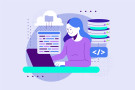
- 3rd Nov 2023
- 14:04 pm
Python is a flexible and powerful programming language renowned for its data manipulation and processing capabilities. In situations where you're dealing with nested lists or arrays, you might encounter the need to transform these multi-dimensional structures into a single, one-dimensional list. This operation is commonly referred to as "flattening" a list. In this comprehensive guide, we will delve into what Python Flatten List means, how it operates, various approaches for implementation, and offer code examples to efficiently achieve list flattening.
What is Python Flatten List?
The process of flattening a list in the Python programming language entails converting a multi-dimensional or nested list into a more straightforward, one-dimensional list. This process simplifies the complex nested structure, extracting individual elements from the nested lists and arranging them into a linear sequence.
The need for list flattening typically arises when working with hierarchical or nested Data structures. For example, consider a list of lists where each inner list represents a category and contains elements related to that category. The process of flattening this structure yields a unified list that encompasses all the constituents, hence enhancing convenience for a multitude of tasks.
The practice of flattening a list is a frequently employed procedure in the data processing. It presents various benefits, including the facilitation of iteration, searching, and manipulation of list members. It eliminates the need to navigate through multiple levels of nesting, significantly improving efficiency when working with intricate data structures in Python.
In Python, multiple methods are available for flattening a list, each tailored to specific use cases and offering unique advantages. These methods encompass a range of techniques, from utilizing list comprehensions and built-in functions to crafting custom recursive functions, all with the common goal of simplifying data manipulation in Python. Proficiency in flattening lists is a valuable skill for Python developers, particularly when handling complex, multi-dimensional data structures. It allows for more efficient and streamlined data processing, making it a critical skill in various Python applications.
How Does Python Flatten List Work?
The method of flattening a nested list in Python involves converting a multi-dimensional or nested list into a one-dimensional list. This simplifies the structure by extracting and combining the items. Python offers several techniques to achieve this, enhancing data processing efficiency and accessibility.
- List Comprehension: This method employs concise Python code to iterate through the nested list, extracting elements and forming a new one-dimensional list.
- Built-in Functions: Python provides functions like sum() and itertools.chain() for list flattening. The sum() function adds up elements in the nested list, effectively merging them. The itertools.chain() function concatenates sublists into a flattened list.
- Recursive Functions: Custom recursive functions can navigate nested lists, extracting and appending elements as they are encountered, accommodating varying nesting levels.
- Using Generators: Python's generators offer memory-efficient list flattening, yielding one element at a time and minimizing memory usage, making them ideal for large datasets.
Implementation of Python Flatten List
The process of flattening a list with levels in the Python programming language is considered a fundamental operation, and it can be executed using multiple approaches. Let's explore some of the most common techniques for flattening lists, along with their code implementations.
- Flatten List Using List Comprehension
List comprehensions offer a concise and readable method for flattening a list. These comprehensions enable you to efficiently iterate through the nested list, extracting each element along the way. The result is a new list that encompasses all the individual elements within a single dimension.
nested_list = [[1, 2], [3, [4, 5]], 6]
flattened_list = [item for sublist in nested_list for item in sublist]
# Output: [1, 2, 3, 4, 5, 6]
- Flatten List Using sum():
The nested list's components can be added to, thereby combining them into a single list, using the sum() method. Although simple, this approach might not be the most memory-efficient option for lengthy lists.
nested_list = [[1, 2], [3, [4, 5]], 6]
flattened_list = sum(nested_list, [])
# Output: [1, 2, 3, 4, 5, 6]
- Flatten List Using Loops
A loop-based approach involves iterating through the nested list and appending each element to a new list. This method offers control over the flattening process and is suitable for more complex scenarios.
nested_list = [[1, 2], [3, [4, 5]], 6]
flattened_list = []
for sublist in nested_list:
for item in sublist:
flattened_list.append(item)
# Output: [1, 2, 3, 4, 5, 6]
- Flatten List Using flatten() Method
The flatten() method is a custom function that uses recursion to flatten nested lists. It's versatile and can handle various levels of nesting.
def flatten(lst):
for item in lst:
if isinstance(item, list):
yield from flatten(item)
else:
yield item
nested_list = [[1, 2], [3, [4, 5]], 6]
flattened_list = list(flatten(nested_list))
# Output: [1, 2, 3, 4, 5, 6]
- Flatten List using chain() with isinstance():
The itertools.chain() function can concatenate the sublists, resulting in a flattened list. Combining it with the isinstance() function allows handling deeply nested lists.
from itertools import chain
nested_list = [[1, 2], [3, [4, 5]], 6]
flattened_list = list(chain.from_iterable(item if isinstance(item, list) else [item] for item in nested_list))
# Output: [1, 2, 3, 4, 5, 6]
- Flatten List using reduce() function:
The functools.reduce() function can be used to apply a flattening operation cumulatively to the elements of the nested list.
from functools import reduce
from operator import add
nested_list = [[1, 2], [3, [4, 5]], 6]
flattened_list = reduce(add, nested_list)
# Output: [1, 2, 3, 4, 5, 6]