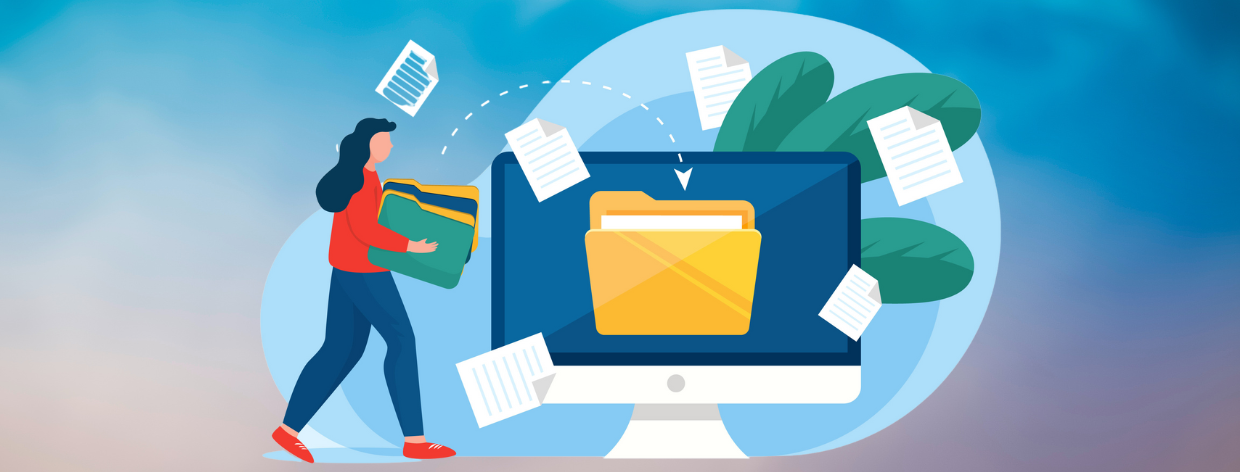
- 22nd Feb 2024
- 22:44 pm
- Adan Salman
Navigating the digital landscape often involves interacting with files and folders. In the realm of Python, adeptly manipulating these elements is crucial for tasks ranging from data analysis to automating workflows. We will blog delves into the essential Python functions for accessing and managing files and directories, empowering you to unlock their potential.
Why Mastering File Management Matters:
From organizing research data to streamlining web development, file and directory operations lie at the heart of many Python projects. Whether you're building a file organizer, automating data cleaning, or crafting web applications, understanding how to access, list, filter, and manipulate files is key.
Common Use Cases for "get-files-directory-python" Functions:
- Data Analysis and Processing: Listing files for analysis, filtering based on specific criteria, and accessing specific data points within files.
- Automating Tasks: Scheduling backups, renaming files in bulk, or organizing downloaded files based on specific rules.
- Web Development: Serving static files like images and CSS, handling user uploads, and managing site content.
- Machine Learning: Preparing data for training by accessing, filtering, and organizing training and testing datasets.
What You'll Learn:
We serve as your comprehensive guide to the "get-files-directory-python" toolkit. We'll explore:
- Essential functions: Unveiling the powerhouses like os.listdir(), glob.glob(), and os.walk(), explaining their functionalities and intricacies.
- Advanced techniques: Delving into handling hidden files, filtering based on complex criteria, and optimizing performance for large datasets.
- Practical applications: Putting theory into action with real-world examples like listing files for backup, searching for specific files, and organizing files into directories.
Ready to conquer file and directory management in Python? Dive into this guide and unlock the power of "get-files-directory-python" functions. Don't get stuck at a hurdle - get expert Python assignment help and Python homework help just a click away!
Essential Methods and Functions
Within the vibrant ecosystem of Python programming, effectively manipulating files and directories forms the cornerstone of many powerful applications. From data analysis to automation tasks, a firm grasp of these functionalities becomes paramount. We will delves into the essential methods and functions that equip you to confidently navigate the file system and manage your digital assets with precision.
- os.listdir():
The os.listdir() function retrieves a list of filenames within a specified directory. It's a simple yet powerful tool for generating basic directory listings. However, remember that it only reveals the immediate contents, excluding nested directories and hidden files.
import os
directory_path = "my_files"
filenames = os.listdir(directory_path)
print(filenames)
# Output: ['file1.txt', 'file2.csv', 'image.jpg']
While convenient, acknowledge its limitations before venturing into complex directory structures or hidden file operations.
- glob.glob():
Need to find specific files based on patterns? glob.glob() empowers you with wildcards, representing characters or groups of characters, to efficiently target files matching your defined criteria.
import glob
# Find all .txt files
text_files = glob.glob("*.txt")
print(text_files) # Output: ['file1.txt']
# Find files starting with "image"
image_files = glob.glob("image*")
print(image_files) # Output: ['image.jpg']
# Use custom patterns with brackets
specific_file = glob.glob("file[12].*")
print(specific_file)
# Output: ['file2.csv']
Remember, while effective for targeted retrieval, globbing can be resource-intensive for large directories due to extensive searches. Use it judiciously.
- os.walk():
For traversing intricate directory hierarchies, os.walk() is your guide. It yields a stream of tuples, each containing the current directory path, its subdirectories, and the filenames within that directory.
import os
for root, dirs, files in os.walk("my_files"):
for file in files:
print(os.path.join(root, file)) # Print full path of each file
# Filtering within the walk
for root, dirs, files in os.walk("my_files"):
if root.endswith("data"): # Filter directories ending with "data"
for file in files:
if file.endswith(".csv"): # Filter files ending with ".csv"
print(os.path.join(root, file))
While os.walk() allows for comprehensive exploration, extensive directory trees can consume considerable memory. Exercise caution with massive structures.
- pathlib module:
Beyond these core functions, the pathlib module beckons for those seeking a more object-oriented approach. It offers path objects representing files and directories, providing a cleaner and more platform-independent way to interact with the file system.
from pathlib import Path
path = Path("my_files")
for file in path.iterdir(): # List files in the directory
print(file)
# Access file attributes directly
print(file.name) # Print filename
print(file.exists()) # Check if file exists
While offering distinct advantages, it might require additional learning for those already comfortable with the core functions discussed earlier.
Advanced Techniques for File Management in Python
Having mastered the fundamentals of file and directory manipulation in Python, you're ready to delve into advanced techniques that unlock greater control and efficiency. This guide explores essential considerations for navigating intricate scenarios while ensuring robust and optimized file operations.
Handling Hidden Files:
Hidden files, shrouded in secrecy, often hold crucial system data or user preferences. While their obscurity provides security, accessing them requires special attention. On Linux and macOS, files prefixed with a dot (.) are typically considered hidden. The os.listdir() function won't reveal them by default, but you can leverage the glob.glob() function with .* to list all files, including hidden ones. Remember, modifying system-critical hidden files can have unintended consequences, so proceed with caution.
Precision Through Filtering:
Filtering files based on specific criteria is essential for efficient data manipulation. Regular expressions, powerful tools for pattern matching, offer granular control. You can filter based on filenames, extensions, or even content within the files. Libraries like re provide the necessary functions to craft intricate patterns.
import re
# Filter files by extension
text_files = [f for f in os.listdir() if f.endswith(".txt")]
# Filter files by modification date
recent_files = [f for f in os.listdir() if os.path.getmtime(f) > time.time() - 86400] # Last 24 hours
# Use regular expressions for complex filtering
pattern = re.compile(r"data_\d{8}_\d+.csv") # Match files starting with "data_", followed by 8 digits and ".csv"
filtered_files = [f for f in os.listdir() if pattern.match(f)]
Custom functions can also be used for complex filtering logic based on file size, content, or other criteria.
Error Handling:
File operations are inherently prone to errors like permission issues, invalid paths, or nonexistent files. Graceful error handling ensures the robustness of your code and prevents unexpected crashes. The try-except block is your shield against these potential pitfalls.
try:
with open("myfile.txt", "r") as file:
data = file.read()
except FileNotFoundError:
print("File not found!")
except PermissionError:
print("Insufficient permissions to access the file.")
Always anticipate potential errors and implement appropriate handling mechanisms to maintain code stability.
Optimizing Performance:
In large projects, file operations can become performance bottlenecks. Here are some strategies to keep your code nimble:
- Caching: Store frequently accessed data in memory to avoid repetitive file reads.
- Avoiding unnecessary walks: Instead of walking the entire directory structure, use targeted functions like os.path.exists() to check specific file existence.
- Using generators: Generators yield data on demand, reducing memory consumption compared to loading everything in memory at once.
By understanding and implementing these optimization techniques, you can significantly enhance the performance of your file-intensive applications.
Applications of Get Files Directory in Python
The ability to access, manipulate, and organize files and directories forms the bedrock of countless Python applications. Whether you're building data pipelines, automating tasks, or crafting web applications, mastering file management empowers you to interact effectively with the digital world. Let's delve into some key applications where "get files directory" functions become your invaluable allies:
Data Analysis and Processing:
- Data Ingestion: Use os.listdir() to list files in a specific directory, then glob.glob() to filter based on extensions (e.g., .csv, .txt).
- Data Cleaning and Transformation: Walk through directory structures with os.walk() and apply custom logic to clean file contents or convert formats.
- Exploratory Data Analysis: Filter files based on specific criteria (e.g., size, date) using regular expressions or custom functions within os.walk().
Automation and Task Management:
- File Backups: Schedule regular backups using os.listdir() to list files and shutil.copy() to copy them to a backup location.
- Bulk File Renaming: Rename files based on patterns or criteria within os.listdir() or os.walk() using string manipulation techniques.
- Log File Processing: Use os.walk() to access log files across directories, filter based on timestamps, and extract relevant information.
- Serving Static Files: Use os.path.join() to construct paths to static files like images or CSS based on user requests.
- Handling User Uploads: Validate and store uploaded files securely, utilizing directory manipulation functions to create appropriate folders.
- Content Management System (CMS): Navigate through directory structures to manage website content, using os.path.getmtime() to track file modifications.
Machine Learning and Artificial Intelligence:
- Data Preparation: Organize and preprocess training and testing datasets using directory manipulation functions to ensure proper data access.
- Model Output Management: Save model outputs (e.g., predictions) to specific directories based on run configurations.
- Experiment Tracking: Track experiment results by organizing log files and model artifacts in a structured manner using directory manipulations.