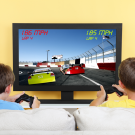
- 13th Oct 2021
- 02:41 am
This C program is a simple racing game simulation that allows the user to choose between two game modes: Auto Race (1 Player) or Manual Race (2 Player). The game reads car information from a database file, simulates a race, and then displays the race results. The user can exit the program at any time by selecting the "Quit" option. #include #include #include #include #include #include #define DATABABSE "car.txt" #define OUTPUTFILE "raceresults.txt" #define TRACKLENGTH 90 #define MAXMOVE 10 #define MINMOVE 0 #define MAXCHAR 100 #define AUTO_MODE 4 #define MANUAL_MODE 2 #define MINCARS 0 #define CARLIST 4 typedef struct CarInfo { char name[MAXCHAR]; char type[MAXCHAR]; int number; char color[MAXCHAR]; int distance; int place; } CarInfo; // Check database file exist or not int isDatabaseExist() { FILE *databaseFile; databaseFile = fopen(DATABABSE, "r"); if (databaseFile == NULL) { return 1; } else { // Close database file before return. fclose(databaseFile); return 0; } } //Prints result to file. Open file and write the result of race void writeOutput(struct CarInfo cars[], int racerNum) { int i = 0; FILE *outputFile; outputFile = fopen(OUTPUTFILE, "w"); if (outputFile == NULL) { printf("ERROR: Can not open the output file to write result\n"); return; } for (i = 0; i < racerNum; i++) { printf("%s is #%d\n", cars[i].name, i + 1); fprintf(outputFile, "%s #%d\n", cars[i].name, i + 1); } fclose(outputFile); } //Display the ranking on the screen with all the data void displayRacingInfo(int distance, struct CarInfo displayArray[], int i) { int j = 0; // Maximum number of spaces (race track) is 90 spaces if (distance > TRACKLENGTH) { distance = TRACKLENGTH; } printf("#%d ", displayArray[i].number); for (j = 0; j < distance; j++) { printf("*"); } printf("\n"); } //Try to sort the list base on place using bubble-sort void sortDatabaseBasePlace(struct CarInfo cars[], int racerNum) { int i = 0, j = 0; for (i = 0; i < racerNum; i++) { for (j = i + 1; j < racerNum; j++) { if (cars[i].place > cars[j].place) { struct CarInfo tmp = cars[i]; cars[i] = cars[j]; cars[j] = tmp; } } } } // Update place at step void updatePlace(struct CarInfo organizeArray[], int racer, int step) { if (organizeArray[racer].distance >= TRACKLENGTH) { if (organizeArray[racer].place == 0) { organizeArray[racer].place = step; } } } //Get random number with max value int getRandomNumber(int max) { return (rand() % max) + 1; } // Check all player finished or not int isFinishedRace(struct CarInfo cars[], int racerNum) { int i; for (i = 0; i < racerNum; i++) { if (cars[i].distance < TRACKLENGTH) { return 1; } } return 0; } // running race int raceRunning(struct CarInfo cars[], int racerNum) { int i, raceStep = 1, racer_pick, distance; int playerNumber = AUTO_MODE; // Check race mode. MANUAL or AUTO if (racerNum == MANUAL_MODE) { playerNumber = MANUAL_MODE; } // Clean all buffer stdin while ((i = getchar()) != '\n' && i != EOF) { } printf("Please press any key to start race!!!!!!!!!!\n"); getchar(); // Reset all infomation for (i = 0; i < playerNumber; i++) { cars[i].distance = 0; cars[i].place = 0; } // If this is manual mode if (playerNumber == MANUAL_MODE) { //Just run until meet distance do { printf("Race Step: %d\n", raceStep); // Pick on player to move racer_pick = getRandomNumber(playerNumber) - 1; // run random distance cars[racer_pick].distance = cars[racer_pick].distance + getRandomNumber(MAXMOVE); // Update place updatePlace(cars, racer_pick, raceStep); // display info to screen for (i = 0; i < playerNumber; i++) { distance = cars[i].distance; displayRacingInfo(distance, cars, i); } raceStep++; //Forces player to click button to advance the race if (playerNumber == MANUAL_MODE) { printf("Press [ENTER] to continue\n"); getchar(); } printf("\n\n\n"); } while (isFinishedRace(cars, playerNumber)); } else if (playerNumber == AUTO_MODE) { // If this is auto mode while (isFinishedRace(cars, playerNumber)) { printf("Race Step: %d\n", raceStep); // Pick player randomly racer_pick = getRandomNumber(playerNumber) - 1; // run random distance cars[racer_pick].distance = cars[racer_pick].distance + getRandomNumber(MAXMOVE); // Update place updatePlace(cars, racer_pick, raceStep); // display info to screen for (i = 0; i < playerNumber; i++) { distance = cars[i].distance; displayRacingInfo(distance, cars, i); } raceStep++; // Sleep 1 second then continue run sleep(1); printf("\n\n\n"); } } return playerNumber; } //AUTO RACE handling void raceWithAutoMode() { int i; CarInfo carDatabase[AUTO_MODE]; FILE *inputFile = fopen(DATABABSE, "r"); if (inputFile == NULL) { printf("Error when read the database. Please check database file: %s\n", DATABABSE); return; } // Try to read all car information for (i = 0; i < CARLIST; i++) { fscanf(inputFile, "%s %s %d %s", carDatabase[i].name, carDatabase[i].type, &carDatabase[i].number, carDatabase[i].color); } // Close file fclose(inputFile); printf("=== THIS IS AUTO MODE RACE ========== (1 Players)\n"); // Run race raceRunning(carDatabase, AUTO_MODE); // Try to sort database after run. Then write to ouput file sortDatabaseBasePlace(carDatabase, AUTO_MODE); // Write to output file writeOutput(carDatabase, AUTO_MODE); } //MANUAL RACE HANDLING void raceWithManualMode() { int i, k, player, validChoiceCar, carChoice, selection, j = 0, racerNum = MANUAL_MODE; CarInfo carDatabase[AUTO_MODE]; CarInfo playerCars[racerNum]; FILE *inputFile = fopen(DATABABSE, "r"); if (inputFile == NULL) { printf("Error when read the database. Please check database file: %s\n", DATABABSE); return; } // Try to read all car information for (i = 0; i < CARLIST; i++) { fscanf(inputFile, "%s %s %d %s", carDatabase[i].name, carDatabase[i].type, &carDatabase[i].number, carDatabase[i].color); } //Close database file after read fclose(inputFile); printf("=== THIS IS MANUAL MODE RACING=== (2 Players)\n"); // Assign car to player do { //Do loop for trapping until proper selection is made do { // Flag for choice valid car validChoiceCar = 0; //For player number player = j + 1; printf("\nPlayer %d, Please select your car:\n", player); // Print car for choice for (i = 0; i < CARLIST; i++) { k = i + 1; printf("\t%d) %s %s %d %s\n", k, carDatabase[i].name, carDatabase[i].type, carDatabase[i].number, carDatabase[i].color); } printf("\n Your choice: "); scanf("%d", &carChoice); if (carChoice <= MINCARS || carChoice > CARLIST) { // This is invalid choice printf("Invalid choice. Please try again.\n"); } else { selection = carChoice - 1; printf("You have selected car #%d, the %s %s driven by %s\n", carDatabase[selection].number, carDatabase[selection].color, carDatabase[selection].type, carDatabase[selection].name); // Copy to playerCar choice playerCars[j] = carDatabase[selection]; } //If player 2 selects same car as player 1, set trap to redo selection if (playerCars[1].number == playerCars[0].number && playerCars[0].number != 0 && playerCars[1].number != 0) { printf("Can not choice the same card. Please try again.\n"); // Reset playerCars playerCars[0].number = playerCars[1].number = 0; validChoiceCar = 0; j = 0; } // If this is valid choice else { validChoiceCar = 1; } } //Loop until valid choice while (validChoiceCar != 1); //Counter for player car selection loop j++; } //Repeat until read enough two player while (j < MANUAL_MODE); //Run racing raceRunning(playerCars, j); //Sort player due to place sortDatabaseBasePlace(playerCars, j); // Write output writeOutput(playerCars, racerNum); } int main(int argc, char** argv) { // The first output (display) must be your name printf("\n"); int choice; do { printf("Please choice option to run:\n"); printf("\t1) Auto Race (1 Player)\n"); printf("\t2) Manual Race (2 Player)\n"); printf("\t3) Quit\n"); printf("Your option: "); scanf("%d", &choice); if (choice == 1) { raceWithAutoMode(); } else if (choice == 2) { raceWithManualMode(); } else if (choice == 3) { printf("Bye!!! Exiting!\n"); } else { printf("Invalid choice. Please try again\n"); } } while (choice != 3); return 0; }
Our C Programming Assignment Expert Analysis on the Programming Solution
-
The program includes necessary header files for input/output, file handling, and sleeping.
-
The program defines several constants and a structure
CarInfo
to store information about cars participating in the race. -
The program contains several functions for handling different aspects of the game, such as checking if the database file exists, writing the race results to an output file, displaying the racing information, sorting the cars based on their positions, updating the place of a car at each step, generating random numbers for racing, checking if all players have finished the race, and running the race in auto and manual modes.
-
The main function is used to present a menu to the user and allow them to choose between auto and manual race modes or quit the game. Depending on the user's choice, the corresponding race function is called.
-
The auto race function
raceWithAutoMode
reads car information from the database file, runs the race simulation in auto mode, sorts the cars based on their positions, writes the race results to an output file, and displays the results. -
The manual race function
raceWithManualMode
also reads car information from the database file, but in this case, it allows two players to select their cars for the race. Then, it runs the race simulation in manual mode, sorts the cars based on their positions, writes the race results to an output file, and displays the results. -
The program uses various loops, conditional statements, and functions to manage the race simulation, handle user input, and perform file operations.