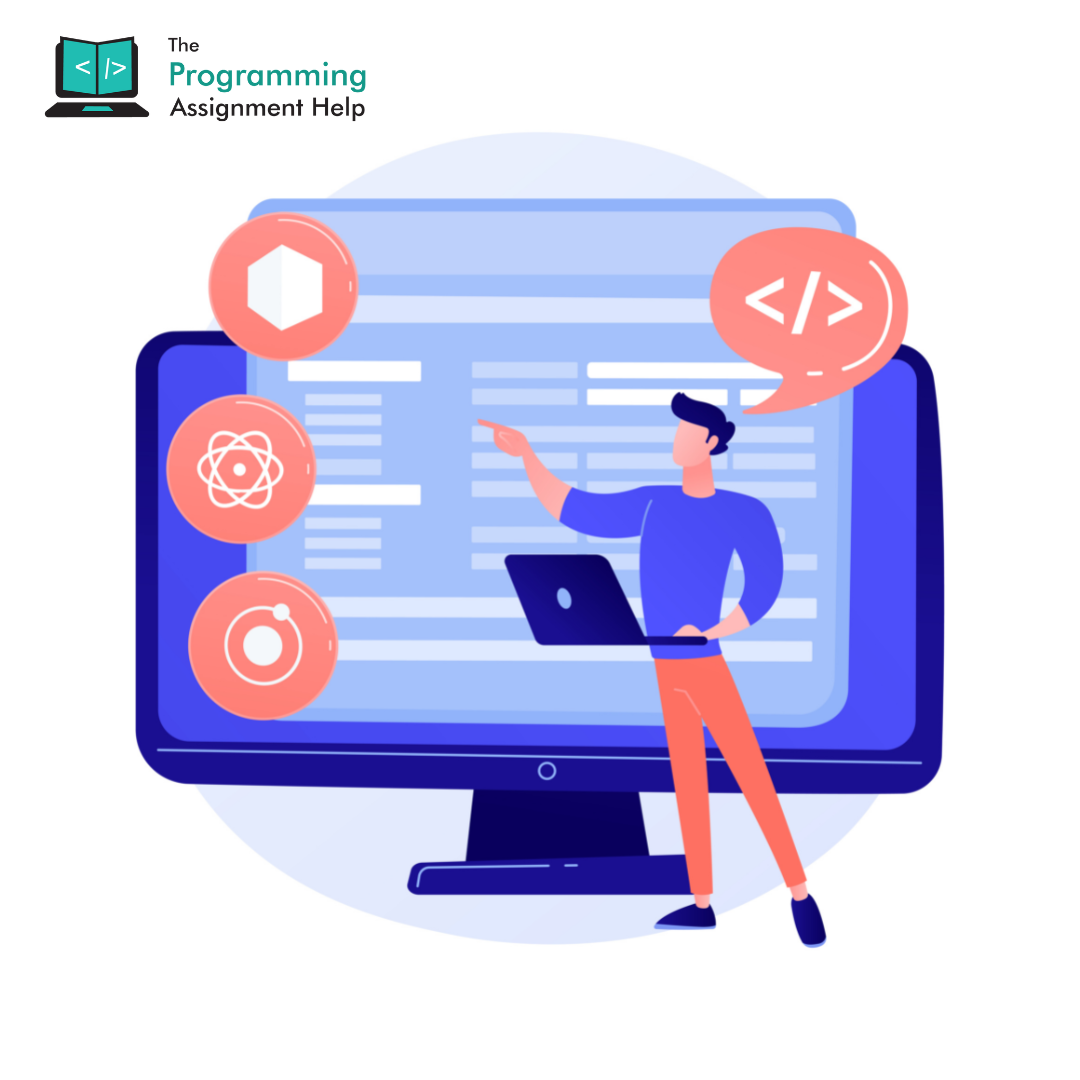
- 22nd Dec 2022
- 03:43 am
- Admin
Student's C++ Programming Assignment Help - Solution on Strings Class
#include
#include
#include
#include
#include
using namespace std;
int main()
{
int sizeOfVector;
vector wordList;
vector wordListCount;
vector stopWord{"a","an","as","be","buy","for","i","is","it","or"};
fstream fp;
string filename = "English.txt";
string word;
fp.open(filename.c_str());
if (!fp)
cout << "\nFile not found\n";
while (fp >> word)
{
for (int i = 0, j = word.size(); i < j; i++)
{
if (ispunct(word[i]))
{
word.erase(i--,1);
j = word.size();
}
}
wordList.push_back(word);
}
for (auto i = stopWord.begin(); i != stopWord.end(); ++i)
{
for (auto j = wordList.begin(); j != wordList.end(); ++j)
{
string temp=*j;
transform(temp.begin(), temp.end(), temp.begin(), ::tolower);
if (temp == *i)
{
wordList.erase(j);
j--;
}
}
}
for (auto j = wordList.begin(); j != wordList.end(); ++j)
{
wordListCount.push_back(count(wordList.begin(), wordList.end(), *j));
}
sizeOfVector=wordList.size();
for (int i = 0; i < sizeOfVector-1; i++)
{
for (int j = 0; j < sizeOfVector-i-1; j++)
{
if (wordListCount[j] < wordListCount[j+1])
{
int tempcount=wordListCount[j];
wordListCount[j]=wordListCount[j+1];
wordListCount[j+1]=tempcount;
string tempWord=wordList[j];
wordList[j]=wordList[j+1];
wordList[j+1]=tempWord;
}
}
}
cout<<"\nThree most Frequent word \n";
string printedWord[3]={"","",""};
int index=0;
for(int i=0;i {
int found=0;
for(int j=0;j {
if(printedWord[j]==wordList[i])
{
found=1;
}
}
if(found==0)
{
cout<<"\nword : "< cout<<"\nOccurence : "< cout< printedWord[index]=wordList[i];
index++;
}
if(index==3)
break;
}
return 0;
}
Programming Assignment Help Expert's Comments on the Coding Solution
It seems like the implemented C++ program reads words from a file, removes punctuation and common stopwords, and then counts the occurrences of each word. Finally, it sorts the words based on their occurrences in descending order and displays the three most frequent words. The overall functionality of the program appears to be correct.
However, a few things that could be improved or adjusted:
-
Including headers: There are some missing header includes. Make sure to include the necessary headers like
<vector>
,<string>
,<fstream>
, and<algorithm>
for the program to work correctly. -
Using
vector<string>
: It seems like you intended to use avector<string>
to store the words and their counts. In C++, the correct syntax for a vector of strings isvector<string>
, notvector
. -
Optimizing word counting: Instead of using nested loops to count the occurrences of words, you can use an
unordered_map<string, int>
to store each word as the key and its count as the value. This will significantly improve the efficiency of the word counting process. -
Checking for punctuation: The current approach for removing punctuation from words is not optimal. You can use the
isalpha
function to check if a character is an alphabet character and keep only those characters in the word. -
Handling uppercase letters: You are converting words to lowercase to handle stopwords, but this may cause different words that differ only in case to be counted separately. To handle this, you can either convert all words to lowercase during the counting process or use a case-insensitive hash function in the
unordered_map
. -
Properly closing the file: Don't forget to close the file after reading it using
fp.close()
.