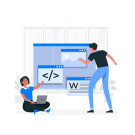
- 14th Nov 2023
- 21:16 pm
In the realm of Java programming, constructors stand as fundamental building blocks, playing a pivotal role in the creation and initialization of objects. They lay the groundwork for object instantiation, enabling the initialization of attributes and the execution of essential tasks during object creation.
What are Constructors in Java?
In Java, constructors are specialized methods within a class, sharing the same name as the class itself. They are primarily responsible for initializing objects during their creation. Unlike regular methods, constructors are automatically invoked upon object instantiation and facilitate the initialization of object attributes.
Constructors are essential in setting up the initial state of an object by assigning the initial values to its properties. They are pivotal in guaranteeing that objects are in a valid and operational state, establishing the fundamental foundation for the object to perform tasks and operations within a Java program. These constructors can vary in type, including default constructors, parameterized constructors that accept specific arguments for initialization, and copy constructors that clone existing object states. Overall, constructors are crucial in object-oriented programming, contributing significantly to object initialization and setup in Java.
How Java Constructors are Different From Java Methods?
In Java, constructors serve a unique function and operate differently from methods. Constructors, which bear the same name as the class, take on the job of initializing objects when they're created. They get automatically activated as soon as an object is brought into existence and mainly focus on setting the initial values for the object's attributes, getting them ready for use within the program.
Conversely, methods are explicitly called within the program to perform specific tasks or operations on objects. While both constructors and methods share similar syntax, constructors lack return types, unlike methods. Constructors cannot be invoked or called directly, while methods are invoked explicitly and can be called multiple times within the program, serving various functionalities. These distinctions highlight the unique roles constructors and methods play in Java's object-oriented programming paradigm.
Types of Constructors in Java
Constructors in Java come in different types, each serving a crucial role in object initialization. The default constructor assigns attributes default values, while parameterized constructors enable customization based on the arguments passed during object creation.
In Java, constructors come in several types, each serving distinct purposes in object initialization.
- Default Constructor in Java: If a class in Java does not explicitly define a constructor, Java automatically generates a default constructor. This default constructor sets object attributes to their default values, establishing the basic structure for the object.
public class MyClass {
// Default Constructor
public MyClass() {
// Initialization code or default setup
}
// Other methods or attributes can follow
}
- Parameterized Constructor in Java: Unlike the default constructor, the parameterized constructor accepts parameters. It enables personalized initialization of object attributes based on the arguments provided during object creation. This flexibility allows for customization in setting up objects.
class Student {
String name;
int age;
// Parameterized constructor
Student(String name, int age) {
this.name = name;
this.age = age;
}
void displayDetails() {
System.out.println("Name: " + name + ", Age: " + age);
}
public static void main(String[] args) {
Student student1 = new Student("Alice", 20);
Student student2 = new Student("Bob", 22);
student1.displayDetails();
student2.displayDetails();
}
}
- Copy Constructor in Java: The copy constructor is a specialized constructor used to generate a new object by replicating the attributes of an already existing object. It aids in creating a duplicate while maintaining the state of the original object.
Constructors Overloading in Java
Constructor overloading in Java encompasses defining multiple constructors within a class that differ in parameters or their number. This approach allows for versatile object creation, accommodating various initialization requirements. Each variation of a constructor can accept different arguments, providing customized ways to create and initialize objects.
By providing multiple constructor options, developers can create objects with specific attributes, adapting to various scenarios or use cases within the program. Constructor overloading enhances flexibility, simplifies object creation, and optimizes code structure by providing distinct pathways for initializing objects, bolstering the adaptability and versatility of Java programs.
public class Book {
private String title;
private String author;
// Default Constructor
public Book() {
// Default values or setup
}
// Parameterized Constructor Overloading
public Book(String title) {
this.title = title;
}
public Book(String title, String author) {
this.title = title;
this.author = author;
}
// Other methods or attributes can follow
}
Private Constructor in Java
In Java, a private constructor restricts external class instantiation, allowing object creation only within the class itself. This deliberate restriction is fundamental in design patterns such as Singleton, ensuring the existence of only one instance of the class.
By declaring a constructor as private, it prevents external classes from creating new instances, maintaining controlled access to the class. This control over object creation is vital for scenarios requiring a single, globally accessible instance or managing resource allocation efficiently within a program, ensuring adherence to specific design architectures or functionalities.
public class Singleton {
private static Singleton instance;
// Private Constructor for Singleton Pattern
private Singleton() {
// Initialization code
}
// Method to access Singleton instance
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
Constructor Chaining in Java
Constructor chaining in Java permits one constructor to call another within the same class, streamlining the process of object initialization. This method enables code reuse, as a constructor can invoke another constructor, minimizing redundancy and ensuring shared initialization logic among multiple constructors.
Connecting multiple constructors enhances organization, improving the class's structure and minimizing repetitive initialization code. Constructor chaining helps maintain consistency in object creation by enabling a primary constructor to perform common tasks, subsequently being invoked by other constructors. This approach leads to a more efficient and well-structured method for setting up objects in Java programming.
public class Vehicle {
private String brand;
private String model;
// Constructor chaining
public Vehicle() {
this("Unknown");
}
public Vehicle(String brand) {
this(brand, "Unknown");
}
public Vehicle(String brand, String model) {
this.brand = brand;
this.model = model;
}
}
Java Super Constructor
The 'super()' constructor in Java plays a significant role in inheritance, allowing a subclass constructor to call its immediate superclass constructor. This invocation facilitates the initialization of inherited members and ensures the subclass object inherits characteristics from its superclass.
By using 'super()', a subclass constructor can explicitly call the superclass constructor, initializing inherited fields before executing its own code. This mechanism maintains the inheritance hierarchy, ensuring proper initialization of both superclass and subclass attributes.
The 'super()' keyword serves as a vital feature, fostering the relationship between subclasses and superclasses, facilitating seamless object initialization and maintaining the integrity of the inheritance structure in Java programming.
class SuperClass {
int value;
SuperClass(int value) {
this.value = value;
}
}
class SubClass extends SuperClass {
int subValue;
SubClass(int value, int subValue) {
super(value);
this.subValue = subValue;
}
public static void main(String[] args) {
SubClass sub = new SubClass(10, 20);
System.out.println("Superclass value: " + sub.value);
System.out.println("Subclass value: " + sub.subValue);
}
}
The Necessity of Constructors within Java Programming
Constructors are integral as they ensure proper initialization of objects, enabling their usability within the program. They establish the starting state of objects, enabling their functionalities to be effectively utilized.
- Object Initialization: In Java Constructors are essential for initializing objects, setting initial values to their attributes, and establishing a valid state for their use within the program.
- Facilitates Functionality: Constructors establish the foundation for object functionalities, ensuring the object is prepared for operation by initializing attributes to specific values during object creation.
- Crucial Object Creation: Constructors are crucial to guarantee that objects are appropriately created and initialized, ready to execute tasks and operations within the program.
- Facilitates Object Utilization: They enable proper setup, ensuring the object is in a state where its methods and functionalities can be utilized effectively, enhancing the program's overall functionality.
- Customization and Flexibility: Different types of constructors enable customized object creation, addressing diverse requirements and scenarios within the application, offering versatility in object setup.