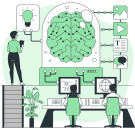
- 17th Nov 2023
- 21:50 pm
- Admin
Dynamic Memory Management in C++ stands as a crucial element, offering adaptability and efficiency in the administration of memory resources. Diverging from static memory allocation, the dynamic approach empowers programmers to allocate and deallocate memory at runtime, facilitating the development of applications that are not only adaptable but also resource-efficient.
What is C++ Dynamic Memory Management?
Dynamic Memory Management in C++ is a pivotal aspect, empowering developers to allocate and deallocate memory during runtime. This dynamic approach, distinct from static memory, offers flexibility and adaptability in handling memory resources. Achieved through operators like new and delete, dynamic memory allocation enables the creation of resizable arrays, dynamic objects, and flexible data structures. However, it demands cautious manual management to prevent memory leaks. Integrating smart pointers enhances reliability, automating memory deallocation. While dynamic memory management provides flexibility, it introduces complexities and the potential for allocation failures, requiring meticulous programming practices for optimal performance and resource utilization.
Types of Memory Management
Memory management in C++ is broadly categorized into static and dynamic types.
- Static Memory:
Static memory is assigned during compile time and possesses a constant size established by the program's structure.
Variables declared with static storage duration, such as global variables, fall under this category.
While static memory is efficient for certain use cases, it lacks the flexibility offered by dynamic memory.
- Dynamic Memory:
Dynamic memory, in contrast, is assigned and released during program execution.
It offers adaptability in handling memory resources according to the program's requirements.
Dynamic memory management is particularly useful when the size of data structures is not known at compile time.
Dynamic Memory Allocation in C++
Dynamic memory allocation in C++ involves the utilization of the operators' new and delete (or malloc and free from C). The new operator is employed to allocate memory on the heap, and the delete operator is used to deallocate the allocated memory.
int* dynamicInt = new int;
*dynamicInt = 42;
delete dynamicInt;
In this scenario, we dynamically allocate memory for an integer, assign a value to it, and subsequently deallocate the allocated memory.
Dynamic Memory for Arrays
Dynamic Memory for Arrays in C++ programming allows the creation of arrays with sizes determined during runtime, overcoming the limitations of fixed-size static arrays. Employing the new[] operator enables the allocation of memory for dynamic arrays, providing adaptability and efficient memory usage.
However, it requires responsible manual management using the corresponding delete[] operator to prevent memory leaks and ensure optimal resource utilization.
int size;
std::cout << "Enter the size of the array: ";
std::cin >> size;
int* dynamicArray = new int[size];
delete[] dynamicArray;
This illustration showcases the dynamic allocation and deallocation of an array, where the size is determined during program execution.
Dynamic Memory for Objects
Dynamic Memory for Objects in C++ is achieved through the new operator, allowing the creation of objects with sizes determined during runtime. This dynamic approach enhances adaptability and flexibility in handling object creation and resource utilization.
class MyClass {
public:
MyClass() { std::cout << "Object created!" << std::endl; }
~MyClass() { std::cout << "Object destroyed!" << std::endl; }
};
MyClass* dynamicObject = new MyClass;
delete dynamicObject;
Memory Leak Prevention
Tackling memory leaks presents a considerable challenge in the domain of dynamic memory management. These leaks occur when allocated memory fails to undergo proper deallocation, leading to a gradual depletion of available memory over time.
Adhering to best practices, including the systematic deallocation of memory after use, becomes imperative to circumvent memory leaks in C++ programming.
int* dynamicInt = new int;
In this example, not deallocating memory using delete can result in a memory leak.
Smart Pointers for Resource Management
In C++, smart pointers, such as std::unique_ptr and std::shared_ptr, are introduced to enhance memory management and reduce the risk of memory leaks. These smart pointers automatically handle memory deallocation, alleviating the burden on the programmer.
#include
std::unique_ptr smartInt = std::make_unique(42);
Smart pointers provide a safer and more convenient alternative to manual memory management.
Memory Allocation Failures
Dynamic memory allocation can fail if there is insufficient memory available. It is crucial to handle allocation failures appropriately to maintain program stability.
int* dynamicArray = new (std::nothrow) int[size];
if (dynamicArray == nullptr) {
std::cerr << "Memory allocation failed!" << std::endl;
} else {
delete[] dynamicArray;
}
The std::nothrow option allows dynamic memory allocation without throwing an exception in case of failure.
Advantages of C++ Dynamic Memory Management
C++ Dynamic Memory Management offers several advantages, including adaptability and flexibility in handling memory resources.
- Adaptability: Resizable data structures based on runtime requirements. Efficient handling of varying program needs.
- Flexibility: Creation of dynamic arrays and objects. Optimal memory utilization.
- Efficiency: Prevents unnecessary resource wastage. Responsive program structure.
- Versatility: Supports adaptable and dynamic program development.
- Optimization: Enables optimized resource utilization. Improved program performance with responsible usage.
Disadvantages of C++ Dynamic Memory Management
Despite its advantages, C++ Dynamic Memory Management introduces complexities and potential pitfalls.
- Manual Management: Requires manual allocation and deallocation.
- Memory Leaks: Potential risk if not handled meticulously.
- Allocation Failures: Mismanagement may lead to memory allocation failures.
- Program Stability: Impact on overall program stability.
- Programmer Responsibility: Demands a thorough understanding of memory management practices.
- Balancing Act: Striking a balance between flexibility and careful management is crucial.
- Prevention Measures: Responsible programming to prevent leaks and failures in dynamic memory allocation scenarios.
Blog Author Profile - Radhika Joshi
Radhika Joshi is a seasoned programming expert with a profound academic background in Computer Science and Machine Learning. Her dedication to the field has been fueled by her relentless pursuit of knowledge and her commitment to pushing the boundaries of technology. PhD in Computer Science from a prestigious university in the United States. Her doctoral research focused on cutting-edge advancements in advanced machine learning algorithms and techniques.