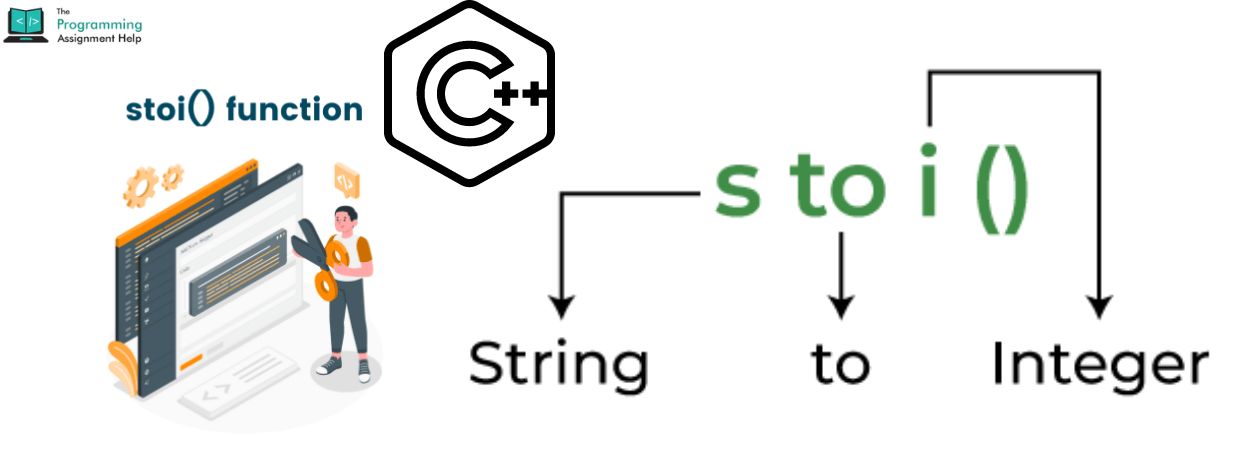
- 30th Sep 2024
- 18:40 pm
- Nia Sharma
The stoi function in C++ is a built-in utility designed to convert strings into integers. It stands for "string to integer" and is part of the header file. This function simplifies handling numeric values when they are stored or represented as strings, making it incredibly useful in a variety of programming tasks, such as processing user input, reading data from files, or parsing numerical information from strings.
How Does the stoi Function Work?
The stoi function takes a string as input and returns the corresponding integer value. If the string contains valid numeric characters, the function will perform the conversion successfully. For instance:
cpp
string numStr = "456";
int num = stoi(numStr); // num becomes 456
In this example, the string `"456"` is turned into the integer `456`. The `stoi` function also allows you to add an optional second parameter that lets you choose where in the string to start the conversion. There’s also a third optional parameter you can use to specify the base for the conversion, like decimal or hexadecimal, though it defaults to decimal if you don’t specify.
Error Handling
One of the standout features of stoi is its error-handling capabilities. If the string cannot be converted into an integer, the function throws exceptions. Two common exceptions include:
invalid_argument: This is thrown when the string doesn’t represent a valid number (e.g., "abc").
out_of_range: This is thrown when the string represents a number that is too large to fit in an integer data type.
Why Use stoi?
The stoi function is reliable, easy to use, and saves time compared to manually converting strings into integers using loops or other methods. Whether you're working on competitive programming, data analysis, or general software development, stoi helps streamline operations involving string-to-integer conversions.
At The Programming Assignment Help, we provide detailed resources and expert guidance on mastering functions like stoi in C++. Understanding such built-in functions can boost your efficiency and enhance your coding skills. Happy coding!