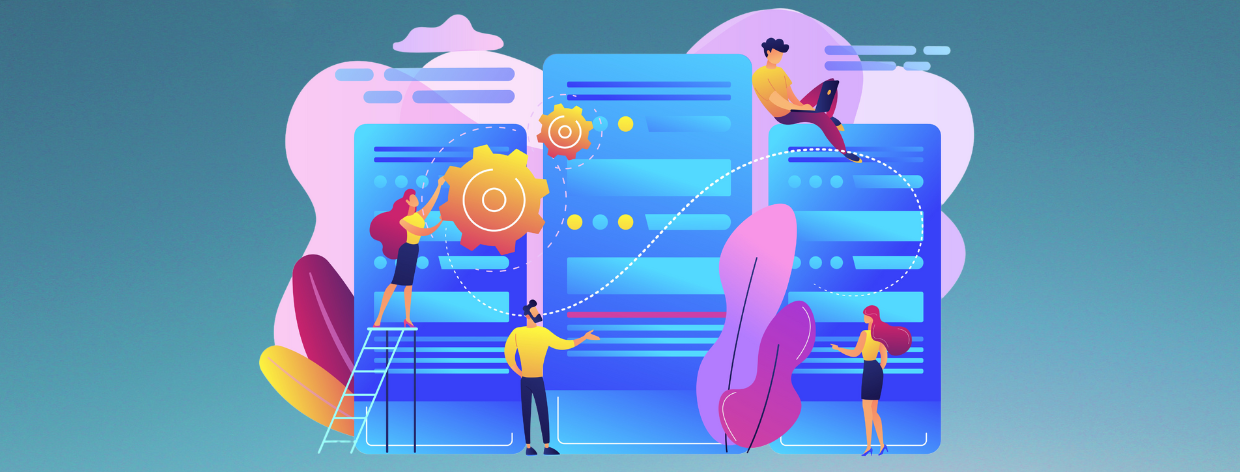
- 14th Mar 2024
- 23:17 pm
- Adan Salman
The digital world thrives on the ability of computers to communicate and share information. This communication happens over computer networks, which are interconnected systems that allow data exchange. But how do individual programs on these machines establish a connection and exchange data? Here's where sockets come into play.
Sockets: The Building Blocks of Network Communication
Sockets can be defined as inter-process communication (IPC) endpoints that provide a mechanism for data exchange between programs on a network. Sockets are the core of network communication in Java. They act like special ports that programs use to exchange data (chat) across a network. These endpoints allow programs to send and receive information, forming the foundation for many network applications. They're like channels that let programs send and receive streams of data (like text or images) back and forth. Sockets are the building blocks for network programming. They offer more control over how data is sent compared to some simpler tools, allowing you to customize how your programs talk to each other on the network.
Benefits of Socket Programming:
- Client-Server Model: Sockets power client-server networks. A server listens for connections on a port, like a central chatroom. Clients connect and chat back and forth (data exchange). This model is great for many network applications.
- Flexibility and Control: Socket programming offers a lower-level approach compared to higher-level network APIs. This grants you greater control over connection establishment, data transfer, and error handling, allowing you to tailor network communication to your specific application requirements.
- Foundation for Diverse Applications: From building simple chat applications to complex file transfer protocols, sockets provide the essential building blocks for a wide range of network-centric applications in Java.
By delving into socket programming, you unlock the potential to create powerful and versatile network applications in the Java ecosystem. Build your network application expertise and Conquer network communication challenges to unleash the power of sockets with expert Java Assignment Help and Java Homework Help!
Demystifying Sockets: The Essentials of Network Programming in Java
Sockets are like special connection points in Java that programs use to talk to each other over a network. They allow programs to send and receive information (like text or images) back and forth, just like chatting with a friend online. This is essential for building many internet applications.
The Client-Server Model: A Foundation for Network Communication
Java socket programming thrives on the client-server network model. This model involves two distinct roles:
- Server: The server program acts like a central chatroom in Java. It waits on a specific channel (like a port number on a walkie-talkie) for someone (a client program) to connect. Once connected, the server can chat back and forth (exchange data) with that client. This allows programs to send and receive information over a network.
- Client: The client program acts like someone calling the server. It reaches out to the server on a specific channel (port) to establish a connection. Once connected, the client can send information to the server and get replies back. This lets programs exchange data over a network.
Sockets serve as the underlying mechanism for facilitating this communication flow within the client-server model.
Java's java.net Package and Key Classes
The java.net package in Java provides essential classes for socket programming:
- Socket: This class represents a connection between a client and a server. It offers methods for sending and receiving data streams over the established connection.
- ServerSocket: The server uses a special class (ServerSocket) to open a waiting area on a chosen channel (port). It provides methods for accepting incoming connection requests from clients.
- InetAddress: This is like an address book for the network. It helps the client program find the server's location (IP address) so it knows where to connect. This way, the client knows the exact "house" (server) to visit on the Internet.
- DatagramSocket: Datagram Sockets use UDP, a speedy way to send data packets. Unlike some delivery services, UDP doesn't check if each packet arrives. It just fires them off directly. This makes it faster, but there's a chance packets might get lost. It's perfect for things like live streams or online games where a little missing data isn't a big deal.
Establishing a TCP Connection: A Step-by-Step Guide
We'll explore the essential steps for setting up a TCP connection between a client and server program using sockets in Java. Grasping this process is foundational for developing network-powered applications.
- Server-Side Setup: The server program creates a special waiting area (ServerSocket) on a chosen channel (port).
- Client-Side Initiation: The client program dials the server's address and port number, requesting a connection.
- Server Accepts Connection: The server's waiting area listens for calls (connection requests). When a client calls (requests a connection), the server accepts the call and creates a dedicated line (Socket) for that client.
- Data Exchange: Now both the server and client can send and receive messages (data) through their dedicated lines (Sockets). They use methods like "get input" and "get output" to do this.
Common Socket Options for Fine-Tuning Communication
Java sockets allow customization through various options. Here are a few examples:
- SO_TIMEOUT: This option lets you set a timer for waiting on data. If no data arrives within the set time, the operation stops to avoid the program getting stuck.
- KEEP_ALIVE: This option lets the server send special packets to keep the connection open even if no data is being exchanged. This is useful to avoid accidental disconnects during quiet periods.
Building a Simple Chat Application with Sockets:
Chat applications have become an essential part of our digital lives. They allow for real-time communication between users across networks. Let's build a basic chat application using Java sockets to solidify your understanding of the concepts discussed earlier.
Components of a Simple Chat Application:
Our chat application will consist of two main components:
- Server: The server program acts like a central chatroom, waiting for clients to connect. It listens for incoming connections and manages communication between them.
- Client: The client program lets users connect to the server, send messages to others, and receive messages from them. This allows users to chat or exchange data over the network.
Server-Side Implementation:
Here's a breakdown of the server-side code:
public class ChatServer {
public static void main(String[] args) throws IOException {
try (ServerSocket serverSocket = new ServerSocket(8080)) {
while (true) {
new ClientHandler(serverSocket.accept()).start();
}
}
}
}
class ClientHandler extends Thread {
private final Socket clientSocket;
public ClientHandler(Socket clientSocket) {
this.clientSocket = clientSocket;
}
@Override
public void run() {
try (var in = new BufferedReader(new InputStreamReader(clientSocket.getInputStream())),
out = new PrintWriter(clientSocket.getOutputStream(), true)) {
String msg;
while ((msg = in.readLine()) != null) {
System.out.println("Client message: " + msg);
}
} catch (IOException e) {
System.err.println("Error: " + e.getMessage());
} finally {
clientSocket.close();
}
}
}
Explanation:
- The server creates a ServerSocket object and binds it to a specific port (e.g., 8080).
- A while loop continuously listens for incoming client connections using accept().
- For each new client, a separate thread (ClientHandler) is created to handle communication with that specific client. This allows the server to handle multiple clients concurrently.
- The ClientHandler thread reads incoming messages from the client using an InputStreamReader and a BufferedReader.
- The received message is printed to the server console (implementation for broadcasting messages to other clients is omitted for brevity).
- In case of exceptions, the thread gracefully closes the connection and associated resources (socket, reader, writer).
Client-Side Implementation (Brief Overview):
Like the server, the client connects to the designated port. It then loops through these steps:
- Get User Input: Read the user's message.
- Send to Server: Send the message using an OutputStream (possibly wrapped in an OutputStreamWriter for encoding).
- Receive from Server: Wait for data and convert it (using InputStream and potentially InputStreamReader) before processing.
Taking the Chat App Further: Enhancing Communication and Functionality
The basic chat application provides a foundation for understanding socket communication. However, it has limitations that can be addressed through enhancements:
- Unidirectional Communication: Currently, messages only flow from the client to the server (unidirectional). To enable real-time conversation, we need to allow the server to broadcast messages back to all connected clients.
- No User Handling: The application doesn't differentiate between clients. Implementing user names or unique identifiers would enhance the user experience and allow for targeted communication.
Enhancing Communication Features:
Here are some concepts to elevate our chat application:
- Multithreading on the Server: The current implementation uses a single thread per client. For handling a large number of concurrent clients efficiently, employing a thread pool or a non-blocking I/O approach can be explored.
- User Names or Identification: Each client can be assigned a unique identifier or username upon connection. This allows for displaying usernames alongside messages, adding personality and context to the conversation.
- Targeted Communication: The basic application broadcasts messages to all clients. However, we can introduce functionality for sending messages to specific users. This could involve implementing a mechanism where clients specify recipient usernames while sending messages.
- Error Handling and Exception Management: Robust communication relies on proper error handling. Implementing exception handling allows the server to gracefully handle unexpected situations like client disconnections or network errors, preventing the application from crashing unexpectedly.
Beyond TCP: Exploring Advanced Socket Programming Concepts
While TCP (Transmission Control Protocol) provides reliable, connection-oriented communication in our chat application, there are scenarios where a different approach might be more suitable.
Datagram Sockets (UDP): Datagram Sockets use UDP, a speedy delivery service for data. Unlike some delivery services (TCP), UDP doesn't check if the package arrives. It just sends packets of data (datagrams) directly. This makes it faster, but there's a chance packets might get lost. It's perfect for things like live streams or online games where a little missing data isn't a big deal.
Advanced Socket Options and Configuration: Sockets offer various configuration options for tailoring communication behavior.
- Non-Blocking I/O: Regular sockets can make your program wait while it listens for data. Non-blocking sockets are different. They let your program check for data without getting stuck waiting. This allows a single thread to handle many connections at once, making things more efficient.
- Timeouts: Setting timeouts on socket operations like read or write can prevent applications from hanging indefinitely if communication stalls.
Security Considerations: Socket programming exposes applications to potential security vulnerabilities. Here are some key considerations:
- Authentication: Implementing mechanisms to verify the identity of communicating parties is crucial. This can involve using secure protocols like TLS/SSL for encryption and authentication.
- Encryption: Encrypting data streams exchanged through sockets protects sensitive information from eavesdropping on unsecured networks.
Conclusion:
This journey through socket programming in Java equipped you to establish connections, exchange data, and build a functional chat application. Remember, sockets unlock vast potential for network applications – from file transfers and remote procedure calls to complex distributed systems. Explore advanced topics like Datagram Sockets, security considerations, and thread management to further refine your network programming skills. The world of socket-powered applications awaits your exploration!
About the Author
Mr. William Garcia
Qualification: Master's degree in Computer Science
Expertise: Java testing advocate, ensuring code quality and application reliability.
Research Focus: Mr. Garcia leverages JUnit and Mockito for comprehensive Java testing, including unit and integration aspects. He also champions TDD practices.
Experience: Designs and implements automated testing strategies for large-scale Java projects. His focus on comprehensive testing contributes to high-quality and reliable applications.
Mr. Garcia's dedication to Java testing guarantees well-tested and reliable software. His research strengthens the foundation of code quality within Java development.