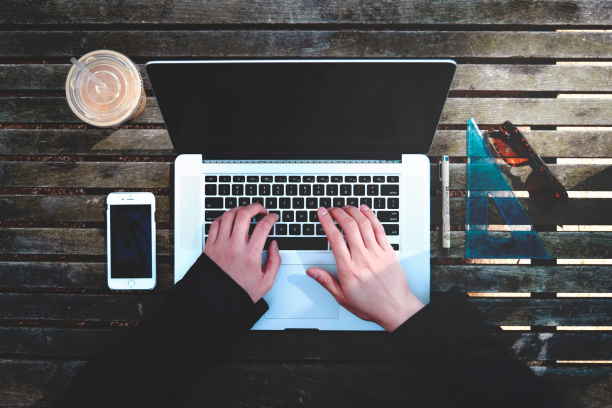
- 5th Aug 2020
- 09:32 am
The provided Java code defines a class named InfixExpressionEvaluator
, which evaluates infix arithmetic expressions with variables. The code uses two custom stack classes: MyBridgesStack
, which seems to be a stack implementation visualized through the Bridges API, and another stack class MyStack
, which is commented out.
package test; import java.lang.Math; import java.util.StringTokenizer; import test.MyBridgesStack; public class InfixExpressionEvaluator { //private MyStack stack = new MyStack(); public static double evaluateInfix(String infix, int[] values) { infix = infix.replaceAll("\\s+", ""); MyBridgesStack elements = new MyBridgesStack(); MyBridgesStack ops = new MyBridgesStack(); StringTokenizer tokens = new StringTokenizer(infix, "{}()*/+^-", true); while(tokens.hasMoreTokens()) { String token = tokens.nextToken(); int val = 0; if(token.matches("[a-f]+") || token.matches("[A-F]+") || token.matches("[0-9]+") ) { if(token.matches("[A-F]+")) { val = values[(int)token.charAt(0) - 65] ; } else if(token.matches("[a-f]+")) { val = values[(int)token.charAt(0) - 97] ; } else { val = Integer.parseInt(token); } System.out.println(val); elements.push(Double.valueOf(val)); elements.visualize(); } else if(token.equals("(")) { ops.push(token); } else if (token.equals(")")) { if(ops.isEmpty() ) { throw new IllegalStateException("IllegalStateException : Unbalanced Paranthesis."); } while ( ops.peek().charAt(0) != '(' ) { Double op2 = elements.pop(); elements.visualize(); String oprnd = ops.pop(); Double op1 = elements.pop(); elements.visualize(); double result = 0.0; if(oprnd.equals("*")) { result = op1*op2; } else if(oprnd.equals("/")) { result = op1/op2; } else if(oprnd.equals("+")) { result = op1+op2; } else if(oprnd.equals("-")) { result = op1-op2; } else if(oprnd.equals("^")) { result = Math.pow(op1, op2); } elements.push(result); elements.visualize(); if(ops.isEmpty() ) { throw new IllegalStateException("IllegalStateException : Unbalanced Paranthesis."); } } ops.pop(); } // Current token is an operator. else if (token.equals("+") || token.equals("-") || token.equals("*") || token.equals("/") || token.equals("^")) { // While top of 'ops' has same or greater precedence to current // token, which is an operator. Apply operator on top of 'ops' // to top two elements in values stack while (!ops.isEmpty() && hasPrecedence(token.charAt(0), ops.peek().charAt(0))) { Double op2 = elements.pop(); elements.visualize(); String oprnd = ops.pop(); Double op1 = elements.pop(); elements.visualize(); double result =0.0; if(oprnd.equals("*")) { result = op1*op2; } else if(oprnd.equals("/")) { result = op1/op2; } else if(oprnd.equals("+")) { result = op1+op2; } else if(oprnd.equals("-")) { result = op1-op2; } else if(oprnd.equals("^")) { result = Math.pow(op1, op2); } elements.push(result); elements.visualize(); } // Push current token to 'ops'. ops.push(token); } } while (!ops.isEmpty() && ops.peek().charAt(0) != '(') { Double op2 = elements.pop(); elements.visualize(); String oprnd = ops.pop(); Double op1 = elements.pop(); elements.visualize(); double result =0.0; if(oprnd.equals("*")) { result = op1*op2; } else if(oprnd.equals("/")) { result = op1/op2; } else if(oprnd.equals("+")) { result = op1+op2; } else if(oprnd.equals("-")) { result = op1-op2; } else if(oprnd.equals("^")) { result = Math.pow(op1, op2); } elements.push(result); elements.visualize(); } if(!ops.isEmpty() && ops.peek().charAt(0) == '(' ) { throw new IllegalStateException("IllegalStateException : Unbalanced Paranthesis."); } // Top of 'values' contains result, return it Double op33 = elements.pop(); elements.visualize(); return op33; } public static boolean hasPrecedence(char op1, char op2) { if (op2 == '(' || op2 == ')') return false; if ((op1 == '*' || op1 == '/' || op1 == '^') && (op2 == '+' || op2 == '-')) return false; else return true; } public static void main(String[] args) { int[] variableValues = {0,0,0,0,0,0}; String input = "7 / 3"; double actual = InfixExpressionEvaluator.evaluateInfix(input,variableValues); System.out.println(actual); } }
Java Programming Expert Comments on the Solution
- The
evaluateInfix
method takes an infix arithmetic expression as input and evaluates it with given variable values. - The method first removes any whitespace from the input infix expression.
- It initializes two stacks,
elements
for storing operands andops
for storing operators. - The method tokenizes the infix expression using the
StringTokenizer
class. - It iterates through the tokens and handles different cases:
- If the token is an operand (variable or number), it is pushed onto the
elements
stack. - If the token is an opening parenthesis '(', it is pushed onto the
ops
stack. - If the token is a closing parenthesis ')', it pops operators from the
ops
stack and operands from theelements
stack to evaluate the expression enclosed within the parentheses. - If the token is an operator (+, -, *, /, or ^), it checks for precedence with the operators already on the
ops
stack. It pops operators from theops
stack and operands from theelements
stack to perform the corresponding operation.
- If the token is an operand (variable or number), it is pushed onto the
- After processing all tokens, it performs the final evaluation if there are remaining operators on the
ops
stack.
The hasPrecedence
method determines the precedence of two operators, and it is used during the evaluation of infix expressions.
In the main
method, a sample infix expression "7 / 3" is provided for evaluation with variable values initialized to zero. The result of the evaluation is then printed.
Please note that this implementation assumes valid infix expressions and uses only basic arithmetic operations. If you plan to extend the code to handle more complex expressions or operators, additional modifications may be needed.
If you require clarification or additional support regarding the code, please email us - support@theprogrammingassignmenthelp.com