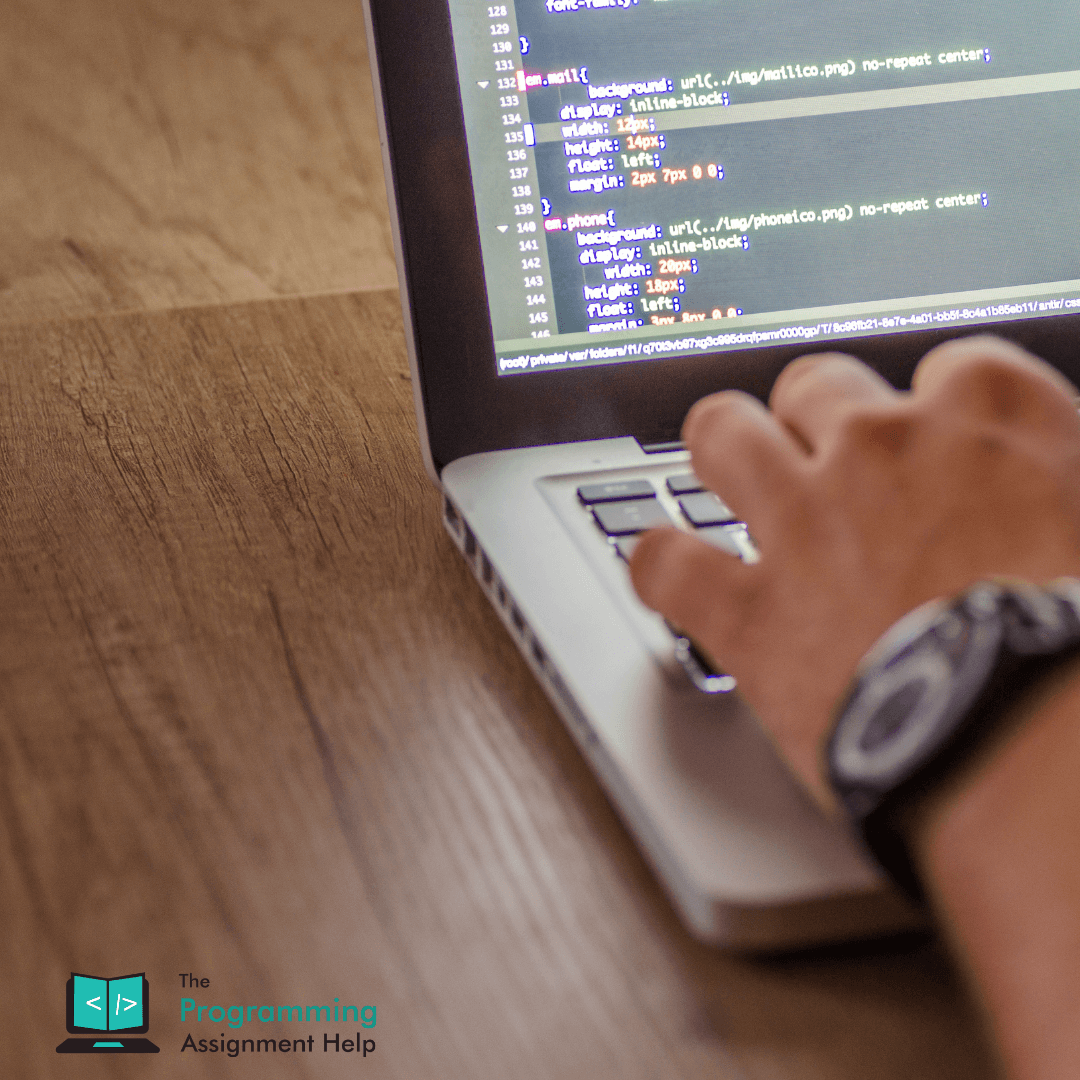
- 29th Jul 2022
- 02:49 am
- Admin
The provided C code is a program that reads lines of text from one or multiple input files (or standard input) and stores them in a linked list data structure. It then prints the lines along with line numbers. #include #include //for string manipulation #include #include #include "linked_list.h" #define BUFFER_SIZE 1024 void addToList(list_t* l, char* line) { if (l->head == NULL) { l->head = (node_t*)malloc(sizeof(node_t)); l->head->line = line; l->head->next = NULL; l->tail = l->head; } else { node_t* new_node = (node_t*)malloc(sizeof(node_t)); new_node->line = line; new_node->next = NULL; l->tail->next = new_node; l->tail = new_node; } } void printList(list_t* l) { int counter = 1; node_t* current = l->head; while(current != NULL) { printf("%03d: %s", counter, current->line); current = current->next; counter++; } } void freeList(list_t* l) { node_t* current = l->head; while(current != NULL) { node_t* node = current; current = current->next; free(node->line); free(node); } } int main (int argc, char *argv[]){ FILE *ifile = stdin; // inputfile, initit as stdin int i; char buf[BUFFER_SIZE] = {0}; // for no exceed more than BUFFER_SIZE length {0} = init everything with 0 char *file_name = NULL; char *buf_ptr = NULL; list_t list; list.head = NULL; list.tail = NULL; for(i = 1; i file_name = argv[i]; ifile = fopen(file_name, "r"); if(ifile == NULL){ perror("failed open file"); fprintf(stderr,"cannot file open : %s\n",file_name); exit(EXIT_FAILURE); } buf_ptr = fgets(buf, BUFFER_SIZE, ifile); while (buf_ptr != NULL){ int len = strlen(buf_ptr) + 1; char* line = (char *)malloc(len * sizeof(char)); strcpy(line, buf_ptr); addToList(&list, line); buf_ptr=fgets(buf, BUFFER_SIZE, ifile); } fclose(ifile); } printList(&list); freeList(&list); return(EXIT_SUCCESS); }
Here's a brief explanation of how the program works:
-
The program uses a custom linked list implementation with a node structure (
node_t
) that holds a line of text (line
) and a pointer to the next node (next
). -
The
addToList
function adds a new node with the given line of text to the end of the linked list. -
The
printList
function traverses the linked list and prints each line along with a line number (counter). -
The
freeList
function frees the memory allocated for the linked list nodes and lines. -
In the
main
function:- The program first initializes a linked list (
list
) with a head and tail set to NULL. - It loops through the command-line arguments (file names) and opens each file in read mode.
- It reads each line from the file and stores it in the linked list using the
addToList
function. - After reading all the lines from a file, the program closes the file and moves on to the next one.
- The program first initializes a linked list (
-
Finally, the program prints the contents of the linked list using the
printList
function and frees the allocated memory using thefreeList
function.
Example usage from the command line:
$ ./program_name file1.txt file2.txt
Example output:
001: Line 1 from file1.txt
002: Line 2 from file1.txt
...
...
The program prints each line from the input files along with a line number in three-digit format (padded with leading zeros for single-digit numbers). Please make sure that you have a proper implementation of the linked_list.h
header file that defines the list_t
and node_t
data structures.
If you have any specific questions or need further clarification on any part of the code, feel free to ask our programmers!