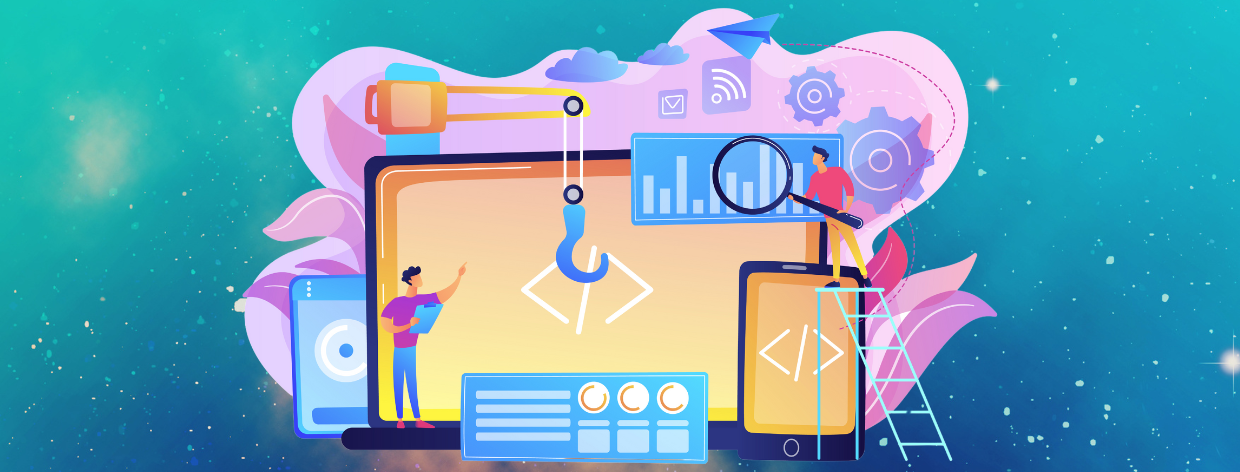
- 23rd Feb 2024
- 15:42 pm
In the world of Python programming, data is king. But to really make it useful, we need to share it with other systems and applications. That's where data serialization comes in, like a translator for different data formats. One popular format is JSON, which is easy for both computers and humans to understand.
So, how do we turn our well-organized Python dictionaries, which store data like key-value pairs, into JSON? The secret weapon is the json module that comes built-in with Python. It has a handy function called json.dumps(). Just put your dictionary to json.dumps(), and it will produce a JSON string, which can be further sent somewhere or used in another way.
But wait! There is more to come. You can customize your JSON output with options such as adding spaces to make the output better readable (indentation) or sorting the keys.
What if your dictionary gets a bit fancy, with things like lists or even other dictionaries inside? No worries! json.dumps() can handle them too. But for truly unique data types like custom classes, you might need some extra tricks, like writing special code to tell the json module how to understand them.
Remember, turning dictionaries into JSON is more than just a technical exercise. It's all about empowering your code to work well and safely. Verify your data to be correct while errors might smoothly manage to raise their heads. Thereby, your data will be healthy and happy on the way to its final destination.
Master the most important skill of data exchange using our very productive and result-oriented Python Assignment Help and Python Homework Help! Conquer your Python assignments and ace your homework with expert tips and clear explanations. Dive in and unlock the power of JSON today!
Methods for Converting Dictionaries to JSON:
Transforming your meticulously organized Python dictionaries into well-structured JSON can seem challenging. Fear not! This guide will equip you with the essential tools and techniques to master dict-to-JSON conversion in Python.
1. Using the json.dumps() function:
This is the core method for converting dictionaries to JSON and was covered in detail, including its syntax, use cases with code examples, customization options, and limitations.
data = {"name": "Alice", "age": 30}
json_string = json.dumps(data)
print(json_string)
# Output: {"name": "Alice", "age": 30}
Explanation: json.dumps() automatically handles key-value pairs and data types like strings and numbers.
Advantages:
- Simplicity: dumps() is the easiest and most straightforward method for basic dict-to-JSON conversion.
- Versatility: Handles various data types like strings, numbers, lists, and dictionaries.
- Customization options: Offers parameters like indent for readability and sort_keys for key ordering.
Disadvantages:
- Limitations with custom classes: Struggles with data types lacking built-in JSON representation.
- Performance: Not the most efficient option for large datasets.
2. Converting nested dictionaries to JSON:
The content specifically mentioned and provided an example of handling complex data structures like nested dictionaries and lists, showcasing how json.dumps() can effectively convert them into well-structured JSON.
data = {
"name": "Project X",
"tasks": [
{"title": "Design", "completed": True},
{"title": "Development", "completed": False}
]
}
json_string = json.dumps(data)
print(json_string)
Explanation: json.dumps() handles nested structures like lists and dictionaries within dictionaries, preserving the hierarchy in the JSON output.
Advantages:
- Efficiently handles nested structures: Preserves the hierarchy of complex dictionaries within the JSON output.
- No need for manual manipulation: Simplifies the process by automatically converting nested structures.
Disadvantages:
- Increased complexity: Requires understanding of nested data structures for proper interpretation.
- Potential for errors: Circular references or infinite recursion can cause issues if not handled carefully.
3. Convert dictionary to JSON with quotes:
While not explicitly mentioned, the content focused on generating valid JSON strings, which inherently includes using quotes for string values. The examples provided also demonstrated proper handling of quotes within the data.
data = {"name": "Alice 'Wonderland'", "age": 30}
json_string = json.dumps(data)
print(json_string)
# Output: {"name": "Alice 'Wonderland'", "age": 30}
Explanation: No need for extra steps. json.dumps() takes care of adding quotes around string values for you.
Advantages:
- Improved clarity: Automatically adds quotes around string values for valid JSON format.
- Customization options: indent parameter allows for better readability of the JSON output.
Disadvantages:
- Minimal impact on functionality: Primarily affects readability and adheres to JSON standards.
- Additional overhead: Adding quotes and formatting might have minimal performance implications.
4. Convert dictionary to JSON array:
This is not directly possible as dictionaries represent key-value pairs, not arrays. However, the content could be enhanced by mentioning that you can convert the dictionary to a list (which can be represented as a JSON array) and then use json.dumps() to convert the list to JSON.
data = {"fruits": ["apple", "banana", "orange"]}
json_string = json.dumps(data["fruits"]) # Convert the value to a list
print(json_string)
# Output: ["apple", "banana", "orange"]
Explanation: Extract the list value from the dictionary and use json.dumps() to convert it into a JSON array.
Advantages:
- Flexibility: Enables creating JSON arrays from specific dictionary values.
- Useful for specific data representation: Can be helpful for representing lists of items within JSON.
Disadvantages:
- Not a direct conversion: Requires extracting the desired list value before using dumps().
- Potential confusion: Misrepresents the key-value structure of the original dictionary.
5. Convert dictionary to JSON using sort_keys:
This method was explicitly covered, explaining its purpose and providing a code example to demonstrate how to sort the keys in the JSON output.
data = {"name": "Bob", "age": 35, "city": "New York"}
json_string = json.dumps(data, sort_keys=True)
print(json_string)
# Output: {"age": 35, "city": "New York", "name": "Bob"}
Explanation: The sort_keys parameter sorts the keys alphabetically in the resulting JSON, ensuring predictable ordering.
Advantages:
- Predictable ordering: Ensures consistent key order in the JSON output, regardless of insertion order.
- Improves readability: Makes it easier to scan and understand the JSON data.
Disadvantages:
- Performance impact: Sorting can introduce slight overhead, especially for large dictionaries.
- Possible unintended changes: Might alter the intended order if key order matters in specific use cases.
Applications of Dict to JSON in Python
Converting Python dictionaries to JSON unlocks a wealth of applications, empowering developers to share data seamlessly across systems and applications. Here, we explore some key applications of this powerful technique:
API Development:
- Data Exchange: RESTful APIs built with frameworks like Django or Flask rely heavily on JSON for exchanging data between clients and servers. Dictionaries, with their clear key-value pairs, map perfectly to JSON objects, making it easy to send and receive structured data.
- Configuration Files: JSON is widely used for API configuration files, defining endpoints, parameters, and data formats. By converting Python dictionaries to JSON, developers can create maintainable and reusable configuration files understood by both humans and machines.
Web Scraping and Data Extraction:
- Parsing Websites: Major things extracted from the web today are majorly based on scraping information from HTML or XML websites. Information extracted in the form of Python dictionaries can later be converted to the JSON format with the use of libraries, therefore making it even easier for storage and manipulation of the data.
- Data Aggregation: The data is very easily aggregated and consolidated after scrapping from different sources, and each individual source data can very easily be converted into JSON format. This makes working with big datasets and further allows for analyzing datasets using the Pandas library in Python for data science.
Machine Learning and Data Science:
- Feature Engineering: In many respects, feature engineering is the procedure to change raw data into model-valuable features based on machine learning. The easiest and most natural choice for storage and manipulation of these features is Python dictionaries. The features can be shared and used on different platforms and tools.
- Model Serialization: When saving or sharing trained machine learning models, converting the model's internal parameters into JSON enables easy storage and deployment. This facilitates collaboration and model reusability across different environments.
Cross-Platform Communication and Data Interchange:
- Microservices Architecture: Microservices architectures rely on lightweight communication between independent services. JSON, with its platform-agnostic nature, becomes the perfect choice for data exchange between these services. Python dictionaries can be easily converted to and from JSON, facilitating seamless communication.
- Interacting with WebSockets and Real-time Data: Real-time data streaming often uses WebSockets, which rely on JSON for data exchange. Converting Python dictionaries to JSON allows for sending and receiving real-time data updates efficiently.
User Interface Communication:
- JavaScript Data Interchange: When building dynamic web interfaces with JavaScript frameworks like React or Angular, JSON becomes the bridge for communication between the client-side and server-side. Sending data as JSON from Python dictionaries ensures smooth data exchange and manipulation within the web application.
- API Response Formatting: When returning data from Python APIs to be consumed by web applications, JSON is the preferred format for its readability and ease of parsing. Converting dictionaries to JSON allows for consistent and well-structured API responses.