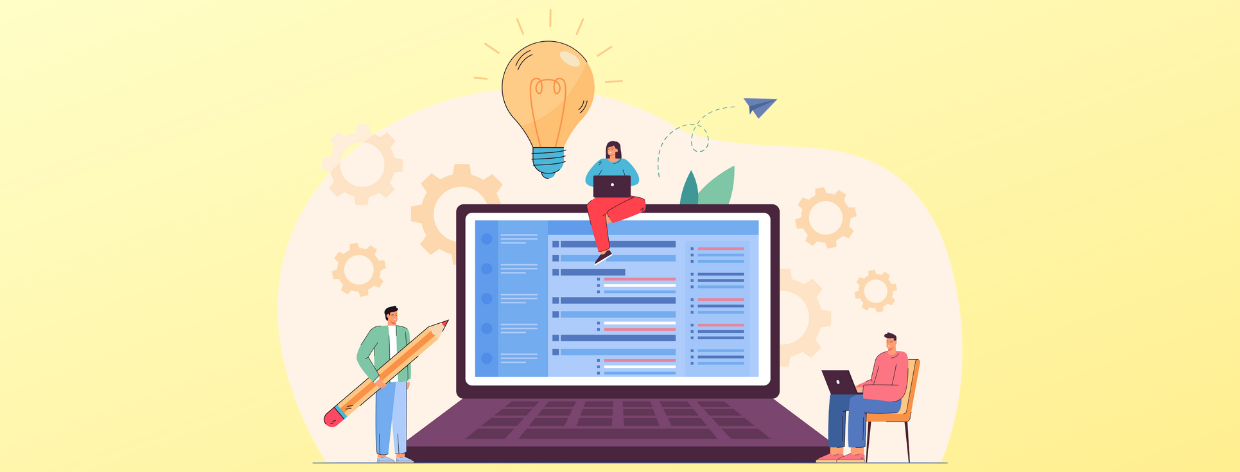
- 11th Mar 2024
- 21:51 pm
- Mr. David Lee
Java is a powerhouse programming language driving the creation of modern software. Its popularity stems from its adaptability, ability to run on various computer systems (platform independence), and easy-to-understand structure (syntax). This makes Java the perfect tool for building complex applications across diverse fields.
Building a strong foundation in Java starts with understanding its key concepts. This guide explores the 10 most important ones, offering a launchpad for beginners and a helpful reminder for experienced programmers.
Master the fundamentals of Java programming and Conquer Java's core concepts with our excellent Java assignment help and Java homework help service and Level up your Java skills!
The 10 Most Important Java Concepts
Ready to unlock the power of Java? Here's a roadmap to the 10 important concepts that shape the foundation of effective Java programming.
1. Data Types:
Data types define the kind of information a variable can preserve. They act because of the language utilized by your program to understand and control different types of data. Java offers two primary categories: primitive and non-primitive data types.
Primitive Data Types: These fundamental data types represent basic building blocks and are directly supported by the underlying hardware.
They have fixed sizes in memory and include:
- int: Stores integers (whole numbers) - typically 4 bytes. (e.g., -2147483648 to 2147483647)
- double: Stores floating-point numbers (numbers with decimals) - typically 8 bytes.
- char: Stores a single character (e.g., 'a', '!', '$') - typically 2 bytes.
- boolean: Represents logical values (true or false) - typically 1 byte.
- Other primitive types like byte, short, and long cater to specific memory usage requirements.
Non-Primitive Data Types: These represent more complex data structures that are built upon the primitive types. They provide mechanisms for storing collections of data or referencing objects in memory.
Examples include:
- String: It holds a set amount of text and can not be changed after it's created.
- Arrays: Ordered lists that group elements of the identical data type in Java.
- Objects: Building blocks in Java, objects bundle data (what something is) and actions (what it can do).
2. Variables:
Variables act as named boxes that hold facts of specific types. Declaring a variable includes specifying an information type and a name that follows Java's naming conventions (starting with a letter, followed by way of letters, numbers, or underscores).
int age = 25; // Declares an integer variable 'age' and assigns the value 25
double pi = 3.14159; // Declares a double variable 'pi' and assigns the value of pi
Scope rules determine the visibility and lifetime of variables within your program. Local variables declared inside methods are only accessible within that method, while class-level variables (also referred to as member variables) are accessible during the class.
3. Operators:
Operators are symbols used to perform various operations on data. Java provides a rich set of operators, categorized as:
- Arithmetic Operators: Perform basic mathematical calculations like addition (+), subtraction (-), multiplication (*), division (/), and modulo (%).
- Logical Operators: Evaluate conditions and return boolean values (true or false). Examples include && (AND), || (OR), and ! (NOT).
- Comparison Operators: Compare values and return boolean results. Examples include == (equal to), != (not equal to), < (less than), > (greater than), <= (less than or equal to), and >= (greater than or equal to).
- Assignment Operators: Assign values to variables. The basic assignment operator is =, but there are compound assignment operators like += (add and assign) and -= (subtract and assign) for shorthand operations.
The order you do math in an expression affects the answer, just like following steps in a recipe. PEMDAS (Parentheses, Exponents, Multiply/Divide, Add/Subtract) is the key. Follow it to avoid surprises!
4. Control Flow Statements:
Control flow statements dictate the execution sequence of your program's instructions. They allow you to make decisions based on conditions and execute code blocks repeatedly.
Conditional Statements:
- If-else: Executes a block of code if a circumstance is authentic, and optionally offers an opportunity block to execute if the condition is fake.
- Transfer: Allows for multi-manner branching based totally on the cost of an expression, comparing it in opposition to more than one case statement.
Loop Statements:
- for: Executes a code block repeatedly for a predetermined number of iterations, typically controlled by a loop counter.
- while: Executes a code block again and again so long as a distinct condition stays true.
- do-while: Executes a code block as a minimum once, then maintains looping as long as a circumstance is true.
Understanding data types, variable operators, and managing flow statements equips you with the important tool to govern information, make decisions within your program, and control its execution flow.
5. Classes & Objects:
Imagine a class as a blueprint for creating objects. It defines the attributes (data) and methods (behaviors) that an object of that class will possess. Think of it like a template for a car - the class defines the car's properties (e.g., color, number of wheels, engine type) and functionalities (e.g., acceleration, brake, turn).
Objects, on the other hand, are individual instances created from a class blueprint. Each object has its own set of attribute values, representing the specific state of that object. For instance, you can create multiple Car objects with different colors, engine types, and current speeds.
Creating objects involves the use of the new keyword observed by means of the magnificence call and optionally providing arguments for the duration of initialization. Once an object is created, you may access its attributes and strategies using dot notation (object_name.attribute_name or object_name.method_name()).
6. Encapsulation:
Encapsulation is like building a secure box for your data in Java. It hides the data (attributes) inside a class and provides special tools (methods) to access and change it. This keeps your data safe from outside interference and ensures it's used properly.
Imagine public members as things anyone can access, while private members are like hidden compartments only the class itself can reach. Protected members are accessible from the class itself and its subclasses, promoting controlled inheritance behavior.
Encapsulation offers several benefits:
- Improved Data Integrity: By controlling access to data, encapsulation prevents unintended modification from outside the class, making sure of data consistency and reliability.
- Modularity: Encapsulation promotes modularity with the aid of separating the inner implementation details of a class from its public interface. This makes code easier to apprehend, maintain, and reuse.
- Reduced Coupling: Encapsulation minimizes dependencies between classes by restricting direct access to internal data. This makes the code more flexible and adaptable to changes.
7. Inheritance:
Inheritance allows you to create hierarchical relationships between classes, promoting code reusability and maintainability. A subclass inherits all the attributes and techniques of its superclass (parent class). This provides a foundation for building specialized classes without duplicating common code.
Here's the basic concept:
- Superclass: The base class that defines the general properties and behaviors shared by its subclasses.
- Subclass: A class that inherits from a superclass, specializing in its functionality and potentially overriding or extending inherited methods.
Inheritance offers several advantages:
- Code Reusability: By inheriting common functionality from a superclass, subclasses keep away from code duplication, which saves development time and effort.
- Code Maintainability: Changes that are made to the superclass automatically propagate to its subclasses, simplifying code maintenance.
- Polymorphism: Inheritance lays the basis for polymorphism, permitting objects of numerous subclasses to respond in every other way to the same approach call.
8. Polymorphism:
Polymorphism refers back to the ability of objects of different classes to reply otherwise to the equal method call at runtime. This flexibility allows you to write generic code which can work with numerous objects without knowing their exact types ahead.
There are two main mechanisms for achieving polymorphism in Java:
- Method Overloading: Like having multiple tools with different functions but the same name, methods with the same name but different parameters let the compiler choose the right one.
- Method Overriding: Subclasses can redefine inherited methods, creating specialized versions for their specific needs. Think of customizing tools for a particular job.
9. Exception Handling:
No program is perfect, and unexpected situations can arise during execution. Exception handling provides a mechanism to gracefully manage these runtime errors or unexpected events. Instead of letting your program crash, you can anticipate potential issues and provide alternative behavior.
The primary tool for exception handling is the try-catch block:
- try block: Encloses the code that might throw an exception.
- catch block: Captures a specific exception type and defines the code to execute if that exception occurs within the try block.
You can have multiple capture blocks to handle distinct sorts of exceptions. Additionally, an end block may be used to execute code that usually runs after the strive block finishes, irrespective of whether an exception was thrown.
Throwing exceptions allows you to signal errors to calling methods. By throwing exceptions from within methods, you can propagate error information up the call stack, enabling robust error handling throughout your application.
10. Interfaces:
Imagine an interface as a contract that outlines what a class must do but not how it does it. It specifies methods that a class must implement, without dictating the implementation details. This promotes loose coupling, meaning classes that implement an interface are not tightly bound to the specific implementation details.
Interfaces often contain abstract methods, which lack an implementation within the interface itself. Concrete classes that implement the interface must provide implementations for these abstract methods.
Here are some key benefits of interfaces:
- Loose Coupling: Interfaces promote loose coupling between classes. A class that implements an interface relies on the behavior defined by the interface, not the specific implementation details of another concrete class. This improves code flexibility and maintainability.
- Abstraction: Interfaces promote abstraction by focusing on the "what" (functionality) rather than the "how" (implementation details). This allows you to define generic behaviors that can be implemented differently by concrete classes.
- Polymorphism: Interfaces are like a universal remote in Java. Different objects can be controlled the same way if they follow the same interface (button layout). This lets you write flexible code that works with various objects without needing to know their exact type.
11. Packages & The Collections Framework:
As your programs grow in complexity, managing numerous classes becomes essential. Packages provide a way to organize classes within a hierarchical structure. Think of them as folders for grouping related classes, preventing naming conflicts, and improving code maintainability.
The Java Collections Framework is a rich set of classes and interfaces that provide proficient approaches to managing collections of items. It gives a whole lot of data structures like lists, sets, and maps, each with distinct functionalities for organizing and accessing factors. For instance, lists maintain the order of elements, while sets ensure unique elements, and maps allow key-value associations.
Conclusion:
Mastering these 10 fundamental Java concepts equips you with the essential tools to construct well-structured and efficient programs. From understanding data types and manipulating them using operators to controlling program flow and leveraging object-oriented principles, you'll gain the ability to translate real-world problems into effective Java solutions. Remember, consistent practice writing Java code is key to solidifying your understanding. As you progress, explore advanced features and libraries to unlock the full potential of Java and build robust applications that meet your programming needs.
About the Author
Mr. David Lee
Qualification: Master's in Computer Science
Expertise: Java guru, crafting complex enterprise applications. Focuses on design patterns for clean, maintainable code and optimizes back-end systems for performance and security.
Experience: Leads Java development teams, building robust back-end systems for critical business applications. Leverages Java frameworks to create efficient, feature-rich applications.
Mr. Lee's Java mastery empowers him to design and implement complex systems, making him a valuable asset in any software development team.