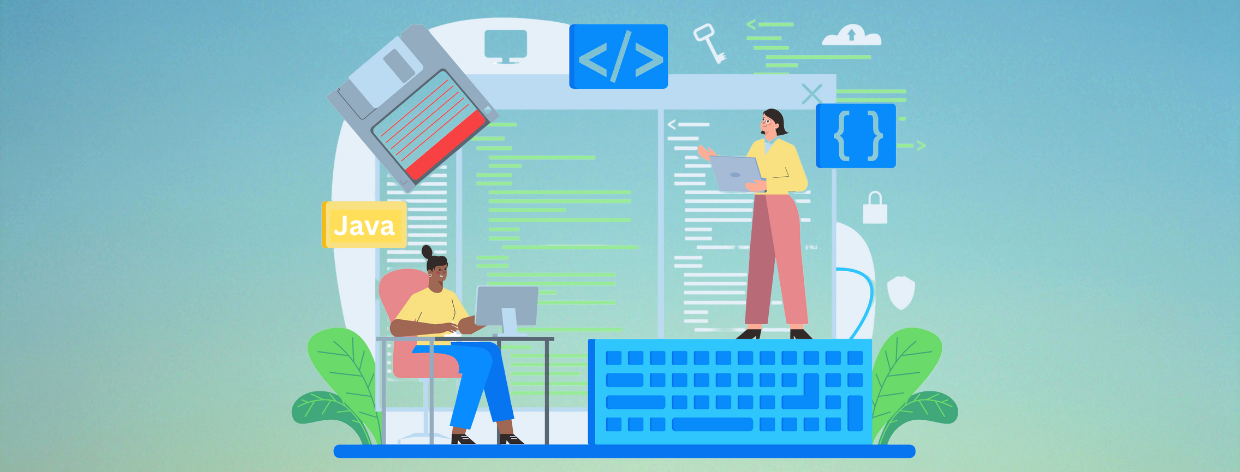
- 12th Mar 2024
- 22:39 pm
- Admin
Effective data organization is a cornerstone of efficient object-oriented programming. In Java, the Java Collections Framework (JCF) empowers developers with a comprehensive suite of interfaces and implementations designed specifically for managing collections of objects.
Think of the JCF as a specialized toolkit for grouping your data. These groups, called collections, enhance code readability, maintainability, and ultimately, program performance. Here's why the JCF is a valuable asset:
- Improved Code Clarity: Structured data leads to cleaner, more readable code, akin to a well-organized workspace.
- Enhanced Reusability: The JCF promotes code reusability by enabling you to develop logic that works with various collection types. This saves time and reduces development effort.
- Optimized Performance: The JCF offers diverse collection implementations, some demonstrably faster than others. Selecting the appropriate collection type can significantly improve program execution speed.
Subsequent sections will delve into the various JCF collection types and explore strategies for effectively organizing your data. By mastering the JCF, you'll transform into a data management pro, ensuring your code remains well-structured and your programs run efficiently.
Struggling to organize your data in Java projects? Master the Java Collections Framework with our comprehensive Java Assignment Help and Java Homework Help! Learn to write clean, efficient, and reusable code. Take control of your data structures today!
Core Interfaces and Implementations in JCF
The Java Collections Framework (JCF) empowers you to organize your data effectively. Let's delve into four fundamental collection types: Lists, Sets, Maps, and Queues. Understanding their functionalities and choosing the right implementation is key to efficient data management in your Java applications.
1. Lists: Ordered Sequences
The List interface represents an ordered collection of elements. You can add, remove, access, and search elements based on their position (index) within the list. Common functionalities include:
- add(element): Adds an element to the end of the list.
- remove(index): Removes the element at a specific index.
- get(index): Retrieves the element at a specific index.
- indexOf(element): Returns the index of the first occurrence of an element (or -1 if not found).
Common List Implementations:
- ArrayList (Resizable Array): This is a fast choice for random access (accessing elements by index) but slower for insertions/deletions in the middle. Use ArrayList when frequent random access is required and the order of elements matters.
- LinkedList (Linked Nodes): Offers fast insertion/deletion at any position but slower random access. Use LinkedList when frequent insertions/deletions in the middle are needed or when you need the flexibility of manipulating elements as a doubly-linked list.
- Vector (Legacy): Similar to ArrayList but thread-safe (synchronized for concurrent access), making it less performant for single-threaded environments. Its use is generally discouraged in favor of ArrayList for most modern Java applications.
2. Sets: Collections of Unique Elements
The Set interface represents a collection that ensures all elements are unique. It doesn't allow duplicates and provides methods for adding, removing, and checking if an element exists. Common functionalities include:
- add(element): Attempts to add an element to the set. Returns false if the element already exists (ensuring uniqueness).
- remove(element): Removes an element from the set if it exists.
- contains(element): Checks if a specific element exists in the set.
Common Set Implementations:
- HashSet (Hash-based): Java's HashSet excels at fast lookups (finding elements) thanks to hashing. The order elements are stored doesn't matter. Use HashSet when quick lookups are a priority and order is irrelevant.
- TreeSet (Sorted Tree): TreeSet keeps your Java elements sorted (like files!), making them easy to find in order or within a range. It's slower for adding/removing elements, but perfect for ordered iteration or range-based searches.
3. Maps: Key-Value Pair Associations
Java's Map interface lets you manage collections like a keycard system. Each key is unique and unlocks its associated value. Maps provide a powerful way to organize data using unique identifiers, making information retrieval and management efficient.
Maps offer functions to:
- Add (put): Insert a key-value pair. If the key exists, update the value.
- Get: Retrieve the value for a specific key (or null if not found).
- Remove: Delete the key-value pair linked to a key.
- Check (containsKey): See if a particular key exists in the Map.
Common Map Implementations:
- HashMap (Hash-based): HashMaps rule Java for super-speedy lookups by key. Imagine a dictionary - fast key searches! They don't care about the order you add things though. Use HashMaps for: Fast key lookups and Order doesn't matter
- TreeMap (Sorted Tree): TreeMap sorts keys in Java (natural or custom order). Slower for adding/removing, but excels at finding elements and searching within key ranges. Choose TreeMap when order or range-based key searches are crucial.
- LinkedHashMap: Unlike most maps, LinkedHashMap remembers the order you add elements. This lets you loop through them in the same order they were added. Use LinkedHashMap when the order elements are inserted matters.
4. Queues: Ordered Collections with FIFO Behavior
The Queue interface represents a collection that follows the FIFO (First-In-First-Out) principle. Elements are added to the back (enqueue) and removed from the front (dequeue). Common functionalities include:
- offer(element): Attempts to add an element to the back of the queue (may throw an exception if the queue is full).
- poll(): Retrieves and removes the element at the front of the queue (or null if the queue is empty).
- peek(): Retrieves the element at the front of the queue without removing it (or null if the queue is empty).
Common Queue Implementations:
- PriorityQueue: Java's PriorityQueue lets you treat elements like a VIP line. You define a priority order (importance), and the most important item gets processed first. Use PriorityQueue when elements need handling based on urgency.
- LinkedList: Java's LinkedList doubles as a queue, ideal for FIFO (First-In-First-Out) needs. It efficiently adds items to the back and removes them from the front. Choose LinkedList queues for both FIFO behavior and LinkedList's flexibility.
Choosing the Right Collection:
Selecting the appropriate collection type depends on several factors:
- Access patterns: Do you need random access by index (List), fast lookups by unique keys (Set), or retrieval based on key-value pairs (Map)?
- Ordering: Do you require elements to be maintained in a specific order (TreeSet, TreeMap, LinkedHashMap) or is the order irrelevant (ArrayList, HashSet, HashMap)?
- Duplicates: Do you need to allow duplicate elements (List, some Queue implementations) or enforce uniqueness (Set)?
- Performance: Consider the trade-offs between different implementations (e.g., faster average-case lookups in HashMap vs. sorted order in TreeMap).
Advanced JCF Tools:
Beyond the core collection types, the JCF offers advanced interfaces and implementations for specific needs. Let's explore these powerful tools:
- Deque: Adding and Removing from Both Ends (Double-ended Queue)
Java's Deque is like a double-sided queue. Add and remove items from both ends, unlike a regular queue (FIFO). Use Deque for flexible data access when order isn't critical. Common functionalities include addFirst(element) (similar to pushing onto a stack) and removeLast(element).
Common Implementation:
- LinkedList: This versatile implementation supports both Queue and Deque interfaces, allowing you to efficiently add/remove from either end.
-
Stacks: LIFO with Deque
While there's no dedicated Stack interface, you can simulate LIFO (Last-In-First-Out) behavior using a Deque. By utilizing methods like push(element) (equivalent to addFirst(element)) and pop() (equivalent to removeFirst()), you can create a stack functionality on top of a Deque implementation, typically using LinkedList.
-
Ordered Sets: SortedSet & NavigableSet
The SortedSet interface extends Set, ensuring elements are maintained in a specific order based on their natural ordering (if they implement Comparable) or a custom comparator. This allows for efficient retrieval based on order and range-based operations. Common functionalities include first() (retrieves smallest element) and last() (retrieves largest element).
Implementation:
- TreeSet: This implementation utilizes a sorted tree structure, guaranteeing elements are stored in ascending order. It provides efficient retrieval of elements in sorted order and operations like finding elements within a specific range.
- NavigableSet: Enhanced Navigation for Ordered Sets
The NavigableSet interface extends SortedSet and offers additional functionalities for navigating through the set based on order. It allows for more precise element retrieval and manipulation within the sorted structure. Common functionalities include ceiling(element) (returns the least element greater than or equal to a given element) and subSet(fromElement, toElement) (returns a view of the set within a specific range).
- Ordered Maps: SortedMap & NavigableMap
Similar to SortedSet and NavigableSet, the SortedMap interface extends Map, ensuring key-value pairs are maintained in a specific order based on the natural ordering of keys or a custom comparator. Common functionalities include firstKey() (retrieves the smallest key) and lastKey() (retrieves the largest key).
Implementation:
- TreeMap: This implementation utilizes a sorted tree structure, guaranteeing key-value pairs are stored in ascending order based on keys. It provides efficient retrieval of key-value pairs in sorted order and operations like finding entries within a specific key range.
- NavigableMap: Navigational Prowess for Ordered Maps
The NavigableMap interface extends SortedMap and offers additional functionalities for navigating through the map based on key order. It allows for more precise key-value pair retrieval and manipulation within the sorted structure. Functionalities mirror those of NavigableSet but operate on key-value pairs.
Choosing the Right Collection
Choosing the right JCF collection hinges on several factors. Here's a quick guide:
- Access Patterns: Do you need random access by index (Lists like ArrayList), fast lookups by unique keys (Sets like HashSet), or retrieval based on key-value pairs (Maps like HashMap)? Each collection is optimized for specific access patterns.
- Ordering: Is maintaining a specific order crucial (TreeSet, TreeMap, LinkedHashMap)? If not, unordered collections (ArrayList, HashSet, HashMap) might be better suited.
- Duplicates: Do you need to allow duplicate elements (Lists, some Queue implementations) or enforce uniqueness (Sets)?
Performance Considerations:
- Insertion/Deletion: Frequent insertions/deletions in the middle might favor LinkedList (Lists) over ArrayList.
- Searching: For fast lookups, consider HashSet (Sets) or HashMap (Maps) with their average-case efficiency.
- Sorting: If sorted retrieval is essential, opt for TreeSet (Sets) or TreeMap (Maps) despite their slower insertion/deletion times.
Remember: There are trade-offs between different implementations. Striking a balance between your specific needs and performance considerations leads to the optimal collection choice for your Java program.
Utility Classes and Methods in JCF
The JCF offers more than just core collections. Let's explore some helpful utility features:
- Collections Class: This static utility class provides various methods for manipulating collections. These include sorting elements (using natural ordering or custom comparators), searching for elements, and creating copies of collections.
- Comparator and Comparable Interfaces: For customized sorting behavior within ordered collections (TreeSet, TreeMap), you can define custom comparators that implement the Comparator interface. Alternatively, elements themselves can implement the Comparable interface, allowing them to specify their own sorting criteria.
- Iterators: The Iterator interface acts like a universal remote for your Java collections (lists, sets, etc.). It lets you use the same methods (like hasNext and next) to loop through elements, regardless of the collection type. This keeps your code cleaner and more flexible.
Generics: Bringing Type Safety to JCF Collections
Java Generics, introduced in Java 5, revolutionized collection handling. Here's how they elevate your JCF experience:
- Type Safety: Before generics, collections could hold any object type, leading to potential runtime errors. Generics allow you to specify the type of elements a collection can hold (e.g., List for a list of strings). This enhances code safety and improves readability by explicitly declaring the expected data type.
- Reduced Casting: Generics eliminate the need for frequent casts when working with collections. The compiler ensures type compatibility at compile time, preventing runtime exceptions caused by incorrect object types.
Generics in Action:
- Collection interfaces like List, Set, and Map are declared with type parameters. These parameters specify the allowed element types.
- Implementations like ArrayList or HashMap explicitly state the element types they hold.
Conclusion
The Java Collections Framework (JCF) supercharges your data handling in Java apps. It provides a range of collections, from familiar Lists and Sets to advanced options. Choosing the right one is key to clean, efficient Java code.
Master the JCF to: Organize data effectively, Find elements fast and Write cleaner code
With the JCF, you'll make informed decisions for smoother data handling and become a Java pro!
About the Author
Mr. Omar Khan
Qualification: Master's in Computer Science
Expertise: Java concurrency guru, optimizing applications for real-time responsiveness.
Research Focus: Explores advanced Java concurrency techniques to achieve peak performance in multi-threaded environments.
Experience: Crafts high-performance Java applications for critical systems, ensuring efficient handling of concurrent requests.
Mr. Khan's Java concurrency expertise builds responsive, scalable systems for real-time demands.