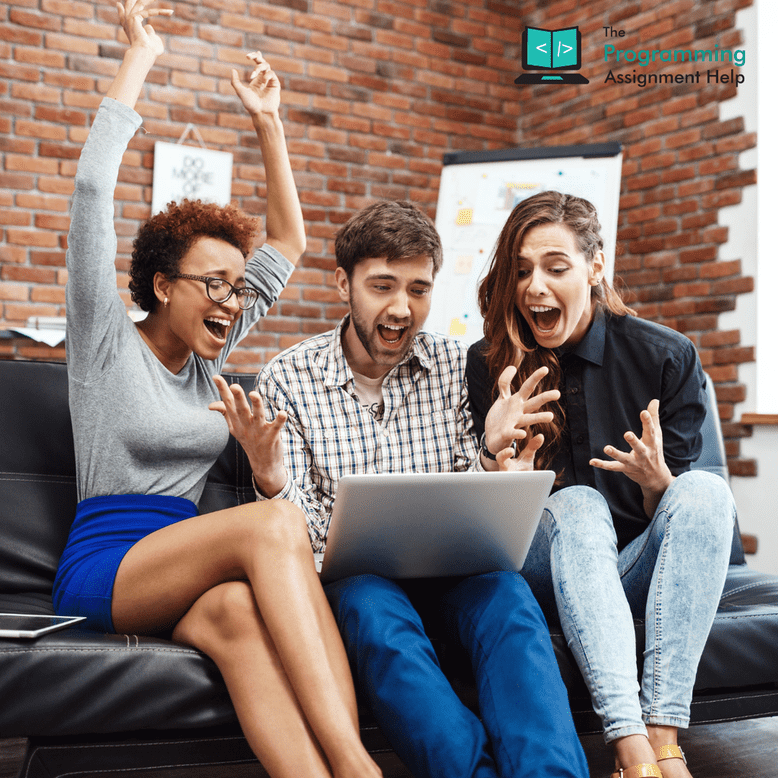
- 17th Nov 2022
- 04:29 am
The program checks the implementation of functions like intarr_create
, intarr_set
, intarr_get
, intarr_copy
, intarr_sort
, intarr_find
, and intarr_resize
. The program uses the assert
macro to check if the functions behave as expected and return the correct status codes.
#include
#include
#include
#include "intarr.h"
int main(void)
{
// Test the intarr_create function
int length = 10;
intarr_t* ptr = intarr_create(length);
assert(ptr->len==length);
for(int i=0;i assert(ptr->data[i]==0);
// Test the intarr_set function
intarr_t* ia = NULL;
intarr_result_t ret = intarr_set(ia, 10, 2);
assert(ret==INTARR_BADARRAY);
ret = intarr_set(ptr, -1, 2);
assert(ret==INTARR_BADINDEX);
ret = intarr_set(ptr, length, 2);
assert(ret==INTARR_BADINDEX);
ret = intarr_set(ptr,1, 2);
assert(ret==INTARR_OK);
assert(ptr->data[1]==2);
// Test the intarr_get function
int val;
ret = intarr_get(ia, 3, &val);
assert(ret==INTARR_BADARRAY);
ret = intarr_get(ptr, length, &val);
assert(ret==INTARR_BADINDEX);
int index = 2;
ptr->data[index] = 5;
ret = intarr_get(ptr,index,&val);
assert(val==ptr->data[index]);
assert(ret==INTARR_OK);
// Test the intarr_copy function
ia = intarr_copy(ptr);
assert(ia->len==ptr->len);
for(int i=0;ilen;i++)
assert(ia->data[i]==ptr->data[i]);
// Test the intarr_sort function
ret = intarr_sort(ptr);
for(int i=0;i<(ptr->len)-1;i++)
assert(ptr->data[i]<=ptr->data[i+1]);
assert(ret==INTARR_OK);
ia = NULL;
ret = intarr_sort(ia);
assert(ret==INTARR_BADARRAY);
// Test the intarr_find function
ret = intarr_find(ia,1,&val);
assert(ret==INTARR_BADARRAY);
for(int i=0;ilen;i++)
ptr->data[i] = i;
ret = intarr_find(ptr,5,&val);
assert(ret==INTARR_OK);
assert(val==5);
val = -2;
ret = intarr_find(ptr,15,&val);
assert(ret==INTARR_NOTFOUND);
assert(val==-2);
// Test the intarr_resize function
ret = intarr_resize(ia, 20);
assert(ia->len==20);
assert(ret==INTARR_OK);
return 0;
}
Here's a brief explanation of what the test program does:
-
Test
intarr_create
: It checks if theintarr_create
function creates an integer array of the specified length and initializes all its elements to zero. -
Test
intarr_set
: It tests theintarr_set
function, checking if it sets the value of a specific index in the integer array correctly. It also tests boundary conditions to handle invalid indices. -
Test
intarr_get
: It verifies theintarr_get
function, ensuring that it returns the value of a specific index in the integer array correctly. Likeintarr_set
, it handles boundary conditions and invalid inputs. -
Test
intarr_copy
: It tests theintarr_copy
function to check if it creates a deep copy of an integer array correctly. -
Test
intarr_sort
: It checks if theintarr_sort
function correctly sorts the elements of the integer array in ascending order. -
Test
intarr_find
: It tests theintarr_find
function, verifying if it can find a given value in the integer array and return the index of the first occurrence. -
Test
intarr_resize
: It checks if theintarr_resize
function correctly resizes the integer array to the specified length.
Note: The code relies on the implementation of the functions declared in the intarr.h
header file. The actual implementation of these functions is likely present in a separate source file.
To ensure that the program runs correctly, make sure the intarr.h
header file and its corresponding implementation file (if available) are included in the project, and the functions are implemented according to the declared prototypes.
The assert
macro is used to verify the correctness of the functions' behavior during testing. If all the assertions pass, the program will exit with a status of 0, indicating successful execution. If any assertion fails, it will trigger an assertion error, and the program execution will stop, helping to identify potential issues in the implementation.
Please let me know if you need any further explanation or assistance with this code or any other programming-related topic!