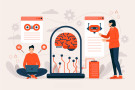
- 15th Nov 2023
- 22:32 pm
- Admin
In Java, memory allocation plays a vital role in its memory management system. Java abstracts this process, which removes the need for developers to handle low-level memory allocation and deallocation tasks. This simplifies programming by automating memory handling, sparing developers from managing memory at a granular level. Java instead employs a hybrid of automated memory allocation and garbage collection.
Memory allocation in Java begins with the creation of objects. When you use the 'new' keyword, memory on the heap is allocated to hold the object's data and methods. The Java Virtual Machine (JVM) is in charge of this allocation, ensuring that objects are generated effectively and memory is handled appropriately.
Java's automated memory management mechanism, which includes garbage collection, is one of its strengths. Garbage collection detects and reclaims memory that is no longer being used, hence preventing memory leaks. This technique contributes to the memory efficiency and general health of Java programs.
Java is a powerful and user-friendly language for constructing a wide range of applications, from web services to mobile apps, since developers can focus on writing code without worrying about memory allocation and deallocation.
Structure of Memory in Java and Memory Management
Memory management is an essential aspect within Java, ensuring efficient memory usage while preventing memory leaks. Java Virtual Machine (JVM) plays a critical role in this process. It organizes memory into specialized zones and uses generational garbage collection to optimize memory allocation and deallocation. This approach allows Java applications to effectively utilize memory resources, enhancing their performance and reliability. The JVM allocates memory in the following manner:
- Extreme Memory: This is where objects are allocated and released. The heap is separated into two sections: the Young Generation and the Tenured Generation.
- Young Generation: In the Young Generation, new things are originally assigned. It is divided into three sections: the Eden space and two survivor spaces (S0 and S1). things are initially assigned to the Eden space, and surviving things are relocated across survivor spaces after each trash collection cycle.
- Old Generation (Tenured Generation): Objects in the Young Generation that survive many trash collection cycles are promoted to the Old Generation. This area holds things that have a lengthy lifespan and is collected less regularly.
- Permanent Generation: In previous Java versions (up to Java 7), the Permanent Generation was in charge of keeping class information, interned strings, and other JVM-related data. Permanent Generation was superseded in Java 8 and later by Metaspace, which manages class metadata dynamically.
- Metaspace: Metaspace is used to hold class metadata in Java 8 and beyond, and it dynamically modifies its size as needed. This modification was introduced to alleviate difficulties with class loading and unloading.
In Java, the garbage collector is a vital mechanism that reclaims memory from objects no longer referenced by the program. The Java Virtual Machine (JVM) oversees memory allocation, deallocation, and garbage collection execution.
Automatic memory management and garbage collection in Java significantly minimize memory-related risks, like memory leaks. This feature enhances Java's safety and user-friendliness, making it more reliable and convenient for developers. Developers may concentrate on developing code while the JVM handles memory management.
How Memory is Allocated in Java
The Java Virtual Machine (JVM) manages memory allocation as a dynamic and automated process. The JVM is in charge of allocating memory when objects are generated, as well as ensuring that memory is appropriately managed to avoid concerns such as memory leaks. Here's how memory is allocated in Java:
- Object Design: When you use the 'new' keyword to create an object in Java, the JVM allocates heap memory to hold the object's data and methods. The heap is a section of memory dedicated to runtime data.
- Heap Memory: In Java, memory is mostly allocated on the heap. To handle items with various lives, the heap is separated into generations, including the Young Generation and the Old Generation (Tenured Generation).
- Young Generation: In the Young Generation, new things are originally assigned. The Eden space and two survivor spaces (S0 and S1) further segregate the Young Generation. Objects are produced in the Eden space initially.
- Generational Waste Collection: To optimize memory management, Java implements generational garbage collection. Most artifacts have a limited lifespan, and generational collection capitalizes on this reality. Objects that survive the Young Generation's first collecting are promoted to the Old Generation.
- Automated Garbage Collection: Garbage collection is performed automatically by the JVM to discover and reclaim memory held by things that are no longer referenced or are unreachable. The JVM finds and frees memory from objects that have no active references during garbage collection.
- Metaspace (Java 8+): Class metadata, such as class definitions and method information, is stored in Metaspace in Java 8 and subsequent versions. Metaspace controls its size dynamically to avoid problems with class loading and unloading.
- Manual Memory Management (Uncommon): While it is generally advised, developers can directly request garbage collection by calling 'System.gc()'. Manual memory management is usually reserved for extreme circumstances.
- Handling OutOfMemoryErrors: When the JVM concludes that it does not have enough memory to allocate for new objects, it raises a 'OutOfMemoryError'. Proper memory management procedures, such as controlling references and clearing away unwanted objects, aid in the prevention of such problems.
Java memory allocation is intended to be automated and developer-friendly, lowering the chance of memory-related issues such as memory leaks. The garbage collector in the JVM guarantees that memory is managed and reclaimed quickly, making Java a safer and more efficient language for application development.
How Memory is Deallocated in Java
Memory deallocation in Java is mostly the job of the Java Virtual Machine (JVM), which manages the process of freeing up memory held by no-longer-in-use objects automatically. Here's how Java handles memory deallocation:
- Collection of Garbage: A garbage collector is used by the JVM to identify and recover memory used by items that are no longer accessible or in use. Garbage collection is an automatic operation that operates in the background on a regular basis.
- Collection of Generational Garbage: To optimize memory management, Java implements generational garbage collection. Objects are classified into two generations: the Young Generation and the Old Generation.
- Generation Y: In the Young Generation, new things are originally assigned. This is where objects with limited lifespans tend to live. Garbage collection is regular and efficient in the Young Generation.
- Old Generation (Tenured Generation): Objects in the Young Generation that survive many trash collection cycles are promoted to the Old Generation. The Old Generation comprises artifacts that have a lengthy lifespan and is gathered less regularly.
- Algorithm of Mark and Sweep: Garbage collection is usually done in two steps. The JVM first identifies items that are still accessible or in use. Then it goes across memory, clearing any items that were not tagged as accessible. Unmarked items are treated as garbage and are deallocated.
- Finishing: Some objects may contain a 'finalize()' function that may be used to execute cleaning activities prior to deallocating the object. The usage of 'finalize()', on the other hand, is discouraged, and the ideal practice is to explicitly release resources when they are no longer required.
- Automatic Memory Management: Memory management in Java is mostly automated. Developers do not need to deallocate memory directly or worry about memory leaks. The JVM manages memory deallocation by finding and freeing memory from no longer-in-use objects.
- Manual Memory Management (Uncommon): In certain instances, developers can use 'System.gc()' to request garbage collection. Manual memory management, on the other hand, is often discouraged because the JVM is built to handle memory deallocation automatically.
Memory deallocation is an important component of Java's architecture, with the goal of simplifying the process for developers and lowering the likelihood of memory-related issues such as memory leaks. The garbage collector in the JVM is in charge of automatically finding and clearing away unneeded objects, making Java a more secure and efficient language for application development.