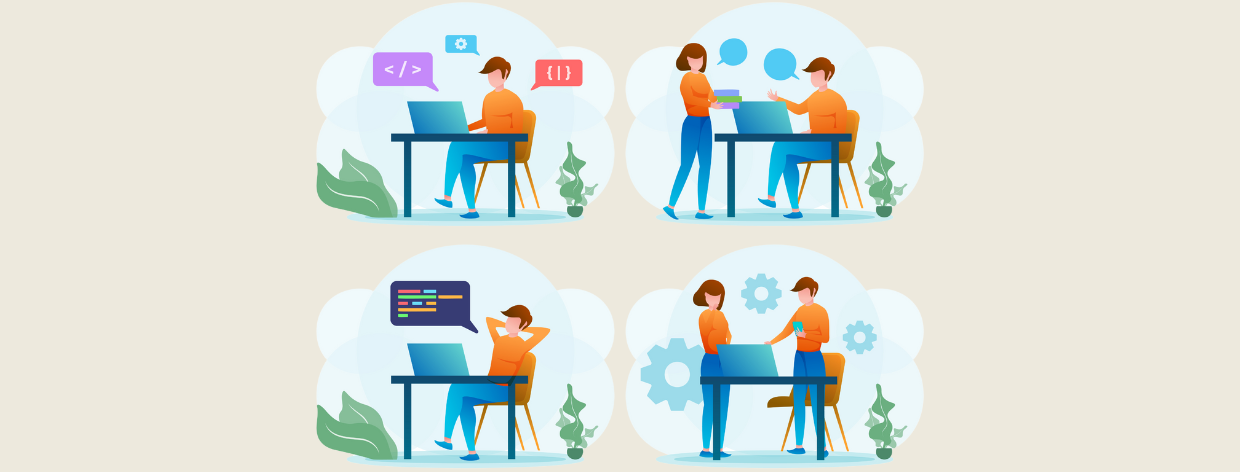
- 20th Feb 2024
- 15:24 pm
- Admin
Strings, the fundamental building blocks of text data, form the lifeblood of many Python applications. From parsing user input to building intelligent systems, adept string manipulation empowers you to unlock the hidden potential within textual information. Yet, manipulating strings often extends beyond simple character-by-character operations. The need to replace multiple characters simultaneously arises in various scenarios, demanding effective and efficient solutions.
Mastering multi-character replacements in Python strings opens doors to diverse applications across data analysis, machine learning, and more. If you're facing specific challenges related to Replace Multiple Characters in Strings, remember we are here with our Python Assignment Help and Python Homework Help service to support your learning journey.
The Significance of String Manipulation:
String manipulation forms the bedrock of numerous tasks in Python programming:
- Data preprocessing: Cleaning and preparing text data for analysis often involves replacing unwanted characters, handling whitespace inconsistencies, or ensuring specific formats.
- Data validation: Filtering out invalid entries or enforcing data integrity might necessitate the replacement of non-compliant characters.
- Text transformations: Tasks like anonymization, obfuscation, or stylistic formatting rely on strategic character replacements.
- Natural Language Processing (NLP): Stemming, lemmatization, and tokenization, crucial steps in NLP pipelines, heavily involve replacing characters based on linguistic rules.
The Challenge of Multiple Character Replacements:
While single-character replacements are often straightforward, replacing multiple occurrences simultaneously introduces complexities. Imagine trying to remove all punctuation from a sentence or standardize special characters across a large dataset. Manually iterating through each character becomes tedious and error-prone. We need more sophisticated approaches.
Methods to Master:
Python offers a diverse arsenal of methods to tackle this challenge:
- Built-in Functions: Leverage replace(), translate(), or regular expressions with re.sub() for basic to advanced replacements.
- Third-Party Libraries: Explore specialized libraries like pandas or numpy for efficient vectorized operations on large datasets.
- Custom Functions: Craft tailored solutions using lambda functions or recursive approaches for intricate replacement logic.
Understanding the strengths and weaknesses of each method is crucial. Built-in functions offer simplicity but might lack flexibility. Libraries provide power but might have specific use cases. Custom functions grant ultimate control but demand careful design.
Built-in String Methods:
One of the most important tasks in Python when manipulating strings is dealing with a bunch of characters. But no fear, because the Python built-in methods pretty much offer quite a diversified range of weapons for this task—all with their own different advantages and considerations. Let’s start with the five main methods and clarify their function.:
- replace():
The replace() method reigns supreme for basic replacements. It accepts two arguments: the substring to be replaced and the replacement string. Its simplicity makes it ideal for scenarios where you need to replace a specific character or short sequence uniformly throughout the string.
text = "Hello, w0rld!"
new_text = text.replace("o", "a")
print(new_text)
# Output: "Hella, w@rld!"
- translate():
The translate() method empowers you to define a custom translation table, a mapping between characters. It's not just single characters which get replaced but any character in the table can be taken and replaced in the string with its counterpart, hence gives immense flexibility for replacements.
translation_table = str.maketrans("aeiou", "12345")
text = "The quick brown fox jumps over the lazy dog"
new_text = text.translate(translation_table)
print(new_text)
# Output: "Th3 qu1ck br0wn f0x jumps 0v3r th3 l@zy d0g"
- maketrans():
While translate() relies on pre-defined tables, maketrans() allows you to craft your own. It accepts three arguments: the characters to be replaced, the corresponding replacements, and any characters remaining unchanged (defaulting to None). This fine-grained control enables intricate replacements based on your specific needs.
translation_table = str.maketrans("aeiou", "AEIOU", " ")
text = "Th3 qu1ck br0wn f0x jumps 0v3r th3 l@zy d0g"
new_text = text.translate(translation_table)
print(new_text)
# Output: "The quick brown fox jumps over the lazy dog"
- regex:
Regular expressions add another layer of sophistication to the game. The re.sub() function empowers you to define intricate patterns using regex syntax, and replace all occurrences within the string. However, regex mastery requires a deeper understanding, making it suitable for more advanced scenarios.
import re
text = "The quick brown fox jumps over the lazy dog"
new_text = re.sub(r"\d", "*", text)
print(new_text)
# Output: "The qu*ck br*wn f*x jumps *v*r th* l*zy d*g"
- split() + join():
For specific replacements, splitting the string into a list using split() and then joining it back with the replacements offers an alternative approach. While not as efficient for large-scale replacements, it can be intuitive for smaller strings or intricate transformations.
text = "The quick brown fox jumps over the lazy dog"
words = text.split(" ")
new_words = [word.replace("o", "a") for word in words]
new_text = " ".join(new_words)
print(new_text)
# Output: "The qu*ck br*wn f*x jumps *v*r th* l*zy d*g"
Remember, choosing the right method depends on your specific needs, complexity, and comfort level. Experiment with each approach and discover the most effective tool for your multi-character replacement endeavors in Python!
Third-Party Libraries
While Python's built-in methods offer versatility, specific use cases demand more specialized tools. Here's where third-party libraries shine:
- pandas:
For large datasets, pandas reigns supreme. Its vectorized operations excel at replacing characters across entire DataFrames efficiently. Imagine replacing special characters in thousands of product names using:
import pandas as pd
data = {"product_name": ["Prod*ct1", "Pr0duct2"]}
df = pd.DataFrame(data)
df["product_name"] = df["product_name"].str.replace("*", "").str.replace("0", "o")
print(df)
Advantages: Blazing speed for large datasets, convenient data manipulation within DataFrames.
Limitations: Not ideal for single-string operations, requires learning pandas fundamentals.
- numpy:
For strings primarily containing numbers, numpy's array manipulation prowess comes in handy. Imagine replacing numeric placeholders with actual values:
import numpy as np
text = "Th3 qu1ck br0wn f0x jumps 0v3r th3 l@zy d0g"
numbers = np.array([0, 1, 2, 3, 4, 5, 0])
new_text = np.char.replace(text, numbers.astype(str), "")
print(new_text)
Advantages: High performance for numerical replacements, leverages familiar numpy syntax.
Limitations: Not suitable for general character replacements, requires familiarity with numpy arrays.
Advanced Techniques
When basic built-in methods fall short, venturing into advanced techniques unlocks even more intricate and efficient multi-character replacements in Python. Let's explore some powerful tools:
- Lambda Functions:
Lambda functions, anonymous functions defined right within your code, offer dynamic replacements based on conditions. Consider replacing vowels only in uppercase words:
text = "The Quick Brown Fox Jumps Over the Lazy Dog"
new_text = text.translate(str.maketrans("", "", lambda c: c.upper() and c.isalpha() and c in "AEIOU" and c.replace("A", "a").replace("E", "e")))
print(new_text)
# Output: "Th3 Qu1ck Br0wn F0x Jumps Ov3r th3 L@zy D0g"
Advantages: Compact and flexible for conditional replacements within methods.
Limitations: It can become complex for intricate conditions, and readability might suffer.
- Recursive Functions:
For intricate, multi-step replacements, recursive functions come into play. Imagine removing all nested parenthesis, iteratively replacing inner pairs first:
def remove_nested_parens(text):
if "(" not in text or ")" not in text:
return text
new_text = text.replace("()", "")
return remove_nested_parens(new_text)
text = "(This is a (test) string) with (nested) parenthesis"
new_text = remove_nested_parens(text)
print(new_text)
# Output: "This is a test string with nested parenthesis"
Advantages: Powerful for complex multi-step replacements, modular and reusable.
Limitations: Debugging recursive functions can be challenging, with potential stack overflow issues for excessively deep nesting.
- Advanced Regular Expressions:
Regular expressions unlock true power for complex pattern matching and replacement. Imagine removing all HTML tags with their content, and preserving surrounding spaces:
import re
text = "
This is a paragraph with HTML.
"
new_text = re.sub(r"<[^>]+>(.*?)]+>", r"\1", text)
print(new_text)
# Output: "This is a paragraph with HTML."
Advantages: Highly expressive for intricate patterns, versatile for diverse replacement scenarios.
Limitations: Learning curve can be steep, and prone to errors with complex patterns.
Applications of Replacing Multiple Characters in Strings in Python
Mastering multi-character replacements in Python empowers you to tackle diverse real-world challenges across various domains. Here are some compelling examples:
Data Cleaning and Preprocessing:
- Text normalization: Standardize text data by replacing special characters, accents, or symbols with their canonical versions for consistent analysis.
- Handling whitespace inconsistency: Clean text data by replacing inconsistent whitespace characters to ensure uniform formatting.
- Removing unwanted characters: Cleanse text data by eliminating specific characters (punctuation, emojis, control characters) that might impact analysis or visualization.
Data Validation and Filtering:
- Enforcing specific formats: Ensure data adheres to predefined formats by replacing non-compliant characters (e.g., replacing non-numeric characters in phone numbers with spaces).
- Identifying and handling invalid data: Filter out problematic data entries by replacing specific patterns with markers for further investigation (e.g., replacing characters in invalid product codes).
- Preparing data for specific tasks: Tailor data representations for algorithms or tools by replacing characters based on their requirements (e.g., converting all uppercase letters to lowercase for case-insensitive search).
Text Processing and Manipulation:
- Anonymizing text data: Protect sensitive information by replacing relevant characters with placeholders or anonymization techniques.
- Text obfuscation: Scramble sensitive data for temporary storage or transmission by replacing characters with randomized alternatives.
- Creating stylized text: Craft visually appealing text for presentations or dashboards by replacing characters with symbols or alternative glyphs.
Natural Language Processing (NLP):
- Tokenization: Text data are appropriately cleaned for subsequent processing with NLP, where non-alphanumeric characters are replaced with delimiters of word or token splits.
- Stemming and lemmatization: Normalizing words to its base form by replacing suffix or prefix based on rules of language.
- Language-specific character conversion: Handle multilingual data by replacing characters specific to one language with their equivalents in another.