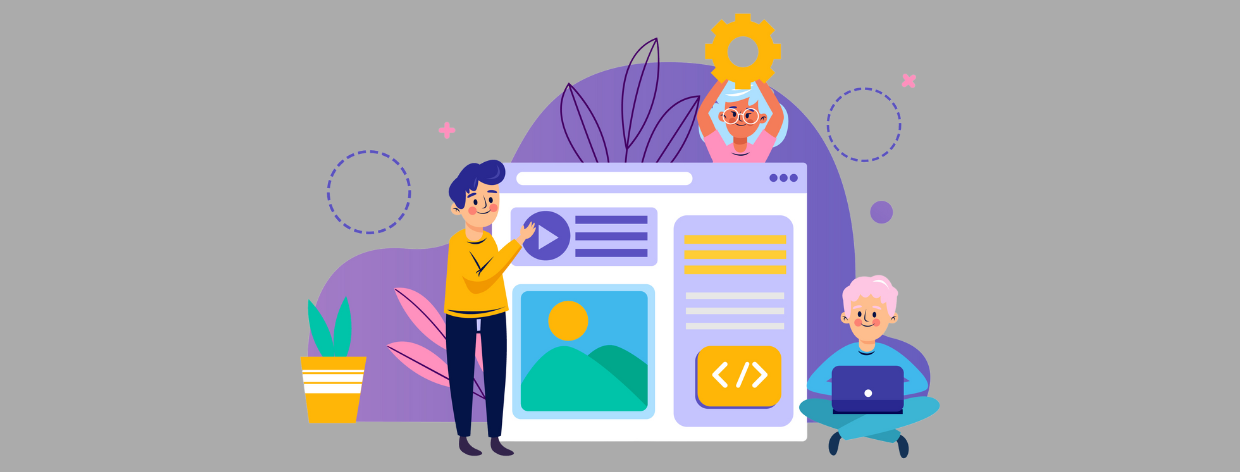
- 16th Mar 2024
- 00:22 am
- Adan Salman
In today’s world, people want software that's simple and fun to use. Graphical User Interfaces (GUIs) are the pictures and buttons you see on your computer screen – they make programs clear and engaging. Swing is a powerful tool in Java that lets you build these GUIs for your applications. With Swing, you can create user-friendly interfaces that make your programs easy and enjoyable to navigate.
Swing, a core part of the Java Foundation Classes (JFC), empowers you to build visually appealing and feature-rich user interfaces. Unlike platform-specific toolkits, Swing boasts:
- Platform Independence: Swing takes benefit of Java's "write once, run everywhere" philosophy, making it a breeze to set up your application across Windows, Mac, and Linux systems. No count matter what operating system your users have, they'll experience the identical interface in your Swing application.
- Rich Component Set: Swing provides a toolbox full of ready-made UI components you can use to build your applications. These include buttons, text fields, menus, tables, and more. This wide variety lets you create all sorts of user interfaces for your programs.
- Customization and Flexibility: Swing gives you control over how your app looks. Change fonts, colors, and borders to create a unique style. You can also arrange buttons, menus, and other elements however you like to make your app both visually appealing and easy to navigate.
With Swing at your disposal, you unlock the potential to create interactive and user-centric Java applications that resonate with your audience. Master Swing, the powerful toolkit for crafting user-friendly interfaces! Conquer Swing concepts and design interactive applications with our expert Java Assignment Help and Java Homework Help, and build GUIs that impress!
Core Swing Components
Swing gives you a box full of ready-to-use pieces for your applications. These include buttons, menus, and more, like building blocks. They let you easily create interfaces that are user-friendly, making your programs easy to use.
Components: The Cornerstones of Swing GUIs
Imagine your Swing GUI as a canvas composed of various elements. These elements are the components, the individual building blocks that provide functionality and visual appeal. Here's a closer look at some essential Swing components:
- JPanels: These act as containers that group other components within your GUI. They don't display anything by themselves but provide a way to organize and structure your layout. Panels help maintain a clean and organized visual hierarchy.
- JLabels: These versatile components are used to display static text or even images within your GUI. Labels are ideal for providing captions, instructions, or informative text to guide users.
- JTextFields: This component allows users to enter single-line text input. Text fields are essential for capturing user data, search queries, or any information requiring user interaction.
- JButtons: These clickable buttons trigger actions within your application when pressed. Buttons can initiate calculations, launch specific functionalities, or perform various tasks based on your program's logic.
- JCheckBoxes and JRadioButtons: These components handle boolean choices where users can select one option (radio button) or multiple options (checkboxes) from a set of choices. They are ideal for representing preferences, filters, or selection criteria.
- JTextAreas: This component provides a multi-line text input and display area. It's perfect for scenarios where users need to enter or view longer passages of text, descriptions, or detailed information.
- JList and JTables: Swing provides components to show data in your GUI, JList - Great for lists of items (like choices or results). JTable - Perfect for tables with rows and columns (like spreadsheets).
- JMenus and JMenuItems: Swing's JMenus and JMenuItems mimic familiar website menus, organizing actions within your application. The main menu bar sits at the top, with individual options within each menu. This clear structure helps users find what they need quickly.
Customizing Your Components:
Swing components are highly customizable. You can tailor their appearance using properties like:
- Font: Set the font style, size, and color for text displayed within components.
- Foreground and Background Colors: Control the background color of the component and the color of the text or image it displays.
- Borders: Define the border style (e.g., solid, dashed) and its color for visual distinction and organization.
Layout Managers: Arranging Your Components
While components offer the building blocks, layout managers dictate how these elements are positioned and arranged within your GUI. We'll delve deeper into layout managers in the next section, but understanding their role is crucial for creating visually appealing and well-organized user interfaces.
Shaping Your GUI: Layout Management in Swing
Layout managers in Swing arrange UI elements (buttons, menus) within containers (panels, windows). They dictate placement and size, creating a well-structured interface.
The Power of Layout Managers:
Layout managers in Swing act like organizers for your app's buttons, menus, and other pieces (components). They arrange them neatly, just like putting things on shelves. This makes your application's interface easy to use and navigate for everyone.
Common Layout Managers in Swing:
Swing offers various layout managers to organize your app's elements (buttons, menus). Let's explore some popular ones!
- FlowLayout: This common layout arranges Swing elements (buttons, menus) left to right, wrapping to new lines. Great for basic layouts, but not ideal for complex ones.
Example:
JPanel panel = new JPanel(new FlowLayout());
panel.add(new JButton("Button 1"));
panel.add(new JLabel("Enter your name:"));
panel.add(new JTextField(15));
- BorderLayout: This layout manager divides the container into five regions: north, south, east, west, and center. You can add components to specific regions for a structured layout.
Example:
JPanel panel = new JPanel(new BorderLayout());
panel.add(new JLabel("Welcome!", BorderLayout.NORTH));
panel.add(new JButton("Click Me"), BorderLayout.SOUTH);
panel.add(new JTextArea(), BorderLayout.CENTER);
- GridLayout: This layout manager puts your Swing components (buttons, menus) in a neat grid, like squares on a checkerboard. All the elements are the same size, making it perfect for showing lists or tables in a clean and organized way.
Example:
JPanel panel = new JPanel(new GridLayout(2, 3));
panel.add(new JButton("Button 1"));
panel.add(new JButton("Button 2"));
panel.add(new JButton("Button 3"));
// Add more buttons to fill the grid (total of 6)
- GridBagLayout: GridLayout lets you organize app elements in a grid, offering more control over placement and size. Great for complex layouts, but requires more setup.
Choosing the Right Layout Manager:
The best layout manager for your Swing GUI depends on the number of components, their arrangement, and the desired visual hierarchy. Experiment with different options like BorderLayout (for a few core regions) or FlowLayout (for dynamic elements) to achieve a well-organized and user-friendly interface.
Event Handling in Swing
A beautiful and static interface isn't enough in today's world. Swing applications come alive through event handling, the mechanism that enables user interaction and responsiveness. Let's explore how events and event listeners work together to create dynamic and engaging GUIs.
The Power of Events:
Events are signals generated by user actions or system occurrences within your Swing application. These actions can include clicking a button, entering text in a field, or even resizing the application window. By handling these events, you can make your GUI react and perform specific tasks based on user interaction.
Event Listeners:
Swing uses "watchers" called event listeners to keep track of what users do with your app's buttons, menus, and other parts (components). When something happens, like a button click, the watcher tells your program to react in a specific way. This lets you control what your program does based on user interactions.
Attaching Event Listeners:
Swing's watchers (event listeners) let your app respond to user interactions. Add listeners with methods like addActionListener (button clicks) or addTextListener (text changes). This makes your program interactive!
Code Example:
Imagine a button that displays a message when clicked. Here's how you would achieve this functionality using event handling:
JButton button = new JButton("Click Me");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(null, "Button Clicked!");
}
});
In this example, an ActionListener object is created to handle the actionPerformed event (button click). This listener object defines the logic to be executed (displaying a message dialog) when the button is clicked.
Beyond Button Clicks:
Swing's watchers (event listeners) can listen to many things, not just button clicks. They can watch for things like - Text changes in typing boxes (TextListener), The window getting bigger or smaller (ComponentListener), And many more! This lets your program react in different ways depending on what the user does or what happens on the computer. This makes your program more interactive and enjoyable to use.
Menus and Dialogs: Enhancing User Interaction in Swing
Swing provides essential UI elements that go beyond basic components – menus and dialogs. These tools elevate user interaction within your applications.
Menus (JMenus) and Menu Items (JMenuItems):
Menus offers a hierarchical structure for organizing functionalities within your GUI. They appear at the top of the window, typically containing JMenuItems that represent specific actions or options. Submenus can be nested within menus to further organize related options.
Here's an example of creating a menu with a submenu and handling menu item selection:
JMenuBar menuBar = new JMenuBar();
JMenu fileMenu = new JMenu("File");
JMenuItem openItem = new JMenuItem("Open");
fileMenu.add(openItem);
menuBar.add(fileMenu);
openItem.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
// Code to handle opening a file
}
});
Dialogs (JDialog):
Dialogs are specialized windows that appear on top of your main application window. They are used for various purposes like:
- Modal Dialogs: These block interaction with the main window until the user interacts with the dialog (e.g., confirmation dialogs).
- Non-Modal Dialogs: These allow users to interact with both the dialog and the main window simultaneously (e.g., progress dialogs).
Here's a simple example of creating a modal dialog to display a message:
JDialog dialog = new JDialog(frame, "Message");
dialog.setModal(true);
JLabel messageLabel = new JLabel("This is a message dialog!");
dialog.add(messageLabel);
dialog.pack();
dialog.setVisible(true);
By incorporating Menus and dialogs in Swing applications makes things easier for users. They provide a clear way to find options and interact with your program, leading to a more enjoyable experience.
Advanced Swing Concepts
While we've explored core Swing functionalities, the journey doesn't end here! Swing offers a rich ecosystem for crafting sophisticated GUIs. Here's a glimpse into some advanced topics:
- Custom Components: Need a specialized UI element beyond standard components? Swing allows you to extend existing components or create entirely custom ones to meet your specific needs.
- Internationalization (I18N) and Accessibility: Swing supports I18N, enabling you to localize your application for different languages and regions. Additionally, accessibility features cater to users with disabilities, ensuring your GUIs are usable by everyone.
- Advanced Layout Managers: MigLayout and FormLayout provide powerful options for complex layouts with more granular control over component positioning and constraints. These layouts are ideal for scenarios requiring intricate UI arrangements.
Exploring these advanced concepts and leveraging Swing's full potential empowers you to build feature-rich, user-centric, and visually appealing applications that cater to a wider audience.
Conclusion:
This hands-on Swing tutorial has equipped you to build user-friendly Java applications. You've explored core components, layout management, event handling, and essential UI elements. Now, leverage Swing's potential! Craft custom components, explore advanced layout managers, and delve into internationalization and accessibility.
Don't stop here! Utilize the Swing API documentation, online tutorials, and GUI builder tools within IDEs like NetBeans or Eclipse. With continued practice, you'll be building interactive Swing applications that impress!
About the Author
Ms. Sophia Chen
Qualification: Master's degree in Computer Science
Expertise: Java GUI programming maestro, crafting user-friendly and visually appealing desktop applications with Swing.
Research Focus: Ms. Chen researches how Swing can better align with HCI principles, focusing on accessibility, user experience, and leveraging Swing's advancements.
Experience: She builds interfaces for computer programs (desktop applications) that are used in many different fields. Her essential aim is to create packages that are not only useful but additionally smooth and exciting to use (user-focused design).
Ms. Chen's expertise bridges Swing and HCI, developing user-friendly interfaces that are both lovely and a pleasure to apply.