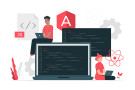
- 29th Nov 2023
- 15:18 pm
- Admin
JavaScript, a versatile language, excels in managing exceptions and organizing code through modules. Exception handling involves structured use of try, catch, and finally blocks, while modules, introduced in ES6, allow for clean and efficient code organization with import and export statements. Let's explore the intricacies of JavaScript Exceptions and Modules for robust and maintainable code.
JavaScript Exceptions
JavaScript exceptions are unforeseen issues that disrupt the normal flow of code execution. These can range from runtime errors to logical errors and can be caused by various factors like invalid user input, unexpected server responses, or issues with external dependencies.
Proper exception handling is crucial for identifying, managing, and gracefully recovering from these issues, ensuring the stability and reliability of JavaScript applications. It involves anticipating potential errors, detecting them when they occur, and implementing strategies to handle and, if possible, resolve them without compromising the entire application.
JavaScript Exception Handling
JavaScript exception handling involves the use of try, catch, and finally blocks. The try block contains the code that might generate an exception, while the catch block catches and handles the exception. The finally block, if present, executes regardless of whether an exception is thrown or caught. This approach enables developers to implement effective error-handling strategies, ensuring application stability and enhancing user experience.
Let's explore an example:
try {
// Code that might throw an exception
let result = someFunction();
console.log(result);
} catch (error) {
// Handling the exception
console.error(`An error occurred: ${error.message}`);
} finally {
// Code to execute regardless of an exception
cleanupResources();
}
Common JavaScript Exceptions
In the dynamic world of JavaScript, encountering exceptions is inevitable. Common JavaScript exceptions, such as SyntaxError, TypeError, and ReferenceError, act as signals that something unexpected has happened during code execution. Understanding these exceptions is crucial for developers to diagnose issues, enhance code quality, and create more resilient applications.
The three common ones are:
- SyntaxError: ‘SyntaxError’ occurs when the JavaScript engine encounters code with a syntax that deviates from the language's grammar rules, preventing proper interpretation and execution.
let x = 10;
console.log(x; // SyntaxError: Unexpected token ';'
- ReferenceError: The `ReferenceError` occurs when the code attempts to access an undeclared variable or references a non-existent function or object during JavaScript execution. This results in a runtime failure in the program.
console.log(y); // ReferenceError: y is not defined
- TypeError: The `TypeError` is triggered when an operation is attempted on an inappropriate data type in JavaScript. It indicates a mismatch in the expected and actual types during runtime in JavaScript programming.
let z = null;
z.toUpperCase(); // TypeError: Cannot read property 'toUpperCase' of null
Understanding these exceptions aids in writing more robust and error-tolerant code.
Custom Exception Handling
Custom exceptions in JavaScript offer developers the flexibility to address specific scenarios unique to their applications. The ability to create custom exceptions enhances the precision of error handling, allowing for more tailored responses to diverse situations.
class CustomError extends Error {
constructor(message) {
super(message);
this.name = 'CustomError';
}
}
// Using the custom exception
try {
throw new CustomError('This is a custom exception');
} catch (error) {
console.error(`${error.name}: ${error.message}`);
}
JavaScript Modules
JavaScript modules are a fundamental part of modern web development, offering a modular and organized approach to code structuring. Modules allow developers to encapsulate functionalities into separate files, promoting better maintainability, reusability, and overall code organization. This modularization facilitates collaboration among developers, as different parts of the application can be worked on independently and seamlessly integrated, enhancing the overall efficiency and scalability of JavaScript projects.
ES6 Modules
ES6 (ECMAScript 2015) introduced native support for modules in JavaScript, providing a standardized way to organize code into reusable and independent units. ES6 modules offer a clean syntax for importing and exporting functionalities between files, promoting a more structured and scalable development approach. This has significantly improved code organization and collaboration in modern JavaScript projects.
Here's a simple example:
- math.js
// math.js module
const add = (a, b) => a + b;
const subtract = (a, b) => a - b;
module.exports = { add, subtract };
- app.js
// app.js module importing math.js
import { add, subtract } from './math.js';
console.log(add(5, 3)); // Output: 8
console.log(subtract(8, 3)); // Output: 5
ES6 modules provide a clean and efficient way to structure code and manage dependencies.
CommonJS Modules
CommonJS is a module system that predates ES6 modules and gained popularity in server-side JavaScript with Node.js. It follows a synchronous approach for loading modules, making it suitable for server environments. CommonJS modules utilize the require function for importing modules and module.exports for exporting functionalities. While still widely used, ES6 modules have become the modern standard for client-side and cross-environment JavaScript development.
- math.js
// math.js module
const add = (a, b) => a + b;
const subtract = (a, b) => a - b;
module.exports = { add, subtract };
- app.js
// app.js module importing math.js
const { add, subtract } = require('./math.js');
console.log(add(5, 3)); // Output: 8
console.log(subtract(8, 3)); // Output: 5
Dynamic Imports
Dynamic imports in JavaScript, introduced in ES6, allow modules to be loaded on-demand during runtime. The import() function returns a Promise, enabling asynchronous loading of modules. This is particularly beneficial for scenarios where modules need to be loaded conditionally or based on user interactions. Dynamic imports enhance performance by reducing the initial load time and optimizing resource usage.
// Dynamic import
const mathModule = await import('./math.js');
console.log(mathModule.add(5, 3)); // Output: 8