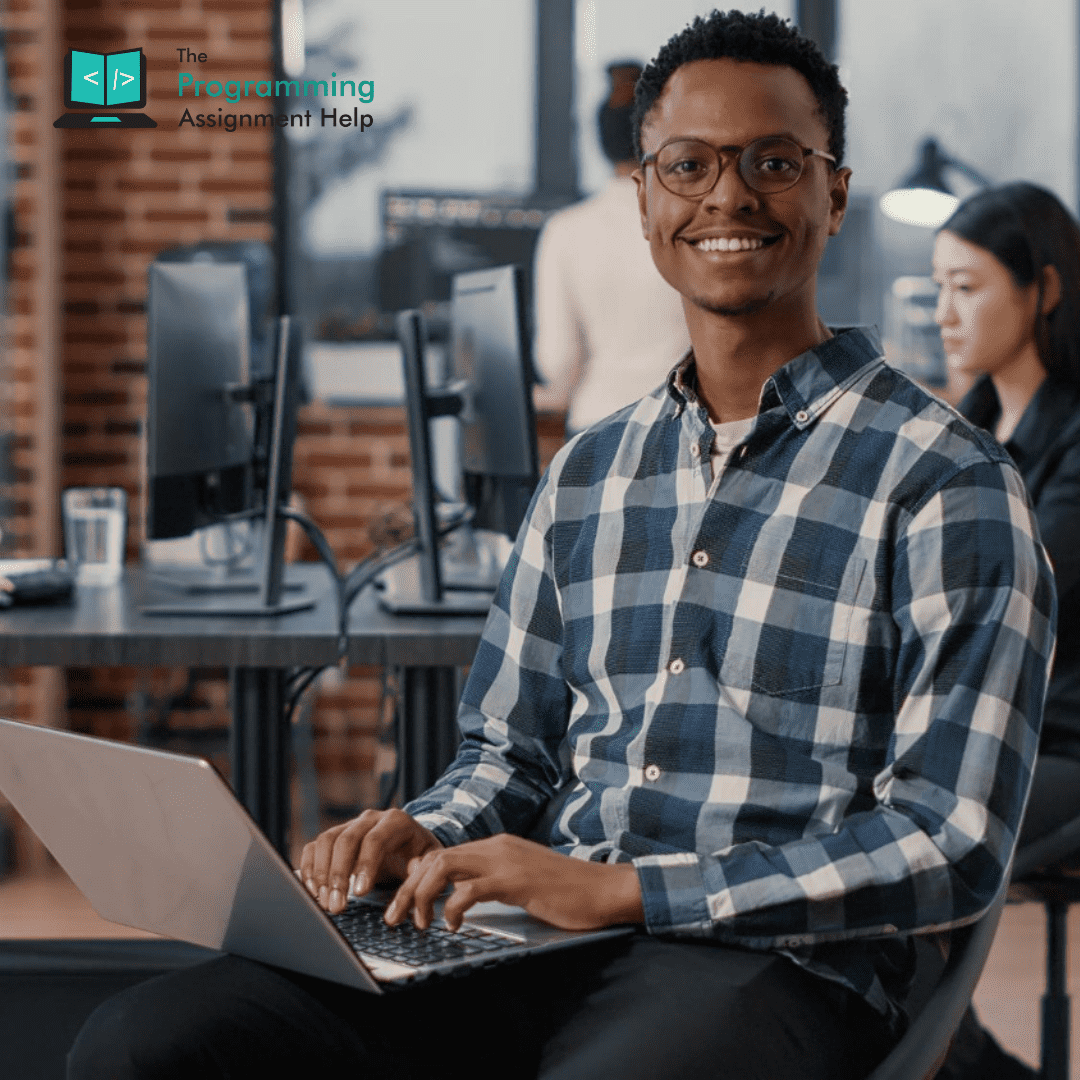
- 11th Nov 2022
- 03:44 am
Python Assignment Help - Python Solution on TicTacToe Game
import os
class Board:
#Constructor should initialize the board to a blank slate
def __init__(self):
self.board = [[' ' for j in range(3)] for i in range(3)] # 3x3 board initialized with ' '
#Should print out the board
def display(self):
print(" {} | {} | {}".format(self.board[0][0], self.board[0][1], self.board[0][2]))
print("------------")
print(" {} | {} | {}".format(self.board[1][0], self.board[1][1], self.board[1][2]))
print("------------")
print(" {} | {} | {}".format(self.board[2][0], self.board[2][1], self.board[2][2]))
#Method that updates a cell on the board based off the player
def update_cell(self, cell_no : str, player: str):
d = {
1 : (0, 0),
2 : (0, 1),
3 : (0, 2),
4 : (1, 0),
5 : (1, 1),
6 : (1, 2),
7 : (2, 0),
8 : (2, 1),
9 : (2, 2),
} # mapping of numbers to the tuple containing the corresponding row and column
x, y = d[cell_no]
if self.board[x][y] == ' ':
self.board[x][y] = player
#Determines if the Game has been won
def is_winner(self,player: str):
if (self.board[0][0] == self.board[0][1] == self.board[0][2] == player) or (self.board[1][0] == self.board[1][1] == self.board[1][2] == player) or (self.board[2][0] == self.board[2][1] == self.board[2][2] == player) or (self.board[0][0] == self.board[1][0] == self.board[2][0] == player) or (self.board[0][1] == self.board[1][1] == self.board[2][1] == player) or (self.board[0][2] == self.board[1][2] == self.board[2][2] == player) or (self.board[0][0] == self.board[1][1] == self.board[2][2] == player) or (self.board[0][2] == self.board[1][1] == self.board[2][0] == player):
return True # check for all possible ways to win
return False
#Checks if the game has been tied
def is_tie(self):
count = 0
for i in range(3):
for j in range(3):
count += self.board[i][j] == ' '
if (count == 0) and (not self.is_winner('X')) and (not self.is_winner('O')): # if board is not empty and neither of player wins then tie
return True
return False
#resets the game board for another round
def reset(self):
for i in range(3):
for j in range(3):
self.board[i][j] = ' ' # reset board