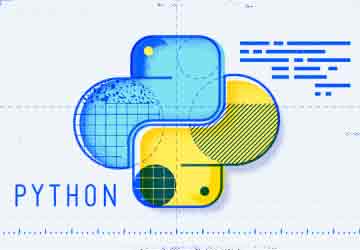
- 5th Jul 2019
- 04:55 am
- Adan Salman
Question 1 -
Write a program that will read in from the user a cubic polynomial f(x) (as a set of 4 coefficients), and use this to compute the derivative polynomial (i.e. compute the three coefficients of the derivative f’(x)). Then, read in a value for x from a user, and evaluate the derivative polynomial at that x.
Code -
A = float(input("Enter A coefficient: ")) B = float(input("Enter B coefficient: ")) C = float(input("Enter C coefficient: ")) D = float(input("Enter D coefficient: ")) x = float(input('Enter the value of x: ')) derivative = A * 3 * x**2 + B * 2 * x + C print("Derivative: ", derivative)
Question 2 -
Evaluating a polynomial derivative numerically
Code
A = float(input("Enter A coefficient: ")) B = float(input("Enter B coefficient: ")) C = float(input("Enter C coefficient: ")) D = float(input("Enter D coefficient: ")) x = float(input('Enter the value of x: ')) actual_derivative = A * 3 * x**2 + B * 2 * x + C a = 0.1 iterations = 0 value, prev_value = None, None while True: prev_value = value left = A * (x + a)**3 + B * (x + a)**2 + C * (x + a) + D right = A * x**3 + B * x**2 + C * x + D value = (left - right) / a iterations += 1 a = a / 2 if prev_value is not None: diff = abs(value - prev_value) if diff < 1e-6: break num_derivative = value print("Actual derivative: ", actual_derivative) print("Numerical derivative: ", num_derivative) print("Number of iterations: ", iterations)
Question 3 -
Evaluating a more complex function.
In your own code, come up with four more complex functions (not a polynomial – e.g. use sin/cos/tan/exp/log/powers/etc.), that you do not know how to compute the derivative for analytically, but that you can evaluate.
Python Code -
import math print('For a function y(x) = x**exp(-x)') x = float(input('Enter the value of x to compute the derivative: ')) a = 0.1 iterations = 0 value, prev_value = None, None while True: prev_value = value left = (x + a)**math.exp(-(x + a)) right = x**math.exp(-x) value = (left - right) / a iterations += 1 a = a / 2 if prev_value is not None: diff = abs(value - prev_value) if diff < 1e-6: break num_derivative = value print("Numerical derivative: ", num_derivative) print("Number of iterations: ", iterations) print('For a function y(x) = (exp(x) - exp(-x)) / (exp(x) + exp(-x))') x = float(input('Enter the value of x to compute the derivative: ')) a = 0.1 iterations = 0 value, prev_value = None, None while True: prev_value = value left = (math.exp(x + a) - math.exp(-(x + a))) / (math.exp(x + a) + math.exp(-(x + a))) right = (math.exp(x) - math.exp(-x)) / (math.exp(x) + math.exp(-x)) value = (left - right) / a iterations += 1 a = a / 2 if prev_value is not None: diff = abs(value - prev_value) if diff < 1e-6: break num_derivative = value print("Numerical derivative: ", num_derivative) print("Number of iterations: ", iterations) print('For a function y(x) = sin(log(x^2 + 1))') x = float(input('Enter the value of x to compute the derivative: ')) a = 0.1 iterations = 0 value, prev_value = None, None while True: prev_value = value left = math.sin(math.log((x + a)**2 + 1)) right = math.sin(math.log(x**2 + 1)) value = (left - right) / a iterations += 1 a = a / 2 if prev_value is not None: diff = abs(value - prev_value) if diff < 1e-6: break num_derivative = value print("Numerical derivative: ", num_derivative) print("Number of iterations: ", iterations) print('For a function y(x) = exp(-exp(exp(-x)))') x = float(input('Enter the value of x to compute the derivative: ')) a = 0.1 iterations = 0 value, prev_value = None, None while True: prev_value = value left = math.exp(-math.exp(math.exp(-(x + a)))) right = math.exp(-math.exp(math.exp(-x))) value = (left - right) / a iterations += 1 a = a / 2 if prev_value is not None: diff = abs(value - prev_value) if diff < 1e-6: break num_derivative = value print("Numerical derivative: ", num_derivative) print("Number of iterations: ", iterations)