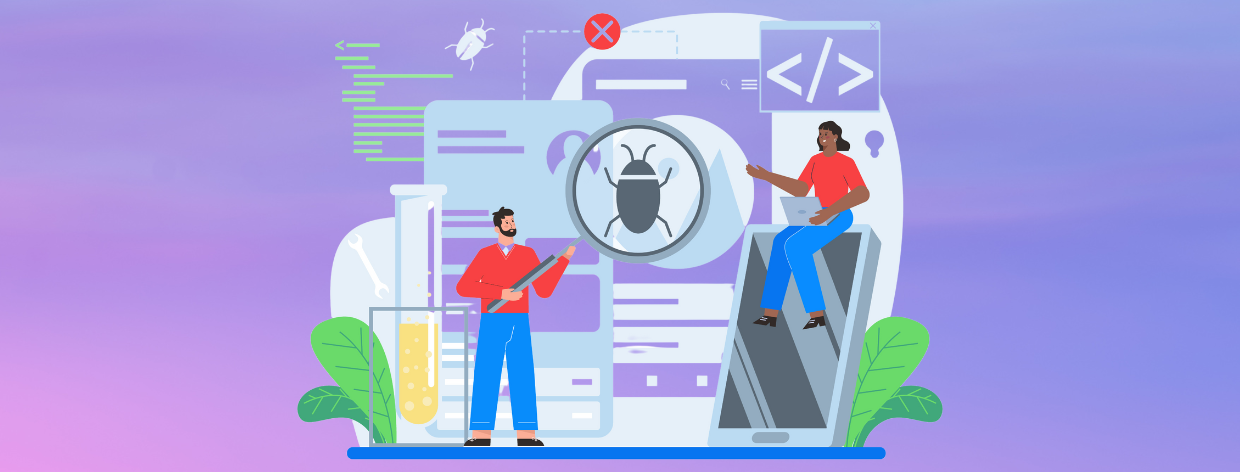
- 12th Mar 2024
- 00:18 am
- Adan Salman
The path of a software developer is rarely a straight line. Bugs, errors, and unexpected behavior are like unexpected detours on your coding journey. This is where debugging comes in – your secret weapon for identifying, isolating, and fixing these roadblocks within your code. Mastering debugging is essential for building strong and reliable Java applications.
Java programmers encounter a wide range of debugging challenges. From sudden crashes caused by runtime exceptions to subtle logic errors that produce incorrect results, these issues can range from straightforward to head-scratching. Typos, missing data references, and endless loops – the list goes on!
The debugging process itself can feel like a puzzle, combining symptom analysis with detective work to find the root cause and implement a fix. But don't worry! By learning the available tools and techniques, you can transform from a frustrated coder to a debugging champion. This guide equips you with the knowledge and strategies to confidently tackle any debugging hurdle you encounter.
Struggling to decipher error messages and get your code running smoothly? Master the art of debugging with our comprehensive Java assignment help and Java homework help service. Take control and become a debugging pro today!
Essential Debugging Tools
The right tools can supercharge your Java debugging skills. Here, we'll explore three allies to conquer those coding roadblocks:
IDEs: Your All-in-One Toolkit
Think of IDEs (Integrated Development Environments) as the Swiss Army knives of development. Popular options like IntelliJ IDEA, Eclipse, and NetBeans offer a treasure trove of features designed to streamline coding and debugging:
- Breakpoints: Hit the pause button! Set breakpoints to halt your code and examine its state at any point.
- Variable Inspection: Like peeking under the hood, you can view variable values at any time, ensuring data integrity and spotting inconsistencies.
- Stack Trace Analysis: Imagine rewinding the tape. Analyze the chain of method calls leading to an error, pinpointing the exact location where it all began.
- Code Stepping: Take it slow and steady. Step through your code line by line, observing variable changes and execution flow in a controlled manner.
- Visualizations (Bonus Feature!): Some IDEs offer visual representations of data structures and program flow, making complex interactions crystal clear.
Command-Line Debuggers: Powerful and Flexible
While IDEs offer a user-friendly interface, command-line debuggers like jdb (part of the JDK) provide a different approach. They offer similar functionalities but through text-based commands. Here's why command-line debuggers might be your secret weapon:
- Lightweight: These debuggers take up less space and memory, making them perfect for computers with limited resources.
- Remote Debugging: Fix problems in applications running on remote machines, ideal for troubleshooting server-side issues.
- Scripting Automation: Repetitive tasks are a thing of the past. Write scripts for the debugger to automate common debugging actions.
Logging Frameworks: Leaving a Trail of Clues
Imagine debugging a complex issue in a finished application. That's where logging frameworks like Log4j and SLF4J come in handy. These tools let you sprinkle "breadcrumb" messages throughout your code. These messages capture details about what's happening at different points (information, debug messages, errors).
Logging brings valuable benefits:
- Detailed Information: Logs record variable values, method calls, and timestamps, providing a rich history of program execution.
- Remote Monitoring: Send logs to a central server for real-time monitoring of live applications.
- Unraveling Complexities: Analyze log entries to find patterns and pinpoint the root cause of tricky issues.
Mastering Breakpoints
Breakpoints are the cornerstones of effective debugging in Java. They act as strategic pause points within your code, allowing you to halt execution at specific locations and examine the program's state. By strategically using breakpoints and stepping options, you can gain valuable insights into the behavior of your code and pinpoint the source of errors.
- Setting Breakpoints in Your IDE:
Most IDEs offer intuitive ways to set breakpoints. You can click on the line number in your code editor or right-click on a line and select "Set Breakpoint." Alternatively, you can use keyboard shortcuts for faster placement. Once set, a red dot or similar visual cue will typically appear next to the line of code, indicating the breakpoint's location.
- Conditional Breakpoints for Targeted Debugging:
Breakpoints are great for pausing your code, but what if you only want to pause when something specific happens? Enter conditional breakpoints! These allow you to set a condition that must be true for the breakpoint to stop your code.
With a conditional breakpoint, you can pause only when a specific variable reaches a certain value, or maybe only when a particular method is called during a specific loop iteration. This targeted approach lets you focus on the exact part of your code causing problems, saving you time and frustration.
- Stepping Through Code Line by Line:
Once you've set your breakpoints, it's time to leverage the power of stepping. Stepping allows you to execute your code line by line, observing how variables change and how the program flow unfolds. This controlled environment provides a valuable opportunity to analyze the program's behavior in detail.
Most IDEs offer various stepping options, each with its own purpose:
- Step Over: This option executes the current line of code and then moves to the next line, skipping any method calls that might occur on the current line. This is useful for following the main flow of your code.
- Step Into: This option steps into any method calls that occur on the current line. This allows you to examine the internal behavior of methods and identify potential issues within them.
- Step Out: This option steps out of the current method, returning to the line where the method was called. This is helpful for quickly navigating out of nested method calls and focusing on the higher-level code flow.
By strategically choosing the right stepping option, you can efficiently navigate through your code, pinpoint where issues arise, and gain a deeper understanding of how your program functions.
- Examining Variable Values and Execution Flow:
Your IDE is your debugging ally. Combine these techniques to conquer those bugs and transform errors into clear solutions. Here's how to use its power:
- Variable Inspection: Pause your code and see what's inside! Check variable values to ensure they hold the expected data and identify any unexpected changes causing issues.
- Code Stepping: Take it slow. Step through your code line by line, observing variable changes and program flow.
- Visual Aids (Bonus!): Some IDEs offer visual representations of data structures and program flow, making complex scenarios easier to grasp.
Understanding Stack Traces
When your Java program crashes with an error, a message called a "stack trace" appears. It might look overwhelming at first, but this stack trace is actually your friend! It acts like a roadmap, guiding you to the exact spot where the error happened in your code. Let's explore what information it provides and how to use it to solve problems effectively.
- Unveiling the Stack Trace:
A stack trace typically displays a list of method calls, starting with the exception that occurred and working its way back to the line in your code that triggered it. Each line represents a method call on the stack, showcasing:
- Method Name: This identifies the method that was executed when the exception occurred.
- File Name and Line Number: This pinpoints the exact line of code within the file where the exception originated.
- Local Variables: Some stack traces might display the values of local variables within the method at the point of the exception. This can offer valuable insights into the state of the program at that specific moment.
- Identifying the Root Cause:
Don't fear the stack trace! This list of method calls in a Java error actually guides you to the problem's source. The error message is just the end result.
Work your way up the stack trace to find the exact line causing the issue. It might be the direct cause (using a missing object) or an indirect one that triggered a chain of events. Analyze the logic and data flow within each method call to pinpoint the culprit. This focused approach helps you fix the problem and prevent future ones.
- Decoding Common Exceptions with Stack Traces:
- NullPointerException: This error pops up when you try to use something that doesn't actually exist in your code (null reference). The stack trace will show you the line where this happened. Look for methods that might accidentally return "nothing" (null) or variables that haven't been given a value yet.
- IndexOutOfBoundsException: This error means you've tried to access an item in a list or array that doesn't exist. Imagine an overflowing basket – you can't take something out if it's not there! The stack trace will show the line where this out-of-bounds access occurred. Review your calculations and loop conditions to make sure you're staying within the valid range of items in your list or array.
- ArithmeticException: This exception arises from division by zero or other invalid mathematical operations. The stack trace will guide you to the line where the problematic operation is performed. Review your calculations and handle potential division by zero scenarios gracefully.
Advanced Debugging Techniques
While mastering breakpoints and stack traces equip you for core debugging tasks, the world of Java development throws more complex challenges your way. Here, we explore advanced debugging techniques to tackle multithreading, memory leaks, and remote applications.
- Debugging Multithreaded Applications:
Things get trickier when your Java program involves multiple threads running at once. These threads can cause issues if they don't access shared data properly (race conditions) or get stuck waiting on each other forever (deadlocks).
Here are some tools to help you debug these multithreaded monsters:
- Thread Dumps: Thread dumps show which threads are running, waiting, or stuck. By analyzing these snapshots, you can spot potential deadlocks or situations where threads are fighting over resources, helping you fix the problem.
- Synchronized Debugging: Some IDEs offer synchronized debugging capabilities. This allows you to step through code execution while considering the behavior of all threads, providing a more holistic view of your multithreaded program's behavior.
- Logging with Thread Identification: Enhance your logging statements to include thread identifiers. This will help you correlate log messages with specific threads, simplifying the analysis of concurrent activities.
- Debugging Memory Leaks:
Memory leaks happen when your program hoards objects it doesn't need. This can slow things down or even crash your app.
Here are some techniques for detecting and preventing memory leaks:
- Memory Profilers: These tools track memory usage and identify objects the garbage collector misses. Fix the leak by finding the culprit object and the code that created it.
- Weak References: Not all objects need to be permanent residents. Use weak references to let the garbage collector reclaim memory from unused objects.
- Code Review and Best Practices: Regular code reviews and best practices (closing resources, avoiding needless object references) also help prevent leaks.
- Debugging Remote Applications:
Debugging applications running on a remote server can pose a challenge. However, several strategies can streamline this process:
- Remote Debuggers: Tools like jdb let you fix Java apps on remote machines. Set breakpoints, step through code, and inspect variables – all remotely. Perfect for distributed development and server-side issues.
- Logging and Monitoring: Implement robust logging mechanisms on your remote application. This allows you to capture detailed information about its execution and identify potential issues remotely.
- Debugging Frameworks: Consider using frameworks designed for remote debugging. These frameworks often provide additional features like centralized log collection and visualization tools, facilitating troubleshooting of remote applications.
Conclusion:
This guide has provided a roadmap to conquer debugging challenges in your Java development journey. You've learned to leverage breakpoints, decipher stack traces, and navigate the complexities of multithreaded applications, memory leaks, and remote debugging.
But remember, debugging is a continuous learning process. As you explore advanced techniques and encounter new challenges, embrace the opportunity to refine your skills. With dedication and practice, you'll transform yourself into a debugging pro, ensuring the unwavering reliability of your Java applications.
About the Author
Dr. Andrew Young
Qualification: Ph.D. in Computer Science
Expertise: Java architect, crafting high-performance enterprise applications. Leverages Spring Boot and microservices for scalability and maintainability.
Research Focus: Integrates cloud computing and AI with Java, optimizing code for distributed environments and seamless AI integration.
Experience: Leads Java dev teams for large organizations, delivering complex applications meeting performance and security standards.
Dr. Young's expertise in modern Java architecture and ongoing research make him a leader in building adaptable enterprise applications.