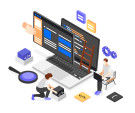
- 15th Nov 2023
- 14:18 pm
Interfaces in Java act as templates defining methods without implementations, allowing classes to implement these methods. They foster a standard structure, enabling multiple classes to share common behaviors. This abstraction mechanism promotes uniformity and flexibility in Java programming, encouraging consistent implementations across diverse class hierarchies.
What are Interfaces in Java?
Interfaces in Java serve as a blueprint for classes, defining a set of method signatures without implementations. They establish a contract, outlining the methods that classes must define upon implementation. This design enables classes to exhibit specific behavior without dictating a particular implementation, fostering standardized behavior across different class hierarchies.
Interfaces promote code adaptability by allowing classes to adhere to common structures without restricting their individual functionalities. They facilitate the creation of a common protocol shared by multiple classes, offering a clear guideline for the methods to be implemented. This abstraction mechanism separates the "what" (desired functionality) from the "how" (implementation specifics), enhancing code organization and flexibility. Interfaces are pivotal in achieving design flexibility, code standardization, and supporting the fundamental concept of polymorphism within the Java programming paradigm.
Relationship Between Class and Interface
In Java, when a class implements an interface, it commits to providing specific implementations for all the methods declared within that interface. This commitment signifies the class's dedication to adhering to the specifications outlined by the interface.
Classes implementing interfaces must define the methods outlined in the interface, ensuring the required behavior is present. This setup establishes a robust abstraction mechanism, enabling different classes to adopt a common structure without compromising their distinctive functionalities. This relationship enables a structured approach to inheritance, supporting the development of different class hierarchies while adhering to the same interface standards, contributing to code standardization, and promoting consistent behavior across diverse class implementations.
Multiple Interfaces
In Java, a class can implement multiple interfaces, allowing it to inherit the abstract behaviors specified by these interfaces. This feature supports the concept of multiple inheritances of behavior without the complexities associated with multiple-class inheritance.
By implementing multiple interfaces, a class gains access to the methods defined within each interface, thereby enabling a diverse range of functionalities and establishing a more versatile codebase. This approach empowers classes to combine various sets of methods from different interfaces, promoting flexibility in incorporating disparate functionalities and behaviors.
Multiple interface implementation contributes to a more robust and adaptable class structure, permitting the integration of different sets of methods from distinct sources while maintaining a clear and manageable code structure.
// Interface declarations
interface Walkable {
void walk();
}
interface Runnable {
void run();
}
// Class implementing multiple interfaces
class Athlete implements Walkable, Runnable {
public void walk() {
System.out.println("Walking");
}
public void run() {
System.out.println("Running");
}
public static void main(String[] args) {
Athlete athlete = new Athlete();
athlete.walk(); // Method implementation from Walkable interface
athlete.run(); // Method implementation from Runnable interface
}
}
Uses of Interfaces in Java
In Java, interfaces play a crucial role in establishing a standardized framework for classes to share common behavior without prescribing exact implementations.
- Standardized Framework: Interfaces define a common structure for classes to share behavior without specifying implementations.
- Building Block for Abstraction: They facilitate the creation of abstract behaviors, separating what is to be achieved from how it's implemented.
- Enforced Contracts: Encouraging classes to follow pre-defined contracts ensures the implementation of particular functionalities.
- Promoting Code Organization: Enable the development of structured, adaptable, and uniform code solutions.
- Flexibility in Shared Functionality: Allow different internal behaviors while implementing shared functionality.
- Consistency Across Classes: Establish a foundation for consistent behavior, promoting predictability among diverse classes in Java programs.
Advantages of Interfaces in Java
Interfaces in Java offer several advantages, contributing to the flexibility, structure, and scalability of programs:
- Achieving Abstraction: Interfaces allow the definition of abstract behaviors, separating what a class should do from how it's implemented.
- Standardization: Interfaces enforce a standard structure, guaranteeing that implementing classes conform to a predefined set of methods.
- Support for Multiple Inheritance: Facilitate the adoption of multiple interfaces, allowing classes to inherit diverse functionalities without complexities.
- Flexibility and Adaptability: Classes can implement multiple interfaces, promoting flexible code architecture.
- Code Consistency: Interfaces establish a consistent framework, aiding in predictable behavior across various classes.
- Future Expansion: They enable easy addition of new functionalities without impacting existing implementations, supporting scalable and modular code development in Java.
Default methods in Java Interfaces
In Java, the introduction of default methods within interfaces permits the addition of new functionalities to existing interfaces without causing issues with backward compatibility.
- Enhanced Interface Functionality: In Java, default methods were introduced, enabling interfaces to hold method implementations.
- Backward Compatibility: They permit the addition of new functionalities to existing interfaces without breaking compatibility with implementing classes.
- Default Implementations: Default methods contain implemented functionality, enabling interfaces to evolve without affecting existing implementations.
- Keyword Identification: Prefixed with the "default" keyword, these methods provide a default behavior within interfaces.
- Extensibility: They support the extension of interfaces with new methods, promoting adaptability and growth without impacting existing implementations.
- Evolving Interfaces: Default methods contribute to evolving interfaces, providing a way to enhance interface capabilities while maintaining backward compatibility.
// Interface with a default method
interface Printable {
default void print() {
System.out.println("Printing...");
}
}
// Class implementing the Printable interface
class Printer implements Printable {
public static void main(String[] args) {
Printer printer = new Printer();
printer.print(); // Accessing default method from interface
}
}
Private and Static Methods in Interface
In Java 9, private and static methods were introduced within interfaces to enhance their functionality. The addition of private and static methods provides a means for encapsulating logic and utility methods within interfaces, improving code organization and maintenance.
- Private Methods:
These methods can be defined within interfaces and are not part of the interface's contract. They serve as helper methods, aiding in code organization and implementation details without exposing them to implementing classes.
// Interface with a private method (Java)
interface Calculations {
default int add(int a, int b) {
return sum(a, b);
}
private int sum(int a, int b) {
return a + b;
}
}
// Class implementing the Calculations interface
class MathOperations implements Calculations {
public static void main(String[] args) {
MathOperations math = new MathOperations();
System.out.println("Sum: " + math.add(5, 7)); // Accessing default method which uses a private method
}
}
- Static Methods:
Like static methods in classes, static methods in interfaces serve as common utility methods. They cannot be overridden by implementing classes and are accessed using the interface name. These methods facilitate shared functionalities not tied to any specific instance, promoting better code organization within interfaces.
// Interface with a static method
interface Utility {
static void showInfo() {
System.out.println("This is a static method in an interface.");
}
}
// Class implementing the Utility interface
class Information implements Utility {
public static void main(String[] args) {
Information.showInfo(); // Calling the static method using the interface name
}
}