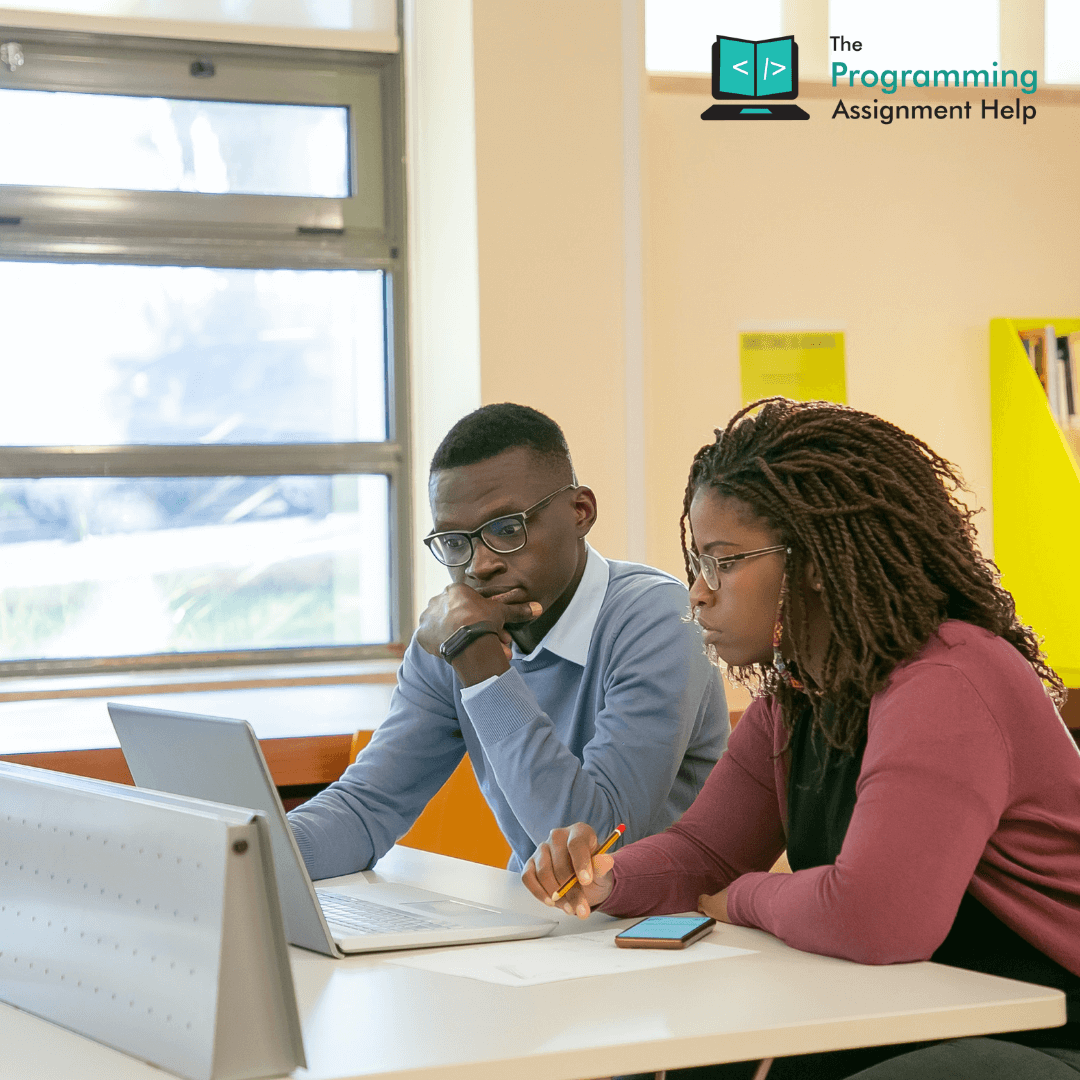
- 31st Mar 2022
- 02:35 am
The provided C++ code seems to implement a program for graph traversals using Depth-First Search (DFS) and Breadth-First Search (BFS). It appears that the graph data is stored in an external header file called "graph.hpp". The program allows the user to input a starting city (vertex) and performs both DFS and BFS traversals on the graph starting from that city. The user can then choose to try another city or exit the program. #include "graph.hpp" int main(){ int s; cout << "Graph Traversals" << endl << endl; cout << "Enter starting city using number from 0 - 11: "; cin >> s; cout << "You entered city name: "<< vertices[s] << endl << endl; dfs_num.assign(num_vertices, 0); vi dfs_vertices; dfs(s, dfs_vertices); cout << "Starting at " << vertices[s] << ", " << dfs_vertices.size() << " cities are searched in this Depth-First Search order:\n"; for(int vertex : dfs_vertices) cout << vertices[vertex] << ", "; cout << endl << endl; vi bfs_vertices; bfs(s, bfs_vertices); cout << "Starting at " << vertices[s] << ", " << bfs_vertices.size() << " cities are searched in this Breadth-First Search order:\n"; for(int vertex : bfs_vertices) cout << vertices[vertex] << ", "; cout << endl << endl; char ch; while(1){ cout << "Try another city (Y/N) "; cin >> ch; cout << endl; if(ch == 'N') break; cout << "Enter starting city using number from 0 - 11: "; cin >> s; cout << "You entered city name: "<< vertices[s] << endl << endl; dfs_num.assign(num_vertices, 0); dfs_vertices.clear(); dfs(s, dfs_vertices); cout << "Starting at " << vertices[s] << ", " << dfs_vertices.size() << " cities are searched in this Depth-First Search order:\n"; for(int vertex : dfs_vertices) cout << vertices[vertex] << ", "; cout << endl << endl; bfs_vertices.clear(); bfs(s, bfs_vertices); cout << "Starting at " << vertices[s] << ", " << bfs_vertices.size() << " cities are searched in this Breadth-First Search order:\n"; for(int vertex : bfs_vertices) cout << vertices[vertex] << ", "; cout << endl << endl; } return 0; }
Our Programming Assignment Help Expert's Comments on the Program
-
The user is prompted to enter a starting city (vertex) using a number from 0 to 11.
-
The Depth-First Search (DFS) is performed starting from the given city, and the visited vertices are stored in the
dfs_vertices
vector. -
The program then prints the order in which the cities were searched during DFS.
-
Next, the Breadth-First Search (BFS) is performed starting from the same city, and the visited vertices are stored in the
bfs_vertices
vector. -
The program prints the order in which the cities were searched during BFS.
-
The user is asked if they want to try another city. If they choose 'Y', the process repeats, allowing the user to input another starting city and performing DFS and BFS from that city.
-
If the user chooses 'N', the program exits.
It's important to note that the specific implementation of the graph and its traversals (DFS and BFS) are not provided in the code snippet. For the code to work correctly, you need to ensure that the necessary graph data structures and functions (dfs
and bfs
) are defined and included from the "graph.hpp" header file or other source files. Additionally, the global variables used in the code should be properly declared and initialized as well.