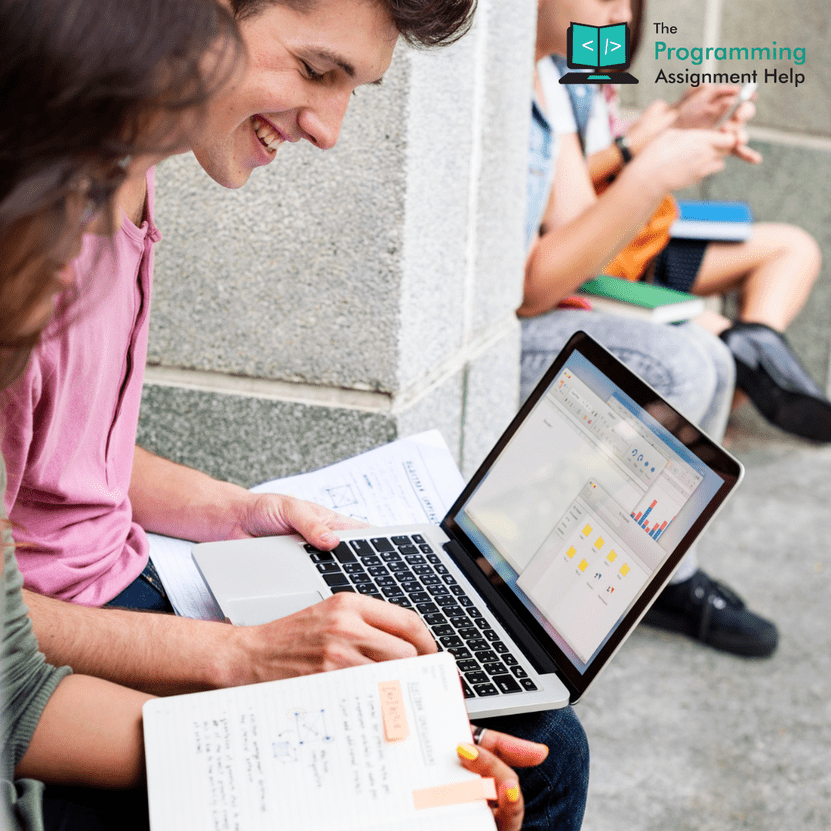
- 26th Jan 2023
- 04:12 am
- Admin
Java is a widely used programming language that is suitable for implementing cryptographic algorithms such as the Diffie-Hellman key exchange method. The Java Cryptography Extension (JCE) provides a set of cryptographic algorithms including the Diffie-Hellman key agreement algorithm which makes it easy to implement secure key exchange in Java-based applications. Additionally, Java's platform independence makes it suitable for cross-platform development. It is a valuable tool for secure communication between parties.
Java Assignment Help - Java Assignment Solution
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class Main {
private static long PRIME_SIZE_LIMIT = 10000000;
private static Random random = new Random();
public static void main(String[] args) {
List safePrimes = generateSafePrimes(PRIME_SIZE_LIMIT);
long p = safePrimes.get(random.nextInt(safePrimes.size()/2) + safePrimes.size()/2);
long q = 2*p + 1;
long g = generateGenerator(p);
// Alice keys
long a = generatePrivateKey(q);
long y_A = generatePublicKey(g, p, a);
// Bobs keys
long b = generatePrivateKey(q);
long y_B = generatePublicKey(g, p, b);
System.out.println("Alice private key: " + a);
System.out.println("Alice public key: " + y_A);
System.out.println("Alice will calculate the Diffie-Hellman key as follows: y_B^a " + exp(y_A, p, b));
System.out.println("Bob private key: " + b);
System.out.println("Bob public key: " + y_B);
System.out.println("Bob will calculate the Diffie-Hellman key as follows: y_A^b " + exp(y_B, p, a));
}
private static long generatePublicKey(long g, long p, long a) {
return exp(g, p, a);
}
private static long generatePrivateKey(long q) {
return signedMod(random.nextLong(),q-3) + 1;
}
private static long generateGenerator(long p) {
long h = signedMod(random.nextLong(), (p-3))+ 2;
return (h*h) % p;
}
private static List generateSafePrimes(long limit) {
List result = new ArrayList<>();
for (long i = 2; i < limit; i++) {
if (isPrime(i) && isPrime(2*i+1)) {
result.add(i);
}
}
return result;
}
private static boolean isPrime(long n) {
for (long i = 2; i*i<=n; i++) {
if (n%i == 0) {
return false;
}
}
return true;
}
private static long exp(long g, long p, long a) {
long result = 1;
for (long i = 0; i result = (result*g)%p;
}
return result;
}
private static long signedMod(long a, long p) {
if (a < 0) {
return a - ((a / p) - 1) * p;
}
return a % p;
}
}