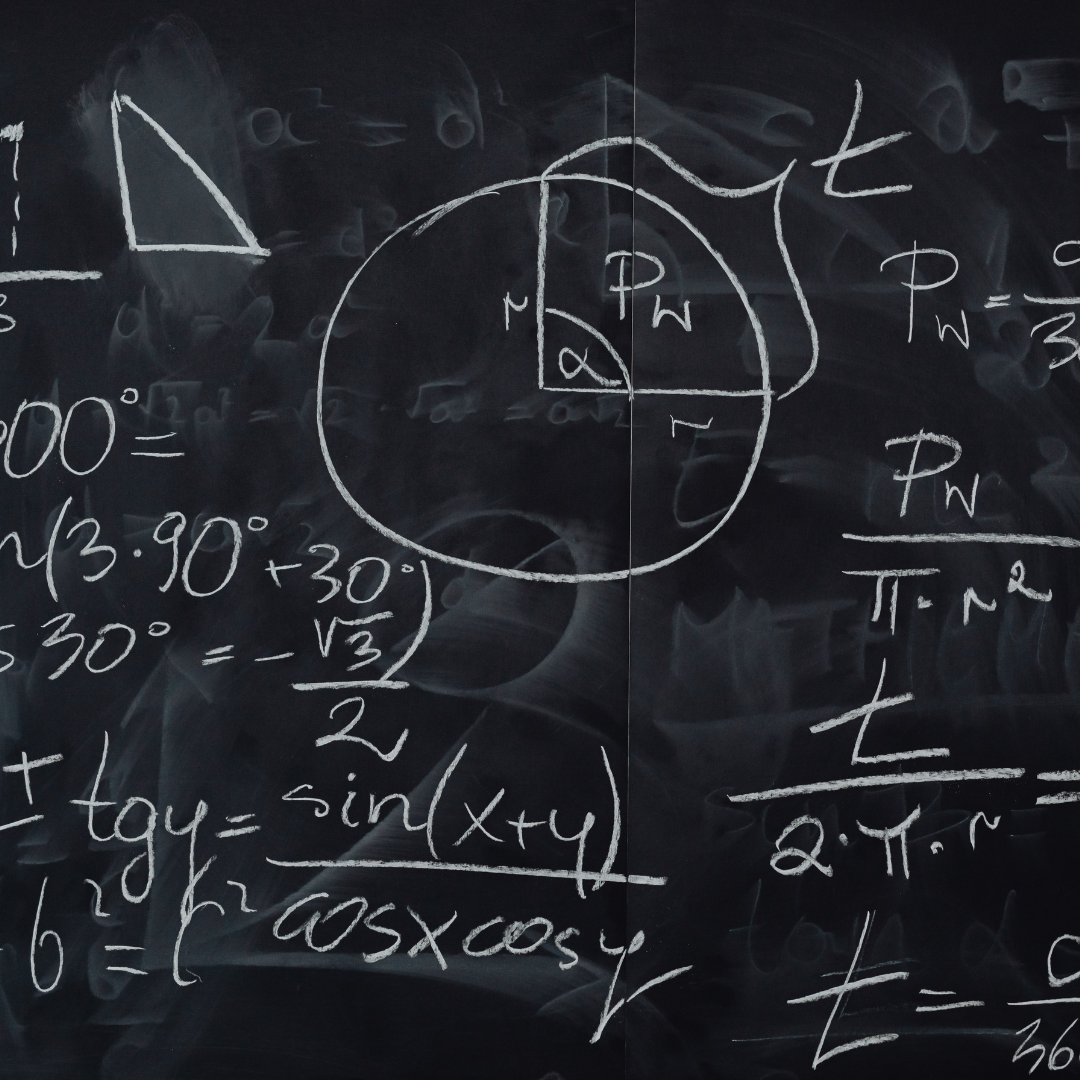
- 3rd Apr 2023
- 09:19 am
Java Homework Question
Sine, Cosine, Tan (using Math and Random classes) - Write an app that generates a random integer in the range of 20 to 40 inclusive, and displays the sine, cosine, and tangent of that number
Java Homework Solution
Here's the Java code for the app that generates a random integer in the range of 20 to 40 inclusive and displays the sine, cosine, and tangent of that number using the Math and Random classes:
import java.util.Random;
public class TrigFunctions {
public static void main(String[] args) {
Random random = new Random();
// Generate a random integer between 20 and 40 inclusive
int angle = random.nextInt(21) + 20;
// Calculate the sine, cosine, and tangent of the angle using the Math class
double sin = Math.sin(Math.toRadians(angle));
double cos = Math.cos(Math.toRadians(angle));
double tan = Math.tan(Math.toRadians(angle));
// Display the angle and its sine, cosine, and tangent
System.out.println("The angle is " + angle + " degrees");
System.out.printf("Sine: %.4f\n", sin);
System.out.printf("Cosine: %.4f\n", cos);
System.out.printf("Tangent: %.4f\n", tan);
}
}
When you run this code, the program generates a random integer between 20 and 40 inclusive using the Random class, calculates the sine, cosine, and tangent of that angle using the Math class, and displays them to four decimal places. For example:
The angle is 32 degrees
Sine: 0.5299
Cosine: 0.8480
Tangent: 0.6249
Note that we used the Math.toRadians() method to convert the angle from degrees to radians before calculating the sine, cosine, and tangent using the Math class.