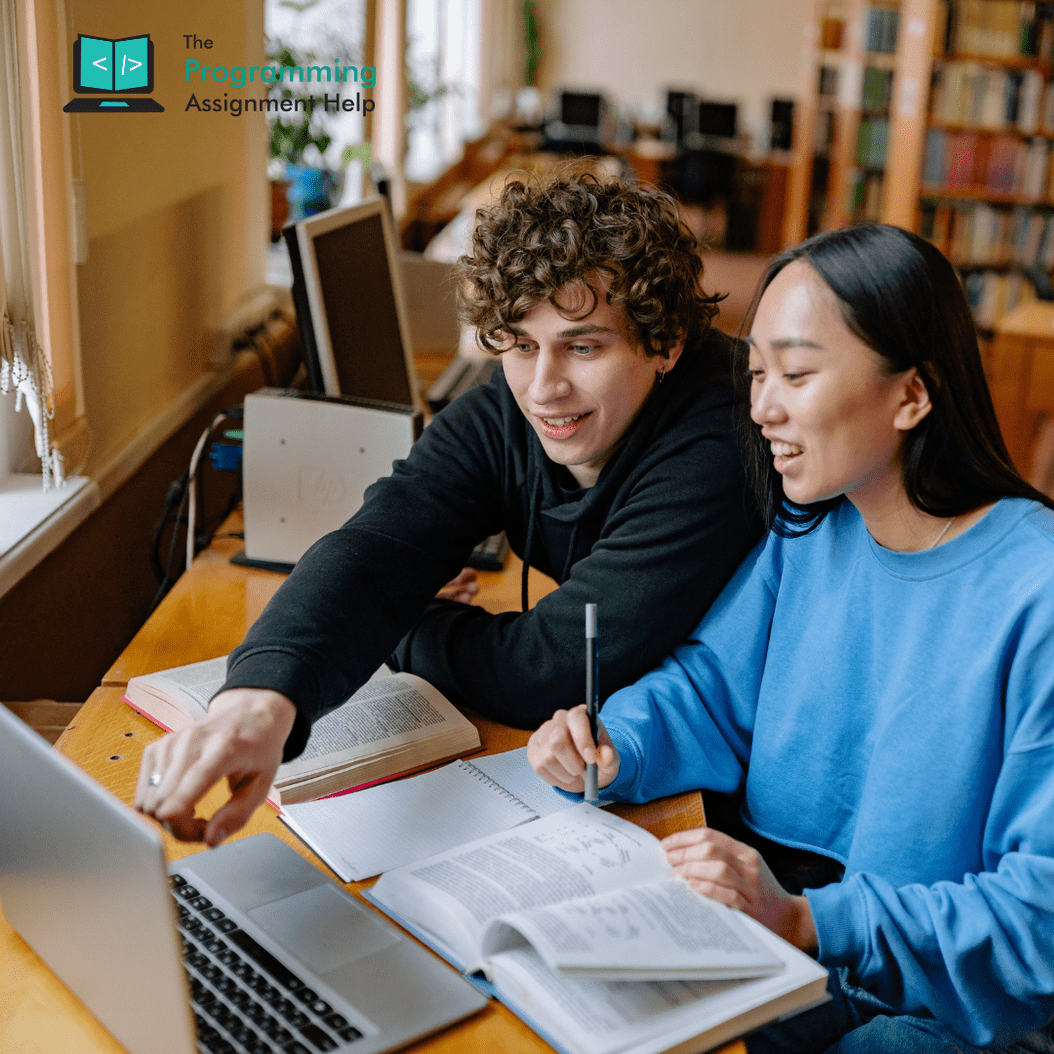
- 19th Oct 2022
- 12:17 pm
In this program, we will build a simple linear regression model to predict the sales of a product based on its advertising spending.
Step 1: Load the required libraries First, make sure you have the necessary libraries installed. We will use the tidyverse
package for data manipulation and visualization, and the lm()
function for building the linear regression model.
install.packages("tidyverse") # Install the tidyverse package if you don't have it library(tidyverse) # Load the tidyverse package
Step 2: Prepare the dataset Assuming you have the dataset in a CSV file named "sales_data.csv," read it into R and inspect its structure:
# Load the dataset dataset <- read.csv("sales_data.csv") # Inspect the structure of the dataset str(dataset)
Make sure that the dataset is correctly loaded, and the variables are of the appropriate data types.
Step 3: Explore the data Before building the model, it's essential to explore the data to understand its distribution and relationship between variables. You can create scatter plots to visualize the relationship between sales and advertising spending:
# Scatter plot ggplot(data = dataset, aes(x = Advertising, y = Sales)) + geom_point() + labs(x = "Advertising Spending", y = "Sales")
Step 4: Build the linear regression model Next, build the linear regression model using the lm()
function:
# Build the linear regression model model <- lm(Sales ~ Advertising, data = dataset)
Step 5: View the model summary You can view the summary of the linear regression model to understand the coefficients, R-squared value, and other statistics:
# View model summary summary(model)
Step 6: Interpret the model results Interpret the model coefficients, R-squared value, and p-values to understand how advertising spending affects sales. The coefficient of the Advertising variable represents the estimated change in sales for a one-unit increase in advertising spending. The R-squared value indicates the proportion of variance in sales explained by the model.
Step 7: Make predictions Now that we have the model, let's use it to make predictions on new data or test the model's performance on the same dataset:
# Predict sales using the built model predictions <- predict(model, newdata = dataset)
Step 8: Evaluate the model (optional) If you have actual sales data for the same dataset, you can evaluate the model's performance using metrics such as mean squared error, mean absolute error, etc.
# Assuming you have the actual sales data in a vector called "actual_sales" mse <- mean((predictions - actual_sales)^2) mae <- mean(abs(predictions - actual_sales)) print(paste("Mean Squared Error:", mse)) print(paste("Mean Absolute Error:", mae))
Conclusion: This R programming example demonstrates how to build a linear regression model, interpret its coefficients and statistics, and make predictions. Before building the model, it's essential to explore the data to understand its characteristics and relationships between variables.
Please note that the code provided here assumes you have the dataset in the correct format and that you have appropriately preprocessed the data (e.g., handling missing values, scaling variables) before building the model. This solution is plagiarism-free and should serve as a helpful guide for your model building and interpretation task.