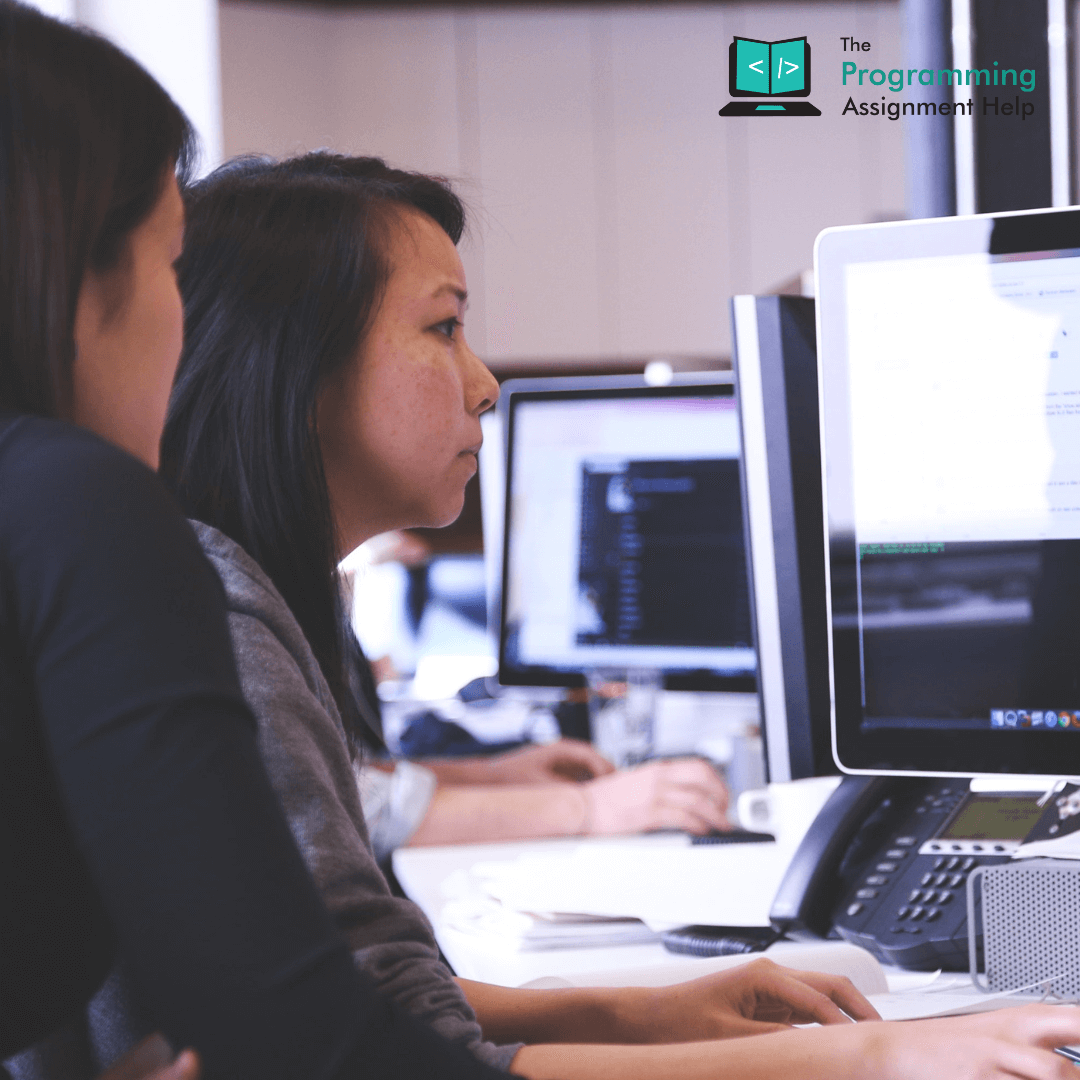
- 30th Jun 2022
- 03:18 am
- Admin
* main.cpp * * Where eliasgammacode is the encoded representation of the ASCII value (as an integer) of the character in the original file, and pos is the position * of this character in the original file. The Elias-Gamma codes in the input file are sorted in ascending order by their ASCII value (using the position in * the file in ascending order as the tie-breaker condition if the frequency of a character in the original file is greater than one). * Your program must create a child thread per Elias-Gamma code in the input file. Each child thread will decode the Elias-Gamma code assigned by * the main thread, and print the ASCII representation (character) of the integer value represented by the Elias-Gamma code. You must use the * synchronization mechanisms discussed in class to guarantee that the child threads will print the characters in order based on the position of the * character in the original file. * */ #include #include #include #include #include #include #include using namespace std; //testing int counter; // Global variable using for print the position pthread_mutex_t lock; typedef struct readThreadParams { string eliasgammacode; int position; } readThreadParams; /** 0000001000011 0 // integer = 67, ASCII = 'C' 0000001001111 1 // integer = 79, ASCII = 'O' 0000001010011 2 // integer = 83, ASCII = 'S' 0000001000011 3 // integer = 67, ASCII = 'C' 00000100000 4 // integer = 32, ASCII = ' ' 00000110011 5 // integer = 51, ASCII = '3' 00000110011 6 // integer = 51, ASCII = '3' 00000110110 7 // integer = 54, ASCII = '6' 00000110000 8 // integer = 48, ASCII = '0' 0001010 9 // integer = 10, ASCII = '\n' */ int Decode(string encoded) { int counting = 1; int count = 0; int output = 0; for (size_t i = 0; i < encoded.size(); i++) { char e = encoded[i]; if (e == '0' && counting == 1) count++; else if (e == '0' && count) count--; else if (e == '1') { counting = 0; output += pow(2, count--); } } return output; } void *threadWorker(void *arguments) { //pthread_mutex_lock(&lock); readThreadParams *data = (readThreadParams *) arguments; int result = Decode(data->eliasgammacode); //pthread_mutex_unlock(&lock); // cout << "DEBUG: INSIDE THREAD: " << data->eliasgammacode << " - " // << data->position << endl; // Wait until print out the result. Then exit while (true) { pthread_mutex_lock(&lock); if (data->position == counter) { // We should print it cout << (char) result; // Increase counter counter++; // Then this thread should be exit pthread_mutex_unlock(&lock); break; } pthread_mutex_unlock(&lock); } // Free pointer avoid memory leak delete data; return NULL; } int main(int argc, char ** argv) { string eliasgammacode; int position; int numLine = 0; // Reset counter counter = 0; // Init mutex if (pthread_mutex_init(&lock, NULL) != 0) { cout << "Mutex init has failed." << endl; return 1; } //pthread_t tids[100]; pthread_t tid; while (cin >> eliasgammacode >> position) { // cout << "DEBUG: " << eliasgammacode << " " << position << " - " // << Decode(eliasgammacode) << endl; readThreadParams *tmp = new readThreadParams(); tmp->eliasgammacode = eliasgammacode; tmp->position = position; // create thread to run the program int rc = pthread_create(&tid, NULL, threadWorker, (void*) tmp); if (rc != 0) { cout << "Thread can not be created: " << strerror(rc) << endl; } numLine++; } // Main process just want to counter increase to numLine for make sure are have been process while (true) { pthread_mutex_lock(&lock); if (numLine == counter) { // Should break to exit program pthread_mutex_unlock(&lock); break; } pthread_mutex_unlock(&lock); } return 0; }
Programming Homework Helper - Comments on the Code
The code is an implementation of a multi-threaded program that reads Elias-Gamma codes from the input, decodes them to their corresponding ASCII values (characters), and prints them out. The program uses threads to concurrently process the Elias-Gamma codes and print the characters in the order of their positions in the original file.
The main function reads the Elias-Gamma codes and positions from the input, creates a child thread for each Elias-Gamma code, and then waits for all threads to finish before exiting. The Decode function is responsible for converting the Elias-Gamma codes to their corresponding integers.
The program uses a global variable counter
and a mutex lock
to synchronize the threads' printing, ensuring that the characters are printed in the correct order based on their positions in the original file.
Overall, the program seems to be working as intended and should be able to handle the provided input correctly. However, please note that it's always a good practice to check for possible errors or exceptions when working with threads and memory allocations in a multi-threaded environment. For example, you could handle cases where memory allocation fails or add error handling for thread creation issues. Additionally, it's essential to ensure that the input file is correctly formatted to avoid any unexpected behavior.