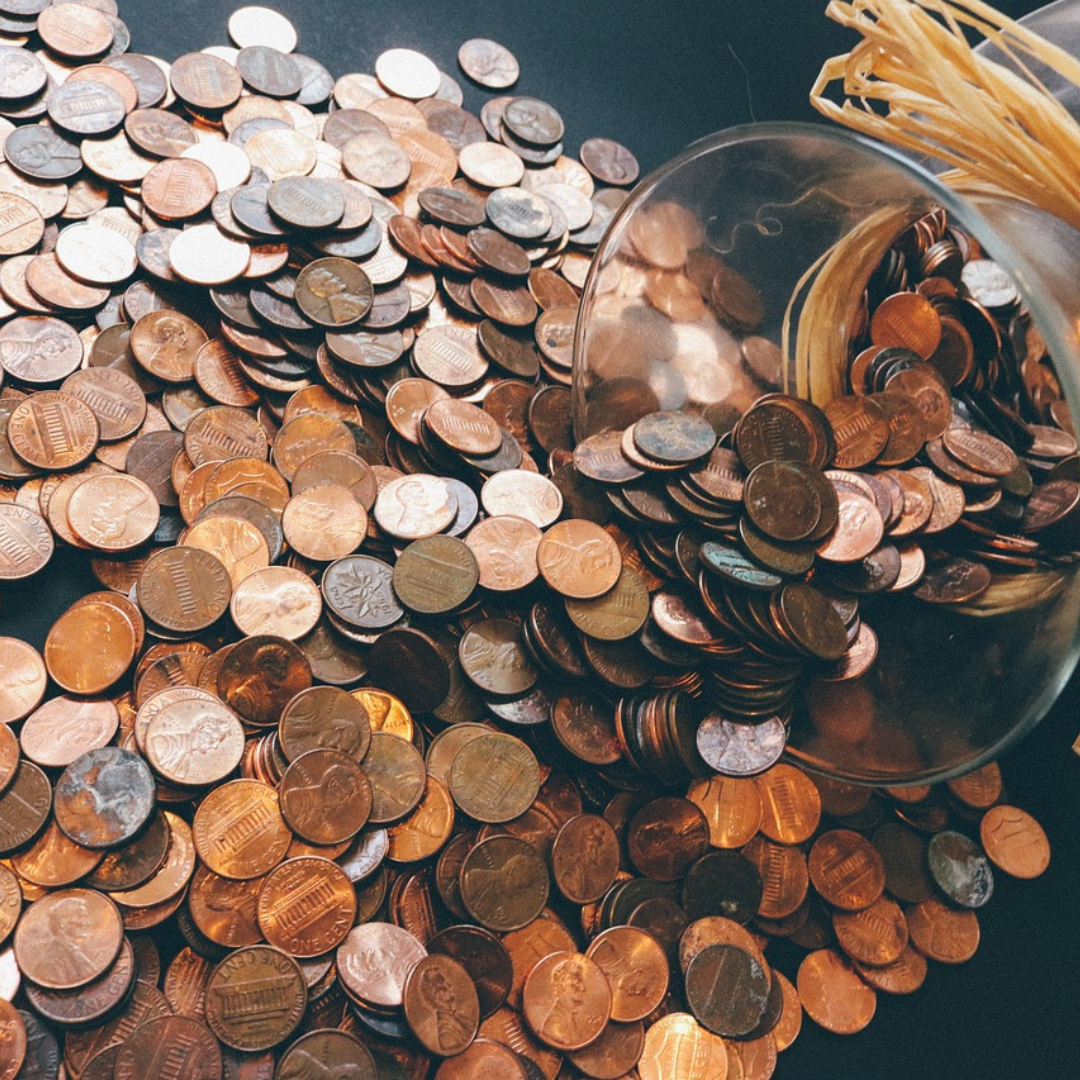
- 27th Mar 2023
- 07:57 am
- Admin
C++ Homework Question
C++ Change For a Dollar Game - Making Decisions - Create a change-counting game that gets the user to enter the number of coins required to make exactly one dollar. The program should ask the user to enter the number of pennies, nickels, dimes and quarters. If the total value of the coins entered is equal to one dollar, the program should congratulate the user for winning the game. Otherwise, the program should display a message indicating whether the amount entered was more than or less than one dollar.
C++ Homework Solution
#include <iostream>
using namespace std;
int main()
{
int pennies, nickels, dimes, quarters;
double total;
// ask the user for the number of coins
cout << "Enter the number of pennies: ";
cin >> pennies;
cout << "Enter the number of nickels: ";
cin >> nickels;
cout << "Enter the number of dimes: ";
cin >> dimes;
cout << "Enter the number of quarters: ";
cin >> quarters;
// calculate the total value of the coins
total = pennies * 0.01 + nickels * 0.05 + dimes * 0.10 + quarters * 0.25;
// check if the total is equal to one dollar
if (total == 1.00)
{
cout << "Congratulations! You won the game." << endl;
}
else if (total > 1.00)
{
cout << "Sorry, the amount entered is more than one dollar." << endl;
}
else
{
cout << "Sorry, the amount entered is less than one dollar." << endl;
}
return 0;
}
In this solution, we first ask the user for the number of pennies, nickels, dimes, and quarters, and then calculate the total value of the coins using their respective denominations. We then use an if-else statement to check if the total is equal to one dollar, more than one dollar, or less than one dollar, and display an appropriate message to the user. Note that we use the "double" data type to store the total value of the coins, since it may include fractional amounts.