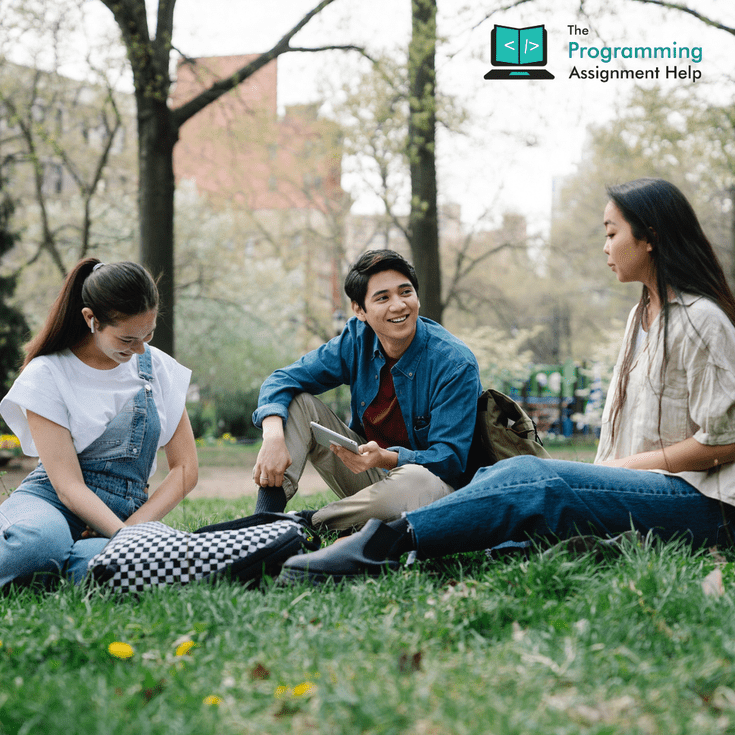
- 19th Oct 2022
- 12:25 pm
- Admin
Question - C++ program calculates the sum and average of 5 numbers based on the user's choice.
C++ Programming Assignment Help - Solution on Sum and the Average of The Numbers
#include
#include
using namespace std;
int sumOfArray(int arr[5])
{
int sum =0;
for(int i=0;i<5;i++)
{
sum = sum+arr[i];
}
return sum;
}
float avgOfArray(int arr[5])
{
int sum =0;
float avg;
for(int i=0;i<5;i++)
{
sum = sum+arr[i];
}
avg = (float)sum/5;
return avg;
}
int main()
{
int arr[5];
int sum ;
float avg;
int userChoice;
cout<<"Enter 5 numbers "< for(int i=0;i<5;i++)
{
cin>>arr[i];
}
cout<<"Press 1 calculate the average of the numbers entered"< cout<<"Press 2 calculate the sum of the numbers entered"< cout<<"Press 3 calculate both the sum and the average of the numbers entered"< cin>>userChoice;
switch(userChoice)
{
case 1:
{
avg = avgOfArray(arr);
cout<<"The average of the numbers entered is: "< break;
}
case 2:
{
sum = sumOfArray(arr);
cout<<"The sum of the numbers entered is: "< break;
}
case 3:
{
sum = sumOfArray(arr);
avg = avgOfArray(arr);
cout<<"The average of the numbers entered is: "< break;
}
default:
{
cout<<"Wrong Input by User"< }
}
return 0;
}
Student - do My C++ Programming Assignment
Overall, it looks good and should work as intended, but there are a few minor improvements that can be made:
-
Missing
cout
statements: The output messages for the average and sum calculations are missing the actual values. Make sure to include the values in the output statements. -
Displaying average with two decimal places: You can use
fixed
andsetprecision
manipulators from the<iomanip>
header to display the average with two decimal places for better presentation. -
Resetting
sum
variable inavgOfArray
: In theavgOfArray
function, you can reset thesum
variable to zero before calculating the sum of array elements. -
Formatting the output of average: When displaying the average, you can format it to show exactly two decimal places for a more consistent and cleaner output.
Revised version of the code
#include <iostream>
#include <iomanip>
using namespace std;
int sumOfArray(int arr[5])
{
int sum = 0;
for (int i = 0; i < 5; i++)
{
sum = sum + arr[i];
}
return sum;
}
float avgOfArray(int arr[5])
{
int sum = 0;
for (int i = 0; i < 5; i++)
{
sum = sum + arr[i];
}
return static_cast<float>(sum) / 5;
}
int main()
{
int arr[5];
int sum;
float avg;
int userChoice;
cout << "Enter 5 numbers: ";
for (int i = 0; i < 5; i++)
{
cin >> arr[i];
}
cout << "Press 1 to calculate the average of the numbers entered" << endl;
cout << "Press 2 to calculate the sum of the numbers entered" << endl;
cout << "Press 3 to calculate both the sum and the average of the numbers entered" << endl;
cin >> userChoice;
switch (userChoice)
{
case 1:
avg = avgOfArray(arr);
cout << "The average of the numbers entered is: " << fixed << setprecision(2) << avg << endl;
break;
case 2:
sum = sumOfArray(arr);
cout << "The sum of the numbers entered is: " << sum << endl;
break;
case 3:
sum = sumOfArray(arr);
avg = avgOfArray(arr);
cout << "The sum of the numbers entered is: " << sum << endl;
cout << "The average of the numbers entered is: " << fixed << setprecision(2) << avg << endl;
break;
default:
cout << "Wrong Input by User" << endl;
}
return 0;
}