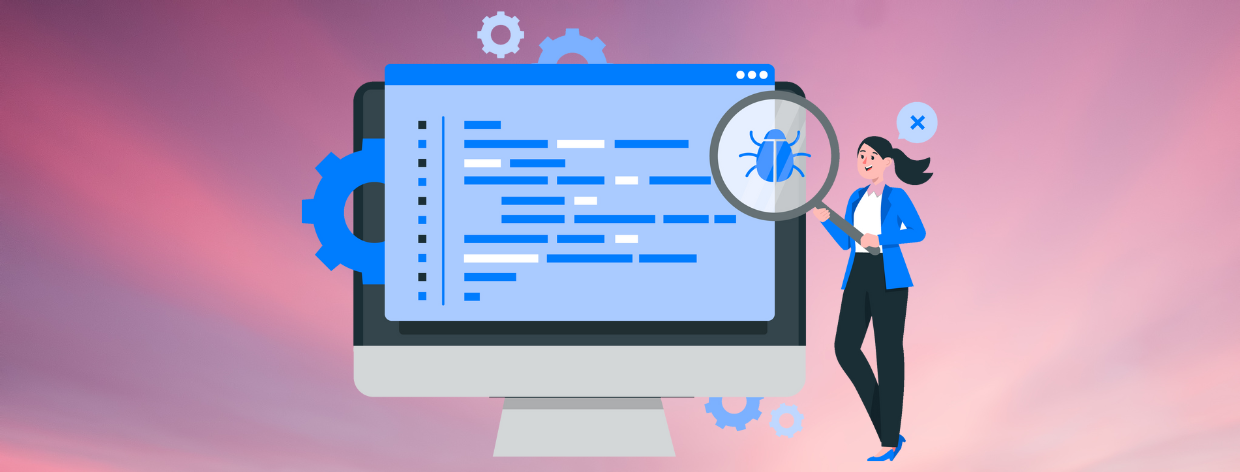
- 29th Feb 2024
- 21:40 pm
- Admin
Within the domain of C++ programming, syntax errors pose significant challenges to achieving successful program execution. These errors, akin to grammatical mistakes in natural language, disrupt the compiler's ability to accurately interpret your instructions, hindering the program's functionality. Mastering the identification and correction of such errors becomes crucial for efficient and error-free program development.
C++ programs adhere to a specific set of rules, analogous to grammar, that govern their structure and instructions. Syntax errors arise when these rules are violated, impeding the compiler's parsing process and ultimately preventing program execution. Examples of such violations include missing semicolons or misusing keywords.
The C++ compiler is your best friend when it comes to detecting these errors. It rigorously evaluates your code, line by line, for potential syntax irregularities. If an error is found, it indicates the specific location and nature of the mistake by way of an error message. These should be read and interpreted properly in conjunction with solid knowledge concerning typical mistakes made when writing syntax. This would help you develop some capability in the expertise for rapid identification and rectification of such errors such that your C++ code flows naturally, giving the result that was originally desired.
Ready to conquer common C++ syntax errors and write error-free code? We provide integrated C++ assignment help and C++ homework help—all for empowering your learning. Go through our resources, and become an assured C++ programmer.
Types of Common Syntax Errors:
Acquiring a solid understanding of common syntax errors and developing the ability to address them builds a strong foundation for your C++ programming journey.
-
Missing Semicolons:
Semicolons act as essential statement terminators in C++. Each individual statement within your code must conclude with a semicolon, signifying the end of that particular instruction. The omission of semicolons disrupts the compiler's parsing process, rendering it unable to distinguish between separate statements. This can lead to unexpected behavior or compilation errors.
Incorrect:
int age = 25
std::cout << "Hello, World!"
Corrected:
int age = 25;
std::cout << "Hello, World!" << std::endl;
-
Mismatched Parentheses:
Parentheses in C++ serve the crucial purpose of enclosing expressions and function calls. They define the order of operations within an expression and ensure the compiler interprets your code accurately. Unequal or misplaced parentheses can introduce ambiguity and lead to misinterpretations of the intended code logic.
Incorrect:
if (age > 18 {
std::cout << "You are eligible to vote";
}
Corrected:
if (age > 18) {
std::cout << "You are eligible to vote";
}
-
Incorrect Use of Operators:
C++ employs various types of operators, including arithmetic, comparison, logical, and others, to perform calculations, comparisons, and manipulations on data. Employing operators incorrectly can lead to unintended or erroneous results.
Incorrect:
if (age != 20 { // Incorrect comparison operator
std::cout << "Age is not 20";
}
Corrected:
if (age != 20) { // Corrected comparison operator
std::cout << "Age is not 20";
}
-
Variable Declaration and Initialization Issues:
Variables in C++ act as essential storage containers for data during program execution. Declaring a variable defines its name and data type, while initialization assigns an initial value. Incomplete or incorrect declaration and initialization can lead to compilation errors or unexpected program behavior.
Incorrect:
int name; // Unddeclared variable
name = "John";
Corrected:
std::string name = "John"; // Declared and initialized variable
-
Control Flow Errors:
Control flow statements determine the order of the execution of your program, including if, else, for, and while statements, thus allowing conditional branching and looping. An error here can make the program execute in any way different from your intended path.
Incorrect:
for (int i = 0; i < 5; i++) { // Missing closing brace
std::cout << i;
}
Corrected:
for (int i = 0; i < 5; i++) {
std::cout << i;
}
-
Array-Related Errors:
Arrays in C++ provide a structured way to store and access collections of elements of the same data type. Common errors related to arrays include:
- Accessing elements outside the array bounds: This can lead to undefined behavior or program crashes.
- Incorrect array declaration or initialization: This can result in compilation errors or unexpected memory access patterns.
Incorrect:
int numbers[5];
numbers[6] = 10; // Accessing element outside bounds
Corrected:
int numbers[5];
numbers[4] = 10; // Accessing element within bounds
-
Pointer Errors (Optional):
Pointers, while powerful tools for memory management, can introduce complexities if not handled carefully. Common pointer-related errors include:
- Null pointer dereferencing: When a null pointer is used to access a location in memory, this action can make a program crash.
- Memory leaks: Not deleting dynamically allocated memory in the pointers before using them can lead to continuous loss of memory space.
- Dangling pointers: Access to memory is pointed to by a pointer that no longer holds a valid memory address. This may lead to undefined behavior.
Understanding and avoiding these errors is crucial when working with pointers in C++.
Note: It is recommended to consult additional resources on pointers if the target audience is unfamiliar with them.
Tips for Preventing and Identifying Syntax Errors
Conquering syntax errors is an essential skill for any C++ programmer. These errors, akin to grammatical mistakes, can halt program execution and hinder your progress. This guide explores strategies to help you prevent and identify syntax errors, paving the way for a smoother and more efficient coding experience.
- Embrace Consistent Formatting and Indentation:
Proper code formatting and indentation go beyond aesthetics. They play a crucial role in readability and error detection. Consistent formatting makes your code easier to understand, both for yourself and others. Additionally, proper indentation visually defines code blocks, highlighting the structure of your program and making it easier to spot potential errors, such as missing braces or misplaced statements.
Example:
Poorly Formatted Code:
if(age > 18) {
std::cout << "You are eligible to vote";
} else {
std::cout << "You are not eligible to vote";
}
Well-Formatted Code:
if (age > 18) {
std::cout << "You are eligible to vote";
} else {
std::cout << "You are not eligible to vote";
}
- Leverage the Power of the C++ Compiler:
The C++ compiler acts as your first line of defense against syntax errors. It meticulously examines your code, line by line, searching for potential syntax violations. Upon encountering an error, the compiler generates an error message. This message pinpoints the exact location and nature of the error, providing invaluable clues for rectification. Pay close attention to these messages and utilize them to identify and fix errors efficiently.
- Employ Integrated Development Environments (IDEs):
Integrated Development Environments (IDEs) present an array of features that can add tremendous value to your programming experience and help in preventing syntax errors from happening in the first place. The following are just a few:
- Syntax highlighting: The elements in the code are colored differently, making it easier to recognize keywords, variables, and operators, which can thereby identify potential syntax errors such as the absence of semicolons or mismatched parentheses.
- Code completion: Suggests code snippets based on your current context, reducing typos and improving accuracy. It speeds up the process of software development by assigning small pieces of code.
- Real-time syntax error checking: Flags potential syntax errors while you code, hence enabling you to make corrections on the fly. This is checked for as you type, therefore allowing you to author code which is cleaner and easier for you to catch mistakes.
Following this code of conduct and constantly practicing the traits, one is liable to minimize all his syntax errors to a greater extent and pave for himself a worthy and operative way in the realm of C++ programming. Because the path to becoming a master of C++ includes a lot of practice with devotion, detail, and an open view toward learning from your faults.
Debugging Strategies for C++
While mastering syntax is crucial, your journey in C++ programming doesn't end there. You'll inevitably encounter logical errors and runtime exceptions that can lead to unexpected behavior even if your code compiles without syntax errors. This section delves into debugging strategies to help you tackle these challenges and become a well-rounded C++ programmer.
- Utilize Debugging Tools:
Most IDEs provide debugging tools that allow you to step through your code line by line, inspect variable values, and set breakpoints to pause execution at specific points. By using these tools, you can gain valuable insight into the flow of your program and pinpoint where issues arise.
- Employ Print Statements:
Strategically placing print statements throughout your code can offer valuable insights into the values of variables at different points in execution. This can help you identify unexpected changes in values or pinpoint where calculations go wrong. However, remember to remove unnecessary print statements before finalizing your code.
- Break Down Complex Problems:
Dividing large complex problems into smaller pieces would simplify the debugging process. This is basically because you're able to concentrate all your efforts on just one piece of code in order to track down the cause.
- Utilize Online Resources:
That huge community makes available a wealth of further online resources, including forums, tutorials, and documentation; if you are ever stuck with a really vexing problem, the C++ community is so vast that you can easily access it through online communities and search for answers related to your exact error message.
Conclusion:
Conquering syntax errors is a crucial step in becoming a proficient C++ programmer. By understanding the common challenges like misplaced semicolons, incorrect parentheses usage, and type mismatches, you can write cleaner and more reliable code. Remember, a program riddled with syntax errors simply won't run!
The key to mastering C++ syntax lies in consistent practice and vigilance. As you write more code, you may increase your eye for potential mistakes and grow to be adept at recognizing them early on. With dedication and continuous learning, you'll soon find yourself writing C++ code with confidence and a keen understanding of syntax.
About the Author
Mr. Edward Williams
Qualification: Master's degree in Computer Science
Expertise: Proficient in C++, object-oriented programming (OOP), and system programming.
Research Focus: Specializes in developing high-performance and efficient C++ applications, focusing on memory management, performance optimization, and utilizing C++ features to create robust and maintainable code.
Practical Experience: Worked on projects involving low-level system programming tasks, including device drivers, embedded systems development, and performance-critical applications. Has experience with various C++ libraries and frameworks, ensuring efficient code and adherence to best practices.
Mr. Williams is a skilled C++ programmer with a passion for system-level programming. His expertise lies in optimizing code for performance and creating robust applications that leverage the power and efficiency of C++.