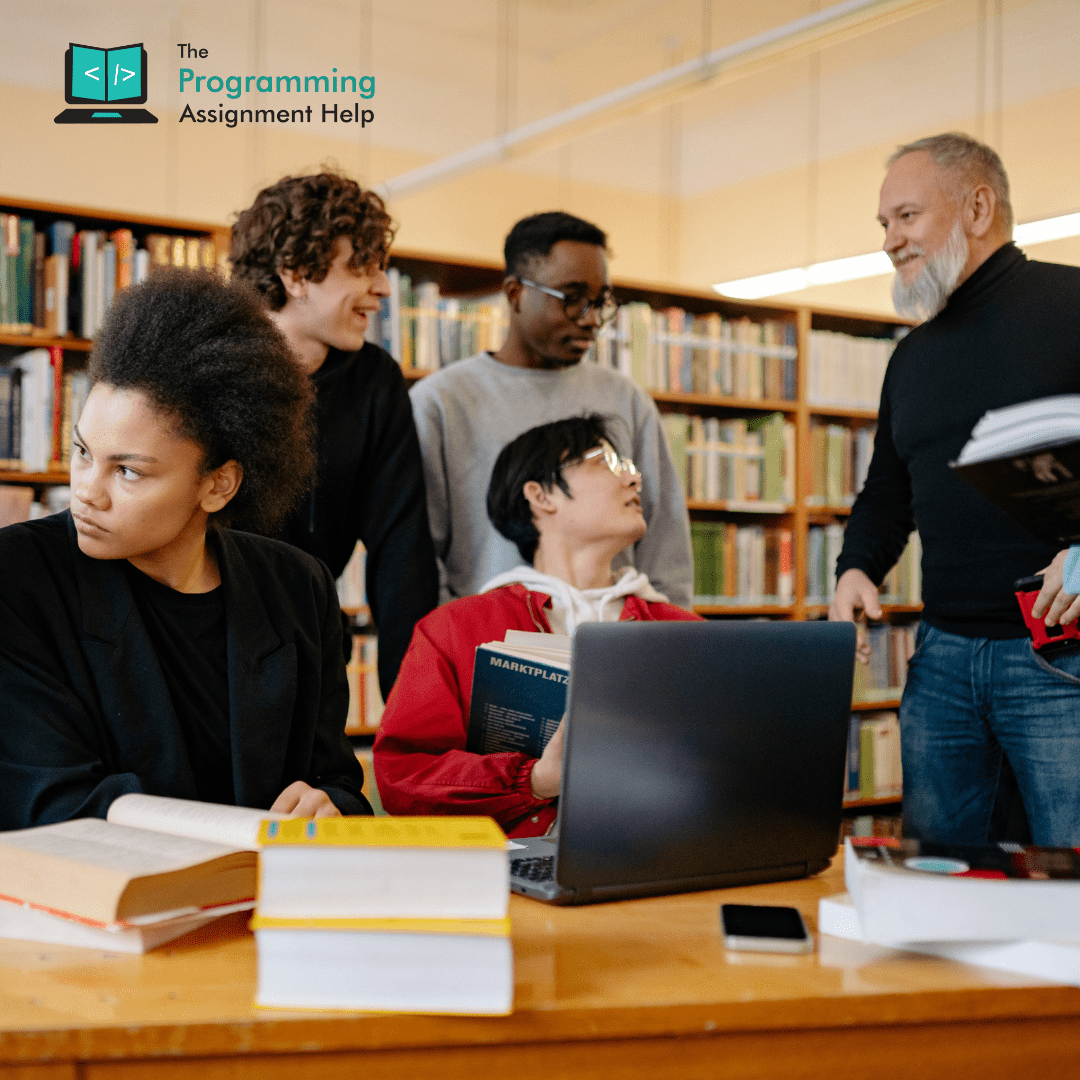
- 4th Nov 2022
- 03:37 am
This C++ program appears to demonstrate the usage of a Max Heap data structure implemented in the "maxheap20.h" header file. The program allows the user to insert elements into the Max Heap and remove elements from it.
Solution
#include
#include
using namespace std;
#include "maxheap20.h"
int main()
{
heap h;
int rv, x;
cout << "Value to insert (-1 to stop): ";
cin >> x;
while (x != -1)
{
h.add( x );
h.printA();
cout << "Value to insert (-1 to stop): ";
cin >> x;
}
cout << "-1 to remove 0 to stop: ";
cin >> x;
while ( x == -1 )
{
rv = h.remove( );
cout << "removed " << rv << endl;
h.printA();
cout << "-1 to remove 0 to stop: ";
cin >> x;
}
return 0;
}
The program provides a simple interactive interface for inserting elements into the Max Heap and removing the maximum element repeatedly until the user decides to stop. The Max Heap ensures that the maximum element remains at the root of the heap.
To fully understand the behavior of the program, it's essential to review the contents of the "maxheap20.h" header file to see how the heap
class is defined and what methods it provides for insertion (add
) and removal (remove
) operations, as well as for printing the current state of the heap (printA
).