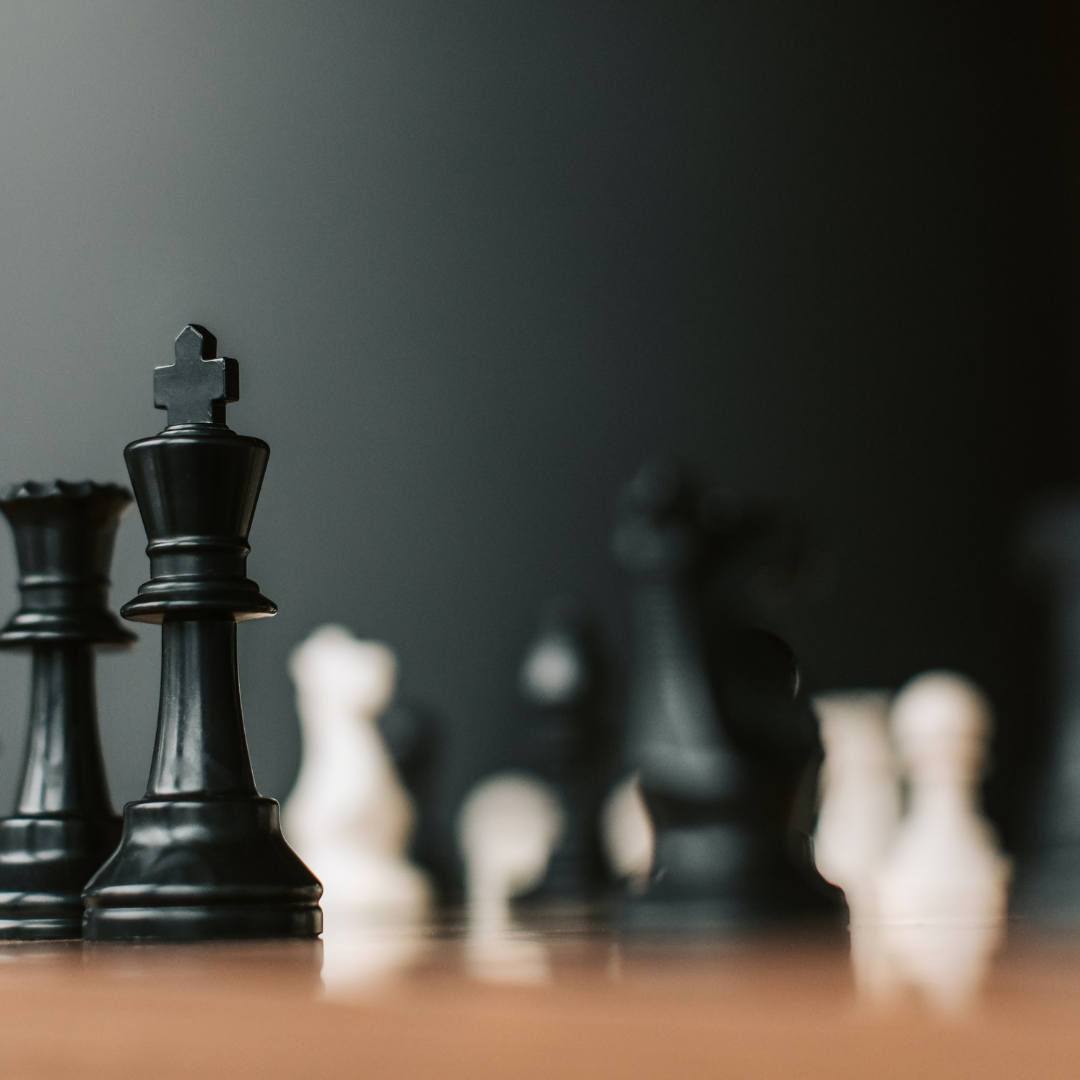
- 6th Apr 2023
- 08:50 am
Python Homework Help
Python Homework Question
Python Logical Puzzles, Games, and Algorithms: 8 Queens problem
Python Homework Solution
The 8 Queens Problem is a classic chess puzzle where the objective is to place eight queens on a standard 8x8 chessboard in such a way that no two queens threaten each other. That is, no two queens can occupy the same row, column, or diagonal.
Here's a Python program that uses the backtracking algorithm to solve the 8 Queens Problem:
def solve_queens(n):
board = [[0 for _ in range(n)] for _ in range(n)]
solutions = []
def place_queen(row):
if row == n:
solutions.append([board[i][:] for i in range(n)])
return
for col in range(n):
if is_valid_move(row, col):
board[row][col] = 1
place_queen(row+1)
board[row][col] = 0
def is_valid_move(row, col):
for i in range(row):
if board[i][col] == 1:
return False
for i, j in zip(range(row-1, -1, -1), range(col-1, -1, -1)):
if board[i][j] == 1:
return False
for i, j in zip(range(row-1, -1, -1), range(col+1, n)):
if board[i][j] == 1:
return False
return True
place_queen(0)
return solutions
# example usage
solutions = solve_queens(8)
print(f"Number of solutions: {len(solutions)}")
for i, solution in enumerate(solutions):
print(f"Solution {i+1}:")
for row in solution:
print(" ".join(str(col) for col in row))
The solve_queens function takes an integer n as input, representing the size of the chessboard. It initializes an empty n x n board, then uses a recursive place_queen function to try placing a queen on each row of the board. The is_valid_move function is used to check if a queen can be safely placed at a given row and column.
The function returns a list of all valid solutions. For each solution, it prints the board in a human-readable format.