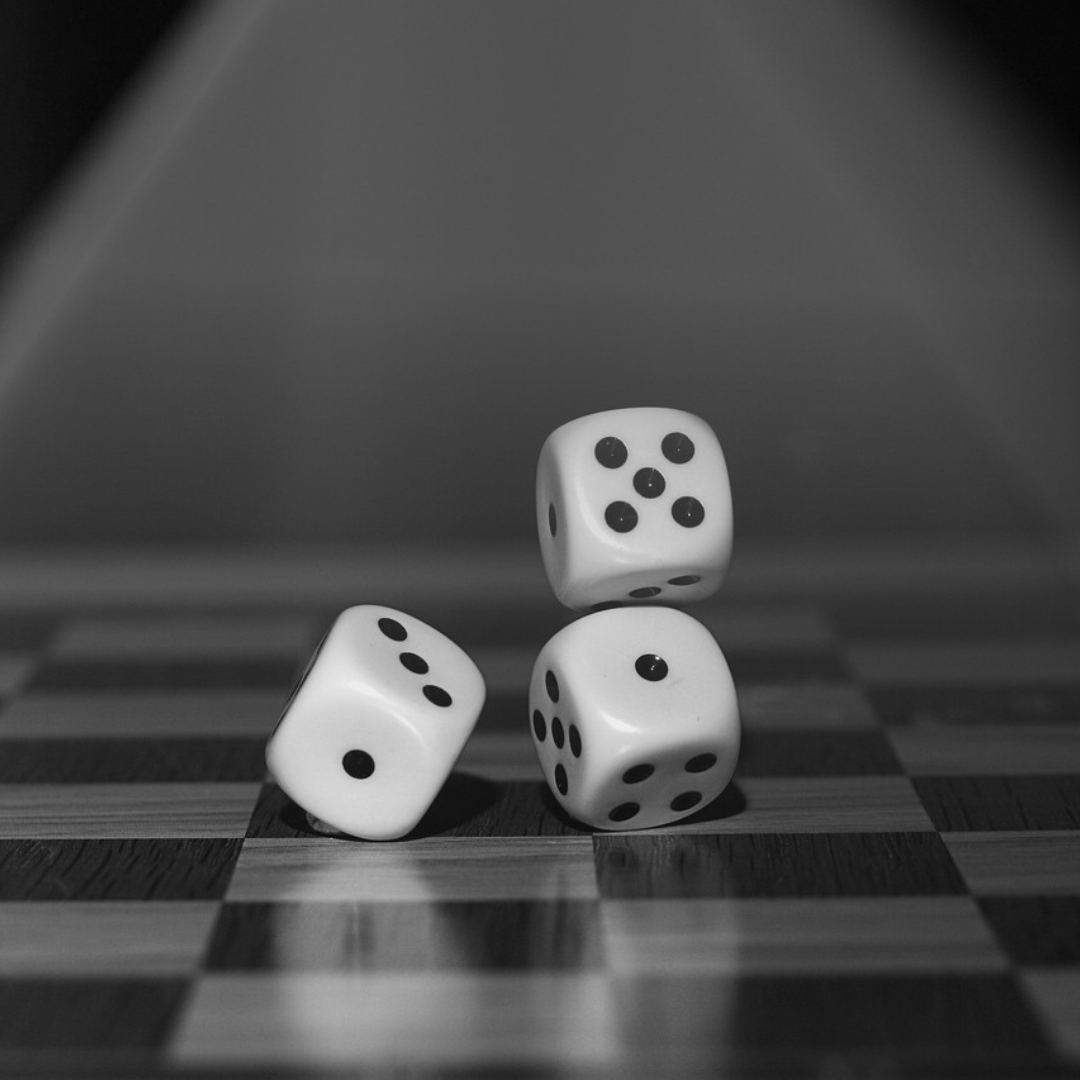
- 5th Apr 2023
- 09:33 am
- Admin
Python Homework Help
Python Homework Question
Python Logical Puzzles, Games, and Algorithms: Roll of Dice
Python Homework Solution
Here's a Python program that simulates rolling a pair of dice:
import random
def roll_dice():
"""Simulate rolling a pair of dice."""
die1 = random.randint(1, 6)
die2 = random.randint(1, 6)
total = die1 + die2
print("You rolled a", die1, "and a", die2, "for a total of", total)
return total
def main():
"""Main function to play the game."""
print("Let's roll some dice!")
total = roll_dice()
if total == 7 or total == 11:
print("You win!")
elif total == 2 or total == 3 or total == 12:
print("You lose!")
else:
print("Your point is", total)
while True:
new_total = roll_dice()
if new_total == total:
print("You win!")
break
elif new_total == 7:
print("You lose!")
break
if __name__ == '__main__':
main()
The roll_dice() function simulates rolling a pair of dice by generating a random number between 1 and 6 for each die, adding the results, and returning the total. The main() function starts the game, calls roll_dice() to roll the dice, and checks the total. If the total is 7 or 11, the player wins. If the total is 2, 3, or 12, the player loses. Otherwise, the game enters a loop where it continues to roll the dice until either the player wins by rolling the original total again or loses by rolling a 7.