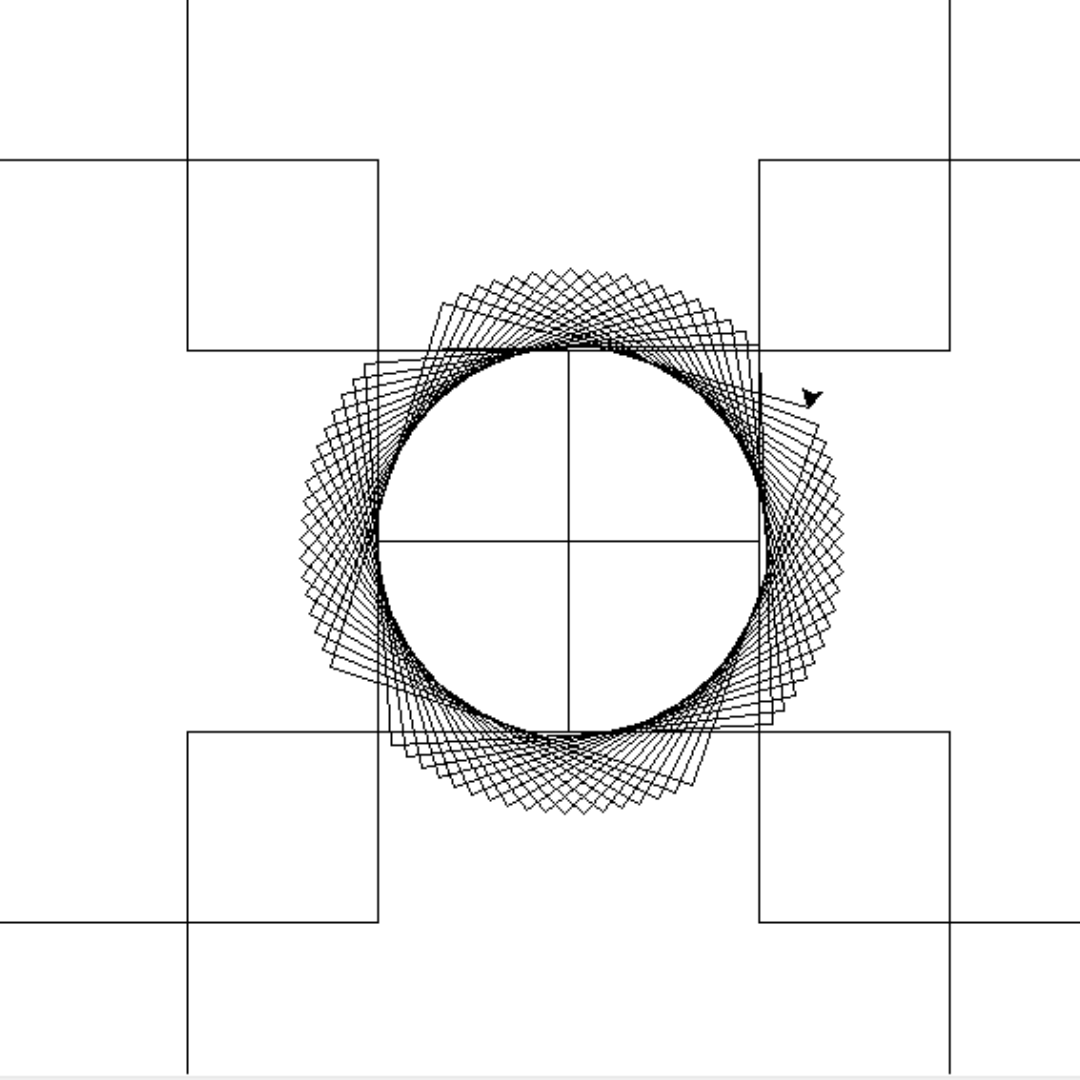
- 9th Apr 2023
- 02:59 am
- Admin
C# Homework Question
C# Logical Puzzles, Games, and Algorithms: Turtle Graphics
the provided C# program defines a Turtle
class with methods to move the turtle forward, turn left, turn right, and print its current position. The Turtle
class uses a coordinate system where the turtle starts at the origin (0, 0) and moves in a specific direction (right, down, left, or up) based on its current orientation.
In the Turtle
class, the methods are defined as follows:
-
MoveForward(int distance)
: This method moves the turtle forward in the direction it is facing by the givendistance
. The direction and distance together determine the new position of the turtle. -
TurnLeft()
: This method changes the orientation of the turtle 90 degrees to the left. -
TurnRight()
: This method changes the orientation of the turtle 90 degrees to the right. -
PrintPosition()
: This method prints the current position of the turtle in the format(x, y)
.
C# Homework Solution
using System;
using System.Collections.Generic;
using System.Linq;
namespace TurtleGraphics
{
public class Turtle
{
private int _x;
private int _y;
private int _direction;
public Turtle()
{
_x = 0;
_y = 0;
_direction = 0; // 0 = right, 1 = down, 2 = left, 3 = up
}
public void MoveForward(int distance)
{
switch (_direction)
{
case 0:
_x += distance;
break;
case 1:
_y += distance;
break;
case 2:
_x -= distance;
break;
case 3:
_y -= distance;
break;
}
}
public void TurnLeft()
{
_direction = (_direction + 3) % 4;
}
public void TurnRight()
{
_direction = (_direction + 1) % 4;
}
public void PrintPosition()
{
Console.WriteLine($"({ _x }, { _y })");
}
}
public class Program
{
public static void Main(string[] args)
{
var turtle = new Turtle();
turtle.MoveForward(10);
turtle.TurnLeft();
turtle.MoveForward(5);
turtle.TurnRight();
turtle.MoveForward(8);
turtle.PrintPosition();
}
}
}
This program defines a Turtle class with methods to move forward, turn left, turn right, and print the turtle's current position. The Program class in the Main method creates a new Turtle object and uses it to draw a simple shape.
Keep in mind that this program is a simplified version of turtle graphics, and you can expand it to implement more complex drawing functionalities and algorithms. Get help from our Programming Assignment Help Experts now.