's generally not recommended. This is because catching everything makes your code harder to understand. It's better to catch specific exceptions so you can provide targeted solutions and keep your code clean and clear.
Limitations of Catch Blocks:
While catch blocks are powerful, they have limitations:
- Multiple Exceptions: Your code might face different errors. Use separate catch blocks for each specific exception you expect. But be mindful - too many can clutter things up! Aim for catching specific errors for cleaner code. Remember, the order of catch blocks matters - list the most specific exceptions first!
- Rethrowing Exceptions: In programming, rethrowing an exception means catching it and then throwing it again. This lets a higher level in your program handle the error. Use it cautiously though, as it can make debugging tougher.
The Finally Block
The finally block serves as the dependable janitor in Java's exception-handling world. Regardless of how the try block exits (normally or due to an exception), the finally block is always guaranteed to execute. This ensures essential cleanup tasks are performed, even in the midst of errors.
Guaranteed Execution:
Imagine an exception erupts within the try block, and the program jumps to the corresponding catch block for handling. But what happens to resources opened within the try block, like files, network connections, or database handles? The finally block guarantees that the code within it will execute, regardless of how the try block exits (normally, with an exception, or even due to a return statement). This ensures proper cleanup and resource management, preventing potential leaks or inconsistencies.
Common Use Cases for the Finally Block:
The finally block proves invaluable in various scenarios:
- Resource Cleanup: Closing files, network connections, database connections, or releasing other system resources acquired within the try block. This ensures they are properly released, even if an exception occurs.
try (BufferedReader reader = new BufferedReader(new FileReader("data.txt"))) {
// Read data from the file
} finally {
// Guaranteed to close the reader, even if an exception occurs
if (reader != null) {
reader.close();
}
}
- Releasing Locks: Releasing locks acquired within the try block to prevent deadlocks. Even if an exception occurs, the lock will be released, allowing other threads to proceed.
- Executing Essential Code: Running critical code that must execute regardless of exceptions, like logging error messages or updating internal state.
Limitations of the Finally Block:
The finally block is great for cleanup, but does not change program flow. Don't use it to return or throw exceptions after errors. Its job is to ensure essential tasks like closing files or freeing memory happen regardless of errors. This keeps your code clean and avoids resource leaks.
By understanding the guaranteed execution and use cases of the finally block, you can ensure your Java code handles exceptions gracefully while maintaining clean resource management practices. The next section will explore best practices for crafting robust exception-handling mechanisms using the try-catch-finally construct.
Mastering Exception Handling:
Crafting robust exception handling goes beyond simply catching exceptions. Here are key practices to elevate your Java code:
- Embrace Specific Exception Handling: While a generic catch (Exception e) block might seem convenient, it's often better to define separate catch blocks for specific exception types. This improves code readability and allows for targeted handling of different exceptions.
- Declare Exceptions in Method Signatures: The throws keyword within a method signature allows you to declare the exceptions a method might throw. This informs callers about potential exceptions and encourages them to handle them appropriately.
- Understand Checked vs. Unchecked Exceptions: Java differentiates between checked and unchecked exceptions. Checked exceptions (like IOException) must be declared in method signatures, forcing callers to consider them. Unchecked exceptions (like NullPointerException) bypass the try-catch mechanism and require more proactive handling throughout your code.
- Leverage Automatic Resource Management (ARM): Java offers resources with built-in automatic closing (like try-with-resources). This simplifies resource management by ensuring proper cleanup, even in the face of exceptions. By utilizing ARM resources, you can often avoid explicit finally blocks for basic closing operations.
Advanced Exception Handling Techniques
The try-catch-finally construct offers a powerful foundation, but Java provides additional tools for complex scenarios:
- Nested Try-Catch Blocks: You can nest try-catch blocks within each other. This allows for more granular exception handling within specific code sections. An inner try block can catch exceptions specific to its code, while an outer try block might handle broader exceptions.
- Try-with-Resources for Automatic Cleanup: Introduced in Java 7, the try-with-resources statement simplifies resource management. It automatically closes resources upon exiting the try block (normally or due to an exception). This eliminates the need for explicit finally blocks for basic closing operations of resources like files, network connections, or database handles.
- Exception Handling in Multithreading: When working with multiple threads, exceptions can become trickier. Java provides mechanisms like synchronized blocks and thread-safe collections to ensure data consistency even when exceptions occur. Additionally, you can propagate exceptions thrown by one thread to the main thread for handling.
Conclusion:
The try-catch-finally construct forms the cornerstone of exception handling in Java. The try block identifies code prone to exceptions, while catch blocks define how to handle specific exceptions that arise. The finally block ensures essential cleanup tasks happen regardless of how the try block exits.
Effective exception handling is key to robust Java code. Catch specific exceptions, leverage throws, and try-with-resources for cleaner code. This prevents crashes, improves error messages, and boosts overall code quality. Remember: handled exceptions are gracefully overcome!
About the Author
Ms. Anya Petrova
Qualification: Master's degree in Software Engineering
Expertise: Java security champion, building secure and robust back-end systems.
Research Focus: Investigate and implement cutting-edge Java security frameworks like Spring Security and OWASP libraries to mitigate vulnerabilities and prevent cyberattacks.
Experience: Develops secure back-end systems for mission-critical applications, employing threat modeling and secure coding practices.
Ms. Petrova's dedication to Java security ensures she crafts reliable and well-protected applications, safeguarding sensitive data and functionality.