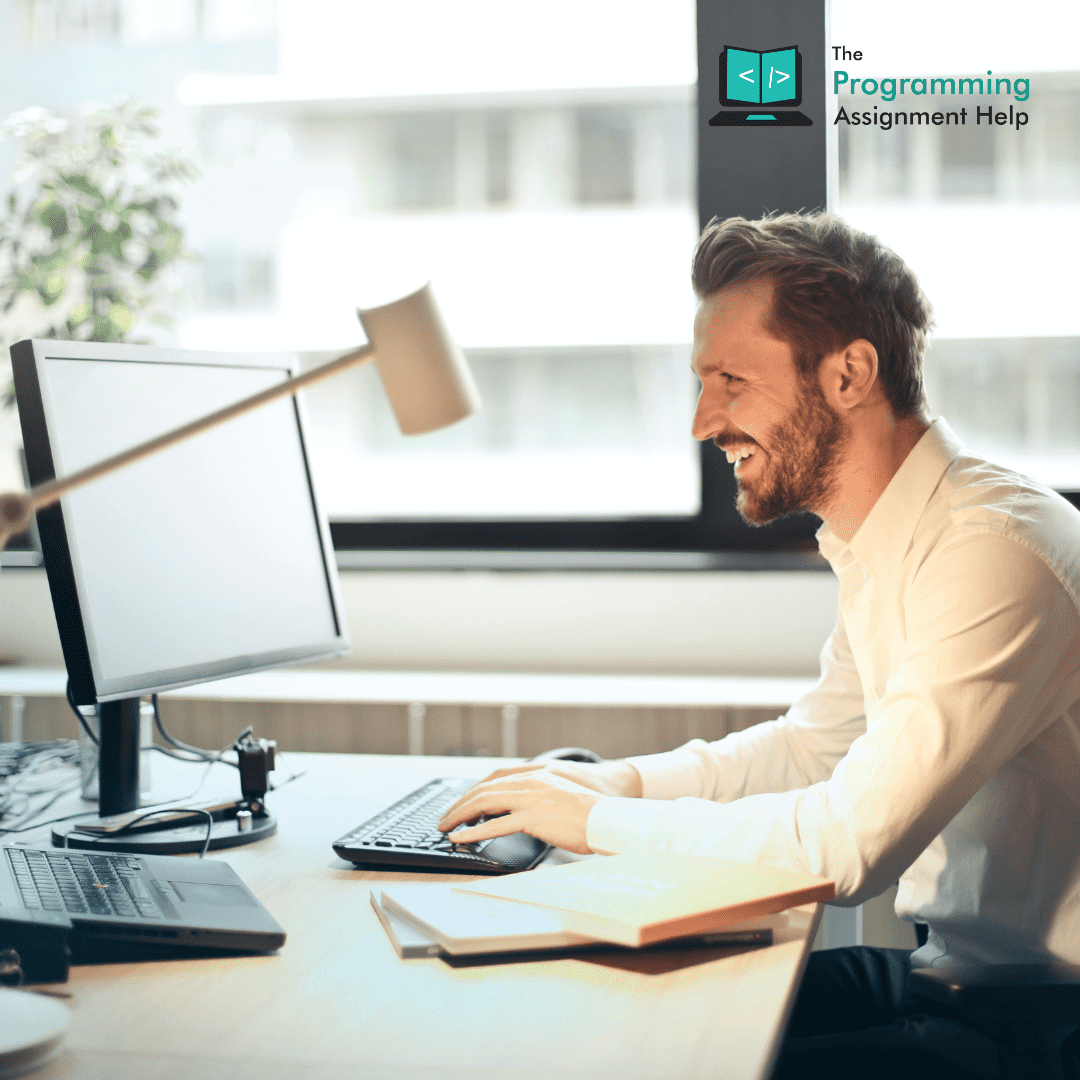
- 19th Oct 2022
- 12:01 pm
- Admin
#!/usr/bin/python3
# for windows the Shebang line is <#! python3>
# Full name:
# Student ID number
class Member:
NORMAL_FEE = '$' + str(80)
GOLD_FEE = '$' + str(100)
PLATINUM_FEE = '$' + str(150)
def __init__(self, fname, lname, phone, status):
self.fname = fname
self.lname = lname
self.phone = phone
if status == 0:
self.status = 'Normal'
self.fee = self.NORMAL_FEE
if status == 1:
self.status = 'Gold'
self.fee = self.GOLD_FEE
if status == 2:
self.status = 'Platinum'
self.fee = self.PLATINUM_FEE
def member_detail(self):
return f'First Name: {self.fname} {self.lname} \nPhone {self.phone} \nMember Status: {self.status} Fee: {self.fee}\n'
def update_member_status(self, status):
if status == 0:
self.status = 'Normal'
self.fee = self.NORMAL_FEE
if status == 1:
self.status = 'Gold'
self.fee = self.GOLD_FEE
if status == 2:
self.status = 'Platinum'
self.fee = self.PLATINUM_FEE
@classmethod
def from_string(cls, member_str):
fname, lname, phone, status = member_str.split('-')
return cls(fname, lname, phone, int(status))
@classmethod
def update_free_amt(cls, status, fee_amount):
if status == 0:
cls.NORMAL_FEE = '$' + str(fee_amount)
if status == 1:
cls.GOLD_FEE = '$' + str(fee_amount)
if status == 2:
cls.PLATINUM_FEE = '$' + str(fee_amount)
@staticmethod
def is_class_free(status):
if status == "Normal":
print("Class isn't free for normal members")
if status == "Gold":
print("Class isn't free for gold members")
if status == "Platinum":
print("Class is free for platinum members")