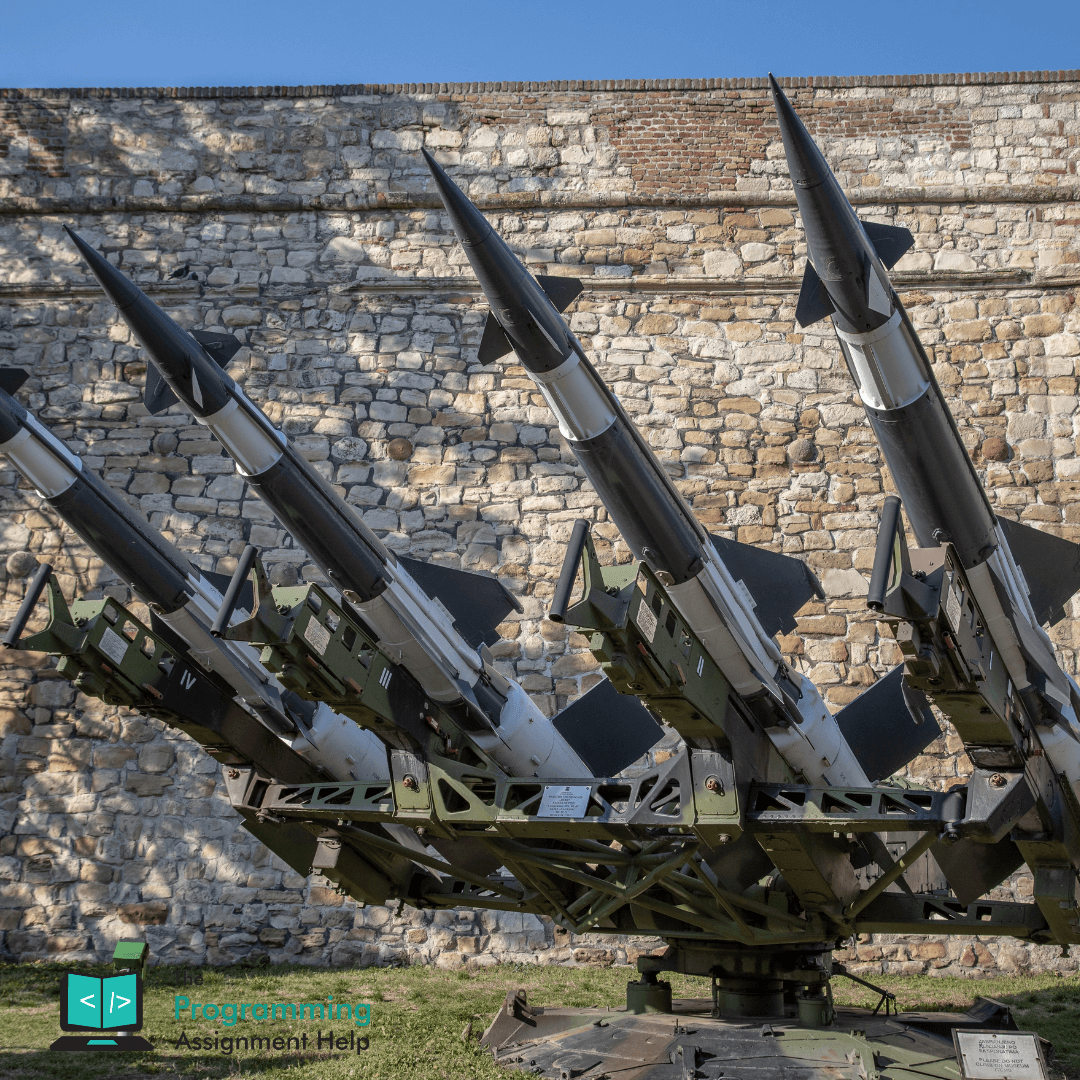
- 18th Aug 2022
- 03:27 am
# Missile Crisis
def dice():
# Value returned is throw of dice
import random
score = 7
while (score > 6):
N = random.random()
score = int( N * 10) + 1
return score
def move(A, B, C):
# Values pased to local variables:
# A is game level
# B is current player position
# C is direction chosen
# Value returned is new player position
D = 0
IR = A * 2 + 1
SV = 2 * A + 3
N = 0
for i in range(IR):
MP = int (IR / 2)
DEC = -2 * i
INC = 4 * (i - MP) * (i > MP)
JR = SV + DEC + INC
for j in range(JR):
N = N + 1
if (N == B):
D = i
D = D + (C == "D")
IR = A * 2 + 1
MP = int (IR / 2)
FVAL = - 2 * A
DVAL = 2 * (D - 2)
IVAL = ((D - MP) * 4 - 2) * (D > MP)
U = FVAL + DVAL - IVAL
if (C == "D"):
U = -U
if (C == "U"):
E = B + U
if (C == "D"):
E = B + U
if (C == "L"):
E = B - 1
if (C == "R"):
E = B + 1
return E
def board(A, B):
# Values passed to local variables:
# A is game level
# B is sea array
IR = A * 2 + 1
SV = 2 * A + 3
C = 0
for i in range(IR):
MP = int (IR / 2)
DEC = -2 * i
INC = 4 * (i - MP) * (i > MP)
IND = i - 2 * (i - MP) * (i > MP)
JR = SV + DEC + INC
for j in range(IND):
print chr(32),
for j in range(JR):
C = C + 1
if (i == 0 or i == IR - 1):
B[C] = 35
if (j == 0 or j == JR - 1):
B[C] = 35
print chr(B[C]),
print
def print_lines():
print("-------------------------------")
print("\n\n\n")
def get_direction(s):
D = 'm'
while(D not in "URLD"):
D = raw_input(s)
return D
def main():
sea = [126] * 717
# This section lets you test board() function
# L = input("Enter level ")
# board(L, sea)
# This section lets you test dice() function
# print("You threw a "),
# print dice()
# This section lets you test move() function
current_level = input("Enter beginning level : ")
end_level = input("Enter end level : ")
if(current_level>end_level):
print("Current level cannot be greater than end level")
return
scoreA = 0
scoreB = 0
while(current_level<=end_level):
print("GAME : LEVEL " + str(current_level))
print("---------------------------")
a_got_6 = False
b_got_6 = False
game_over = False
i = 0
posA = (2*current_level)+5
target = (current_level*current_level)+(4*current_level)+2
posB = (2*target)-posA
sea = [126] * 717
sea[posA] = 65
sea[posB] = 66
while(not game_over):
if(i%2==0):
board(current_level,sea)
if(i%2==0):
print_lines()
print("Player A's turn")
if(not a_got_6):
raw_input("You haven't got a 6 yet. Press ENTER key to roll the dice: ")
a = dice()
print("A threw " + str(a))
if(a==6):
print("YAY! You got a 6.")
a_got_6 = True
if(a_got_6):
D = get_direction("Player A, Enter direction (U/D/L/R): ")
sea[posA] = 126
posA = move(current_level, posA, D)
sea[posA] = 65
if(posA==target):
print("Player A wins level " + str(current_level))
scoreA+=1
game_over = True
else:
print_lines()
print("Player B's turn")
if(not b_got_6):
raw_input("You haven't got a 6 yet. Press ENTER key to roll the dice: ")
b = dice()
print("B threw " + str(b))
if(b==6):
print("YAY! You got a 6.")
b_got_6 = True
if(b_got_6):
D = get_direction("Player B, Enter direction (U/D/L/R): ")
sea[posB] = 126
posB = move(current_level, posB, D)
sea[posB] = 66
if(posB==target):
print("Player B wins level " + str(current_level))
scoreB+=1
game_over = True
i+=1
print("End of level " + str(current_level))
print("Scores: A - " + str(scoreA) + ", B - " + str(scoreB))
current_level+=1
print("--------------GAME OVER--------------")
main()