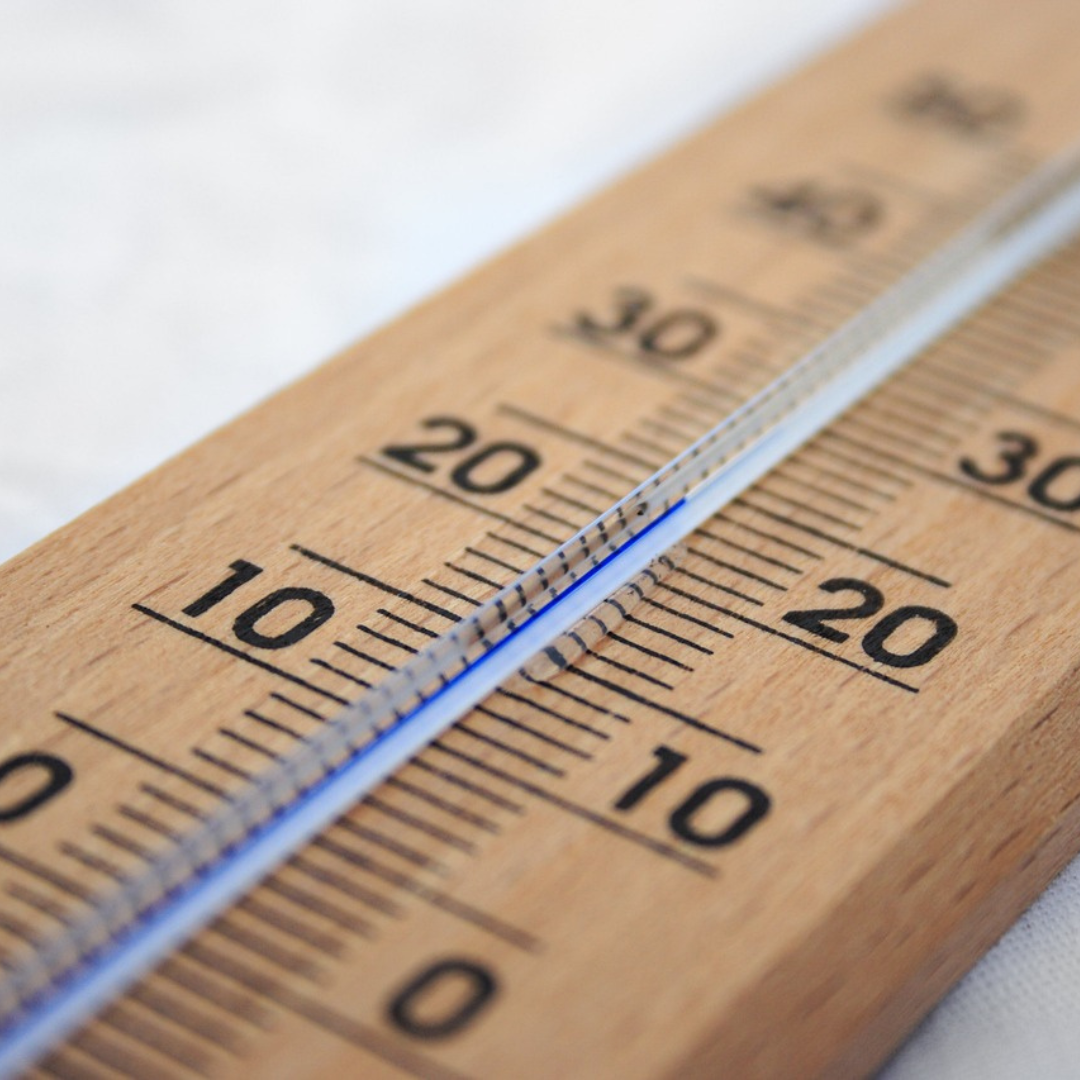
- 2nd Apr 2023
- 06:02 am
Programming Assignments for Beginners - C++ Homework Help
C++ Homework Question
C++ Temperature Conversion (C++ Functions) - Write a function named celsius that accepts a Fahrenheit temperature as an argument. The function should return the temperature, converted to Celsius. Demonstrate the function by calling it in a loop that displays a table of the Fahrenheit temperatures 0 through 20 and their Celsius equivalents. C = 5/9 * (F-32)
C++ Homework Solution
#include
using namespace std;
double celsius(double fahrenheit);
int main() {
// Display the table header
cout << "Fahrenheit\tCelsius\n";
cout << "--------------------------\n";
// Display the table of Fahrenheit temperatures and their Celsius equivalents
for (int fahrenheit = 0; fahrenheit <= 20; fahrenheit++) {
double c = celsius(fahrenheit);
cout << fahrenheit << "\t\t" << c << endl;
}
return 0;
}
// Convert Fahrenheit to Celsius
double celsius(double fahrenheit) {
double c = (5.0 / 9.0) * (fahrenheit - 32);
return c;
}
In this program, we define a function named celsius that accepts a Fahrenheit temperature as an argument and returns the temperature converted to Celsius. We use this function in a loop to display a table of Fahrenheit temperatures and their Celsius equivalents.
Note that we use the formula for converting Fahrenheit to Celsius, which is C = 5/9 * (F-32), where C is the Celsius temperature and F is the Fahrenheit temperature.