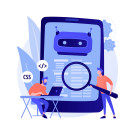
- 14th Nov 2023
- 23:13 pm
- Admin
File handling in Java refers to the capability of reading from and writing to files, granting Java applications the ability to manage file content. This functionality is crucial for application development as it facilitates interaction with external files, including binary, text, and various other file formats. File handling empowers Java applications to retrieve data from files, modify their content, or create new files, playing a fundamental role in manipulating and communicating with external file systems.
- Data Persistence: File management makes data retrieval and storage possible for all time. Writing data to files allows applications to store data that is needed beyond the program's execution. This ensures persistence by enabling data preservation even after the application ends.
- Information Sharing: Information may be transferred between various software programs or systems via files. Writing data to files allows information interchange with other systems while reading data from files makes it easier to incorporate external data into the application.
- Settings & Configuration: Applications' preferences and configuration settings are frequently kept in files. Programs are able to change their settings and behavior by reading and writing to these files.
- Logging and Reporting: Data recording, error reporting, and logging operations are supported by file handling. Programs can save important data, such as failures or occurrences, to a file for further review.
The `java.io` package contains classes and methods that are used in Java to handle files. Developers may create, read, write, update, and remove files with these classes' many functions. An essential component of Java programming for a variety of application requirements, efficient file handling guarantees effective data management, application flexibility, and smooth interaction with external data sources.
Streams in Java File Handling
Streams are a fundamental concept in Java file handling that are used to read from or write to a source, like a file or network connection. Streams enable data flow between an input/output device and a program by transferring data components in a serial fashion. The idea of streams is used by the Java I/O (Input/Output) system to effectively handle data transport.
Two primary categories of streams exist:
- Streams of Input: A software can read data from a source, such a file, by using these streams. `FileInputStream`, `BufferedInputStream`, and `DataInputStream` are a few examples. Methods for sequentially reading data from the source are provided by input streams.
- Streams of Output: A software can write data to a file or other location by using output streams. `FileOutputStream`, `BufferedOutputStream`, and `DataOutputStream` are a few examples. There are ways to write data sequentially to the destination using output streams.
Streams within Java are versatile tools for handling different data types. They possess the capability to both read and write objects, bytes, characters, and primitive data types. This versatility allows for efficient data transfer between software and external sources. Streams provide a straightforward and dependable means of conducting I/O operations, ensuring effective data transmission and manipulation within the software environment Java's streams offer a useful abstraction that makes managing files and data processing simpler, enabling developers to manage data transport efficiently.
Input Stream in Java
Java's input streams serve as a channel to fetch data from varied sources or input devices, enabling a program to collect data systematically from those sources. These streams operate by reading data sequentially from the source and are integral components within Java's input/output (I/O) system. They play a fundamental role in capturing data from different origins and input devices.
Important features and attributes of Java's input streams include:
- Sequential Reading: Data is read via input streams in a sequential fashion, enabling it to be read bigger chunks as needed or byte by byte or character by character.
- Data Sources: Input streams can be used to read from various sources, such as files (`FileInputStream`), memory arrays (`ByteArrayInputStream`), or network connections (`Socket.getInputStream()`).
- Read Methods: Input streams offer a variety of methods to read data, including `read()`, `read(byte[])`, `read(byte[], offset, length)`, and `skip(n)` for skipping bytes.
- Closes the Source: When an input stream is closed (`close()` method), it releases the resources associated with the input source, ensuring proper cleanup and preventing resource leaks.
- Exceptions Handling: During I/O operations, input stream operations may throw exceptions like {IOException}, which need to be managed to control errors.
For instance, Java's `FileInputStream` class enables data reading from files using input streams. Bit by bit or in segments as required, data may be read from the file by launching an instance of `FileInputStream` and utilizing its read methods.
All things considered, input streams are necessary for sequentially reading data from several sources, giving Java developers a flexible and standardized method for processing input in their projects.
Output Stream in Java
Java's output streams facilitate the writing of data to a destination, enabling applications to transmit data to different sources or output devices. These streams are used to write data to a target in a sequential fashion and are an essential component of Java's input/output (I/O) system.
Java output streams include several important characteristics and functionalities, including:
- Sequential Writing: Programs can publish characters, bytes, or other data kinds to a destination by using output streams, which write data sequentially.
- Destination Types: Output streams are used to write data to various destinations, such as files (`FileOutputStream`), memory arrays (`ByteArrayOutputStream`), or network connections (`Socket.getOutputStream()`).
- Write Methods: Output streams provide methods like `write()`, `write(byte[])`, and `write(byte[], offset, length)` for writing data to the output destination.
- Closing the Stream: Upon completion of writing data, it's essential to close the output stream (`close()` method) to release associated resources and ensure proper cleanup.
- Exception Handling: In order to control failures during I/O operations, handle exceptions such as {IOException` that may be thrown by output stream operations.
For example, data may be written to a file using an output stream in Java by utilizing the `FileOutputStream` class. Write operations on an instance of `FileOutputStream} allow writing data to the file, either in chunks or byte-by-byte, according to the needs.
In general, output streams are essential for publishing data in a sequential manner to different locations, offering a reliable and adaptable method of managing output in Java programs.
Java file operations include reading from, writing to, creating, deleting, and altering files, among other file management duties. Classes and methods in Java's Input/Output (I/O) API, included in the `java.io} package, enable these operations.
Key file operations in Java
- File Reading (Input Operations):
Reading data from existing files using input streams (e.g., `FileInputStream`, `BufferedInputStream`, or `Scanner`) to sequentially read file content.
- File Writing (Output Operations):
Writing or appending data to files using output streams (e.g., `FileOutputStream`, `BufferedOutputStream`, or `PrintWriter`) to store data in the file.
- File Creation and Deletion:
Creating new files (`File.createNewFile()`) and deleting existing files (`File.delete()` or `Files.delete()`).
- File Information and Properties:
Retrieving file information such as file size, existence, last modified date, or file permissions using methods like `File.length()`, `File.exists()`, `File.lastModified()`, etc.
- File Manipulation and Renaming:
Manipulating file properties (e.g., renaming files with `File.renameTo()` or checking file types with `File.isDirectory()` or `File.isFile()`).
- Directory Operations:
Working with directories, such as creating directories (`File.mkdir()`), listing directory contents, navigating directory structures, and obtaining directory paths.
File handling classes in Java are used to execute file I/O activities by offering methods for interacting with files and directories. File-based input and output in Java programs, data processing, storage management, and handling external files all depend on these activities. Further file operations capabilities are introduced by the `java.nio.file` package, which also provides more sophisticated file handling functionality.