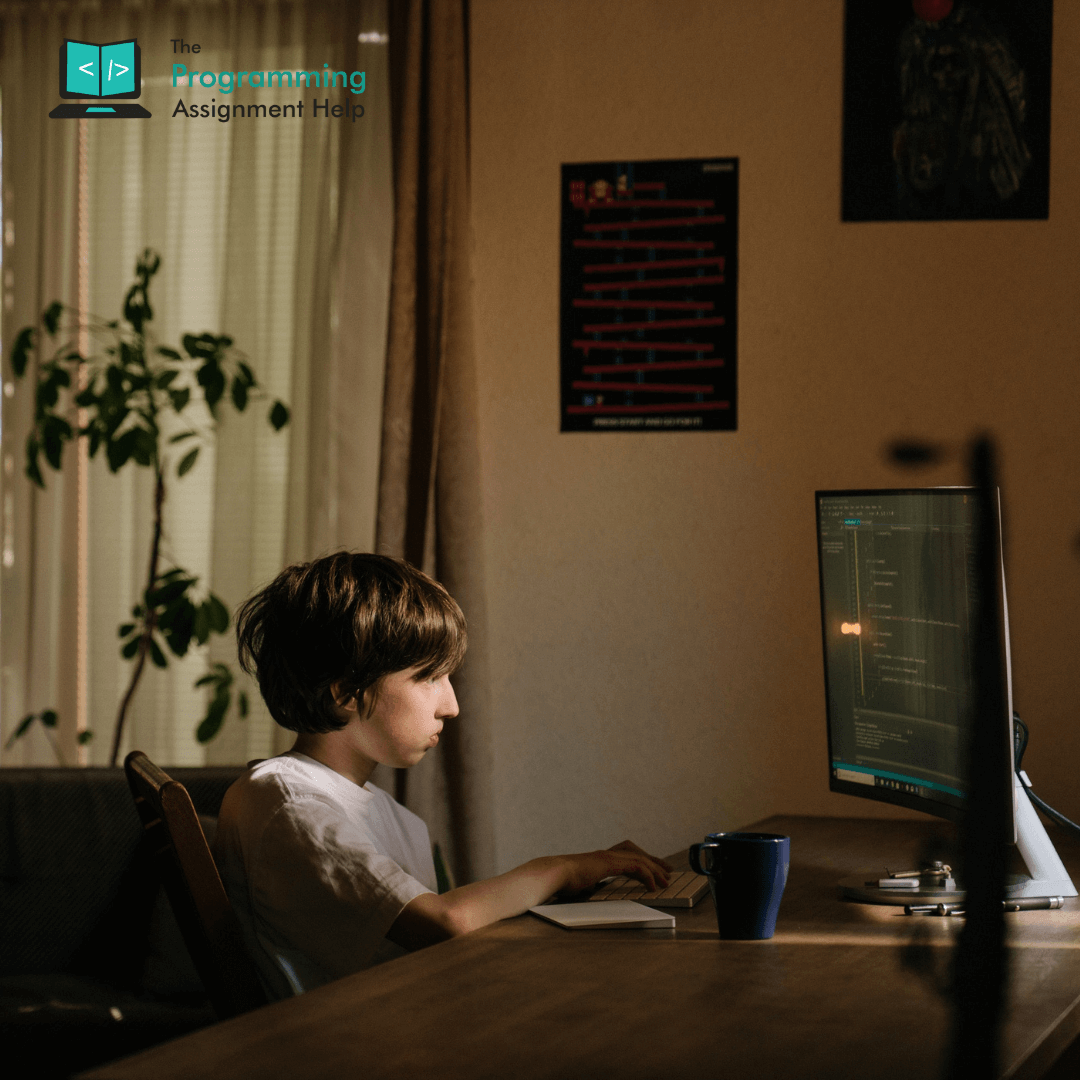
- 13th May 2022
- 02:51 am
This C program converts a binary number (entered as a string) to its corresponding floating-point representation. The function b2f
takes a binary string as input and returns its equivalent floating-point number.
#include
#include
#include
float b2f(char f_b[]){
// to store int and frac parts
int a[20],b[20];
int i,j,k,cntint,cntfra,flag,rem;
// variable for result
float rem1;
cntint=cntfra=flag=rem=0;
rem1=0.0;
// check for . and seperates binary
for(i=0,j=0,k=0;i {
if(f_b[i]=='.')
{
flag=1;
}
else if(flag==0){
a[j++]=f_b[i]-'0';
}
else if(flag==1){
b[k++]=f_b[i]-'0';
}
}
cntint=j;
cntfra=k;
// creates integer part using array stored above
for(j=0,i=cntint-1;j {
rem = rem + (a[j]*pow(2,i));
}
// creates fraction part using array stored above
for(k=0,i=1;k {
rem1 = rem1 + (b[k]/pow(2,i));
}
//result which is sum of int and fraction
rem1 = rem + rem1;
return rem1;
}
int main()
{
char s[20];
printf("Enter the binary no : ");
scanf("%s",s);
printf("%f",b2f(s));
}
Our Programming Assignment Help Expert's Analysis of the Program
-
The program includes the necessary header files for input/output, mathematical functions, and string manipulation.
-
The
b2f
function is defined to convert a binary number (as a string) to its equivalent floating-point value. The function uses two arrays,a
andb
, to store the integer and fractional parts of the binary number, respectively. It also uses variablescntint
andcntfra
to keep track of the number of digits in the integer and fractional parts,flag
to indicate if the decimal point has been encountered, andrem
to store the integer part of the converted value. The variablerem1
is used to store the fractional part of the converted value. -
The
main
function is used to get user input (a binary number as a string) and call theb2f
function to convert it to a floating-point number. The result is then printed on the console usingprintf
. -
The program assumes that the input binary number is in a valid format, with a proper number of integer and fractional digits and no invalid characters. It does not perform error handling for invalid input or handle edge cases.
-
Additionally, it is important to note that converting a binary representation to a floating-point value may result in rounding errors due to the limitations of floating-point arithmetic. This program is intended for educational purposes and may not be suitable for critical applications requiring precise floating-point calculations.