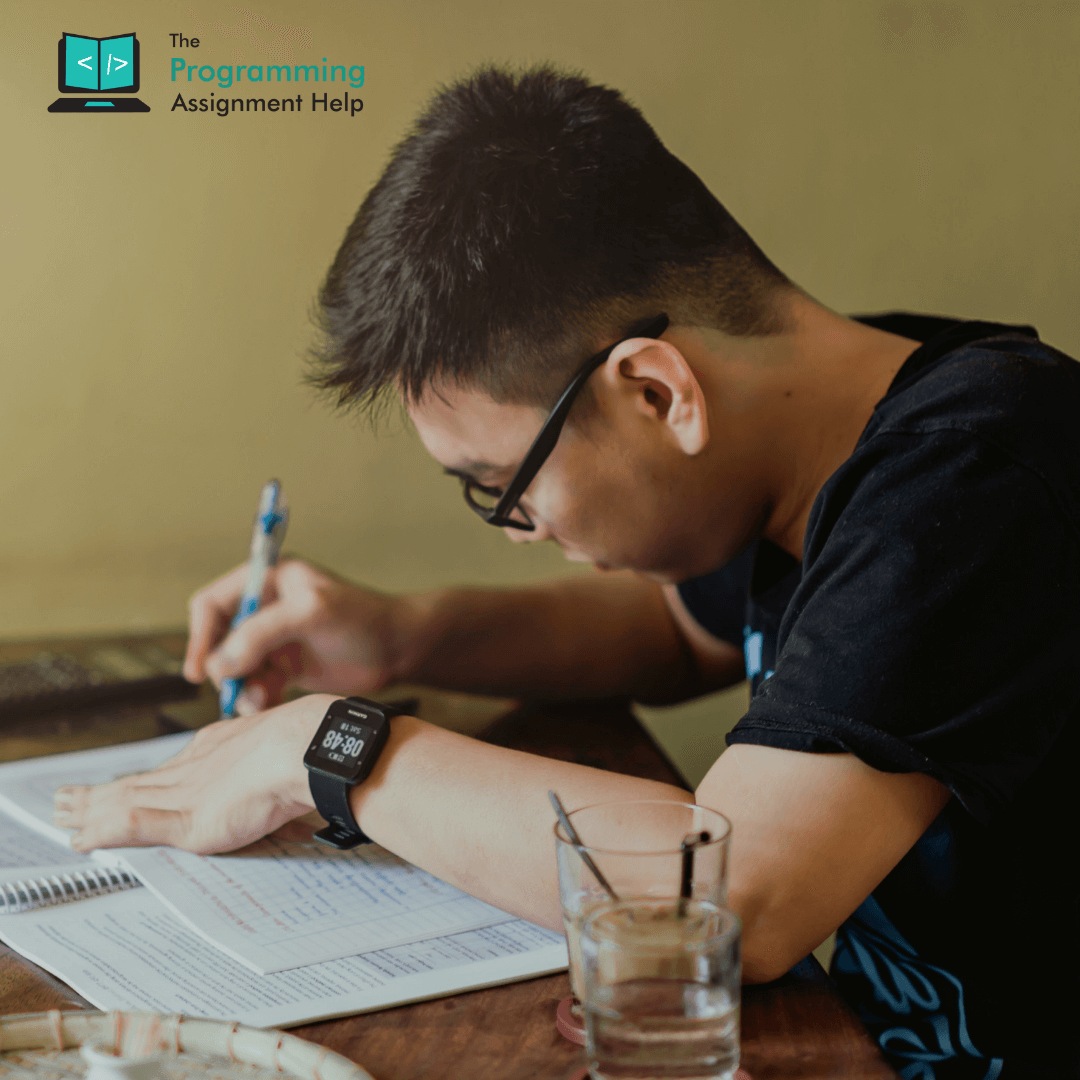
- 20th May 2022
- 04:22 am
In this program, we will cover three algorithms: Bubble Sort, Binary Search, and Factorial Calculation.
Python Assignment Solution - Program 1 - Bubble Sort Algorithm:
Bubble Sort is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
# Example usage:
data = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(data)
print("Sorted array:", data)
Python Homework Solution - Program 2 - Binary Search Algorithm
Binary Search is an efficient search algorithm that works on a sorted list by repeatedly dividing the search interval in half.
def binary_search(arr, target):
low, high = 0, len(arr) - 1
while low <= high:
mid = (low + high) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
low = mid + 1
else:
high = mid - 1
return -1
# Example usage:
data = [10, 25, 30, 40, 50, 60, 70, 80, 90]
target = 50
result = binary_search(data, target)
if result != -1:
print(f"Target {target} found at index {result}.")
else:
print(f"Target {target} not found in the list.")
do my Python Assignment - Program 3 - Factorial Calculation
A simple recursive function to calculate the factorial of a number.
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
# Example usage:
num = 5
result = factorial(num)
print(f"The factorial of {num} is {result}.")
These are some simple Python implementations of commonly used algorithms. Keep in mind that these examples are for educational purposes and might not be the most efficient or optimized implementations for large datasets. In practice, you would use built-in Python functions or libraries to handle sorting, searching, or other algorithmic tasks.
About The Author - Janhavi Gupta
Seasoned programmer with 6 years of experience in designing, developing, and deploying software solutions. Strong problem-solving skills coupled with the ability to collaborate effectively in multidisciplinary teams. Continuously learning and adapting to new technologies and methodologies to drive innovation and deliver high-quality, user-centric solutions.