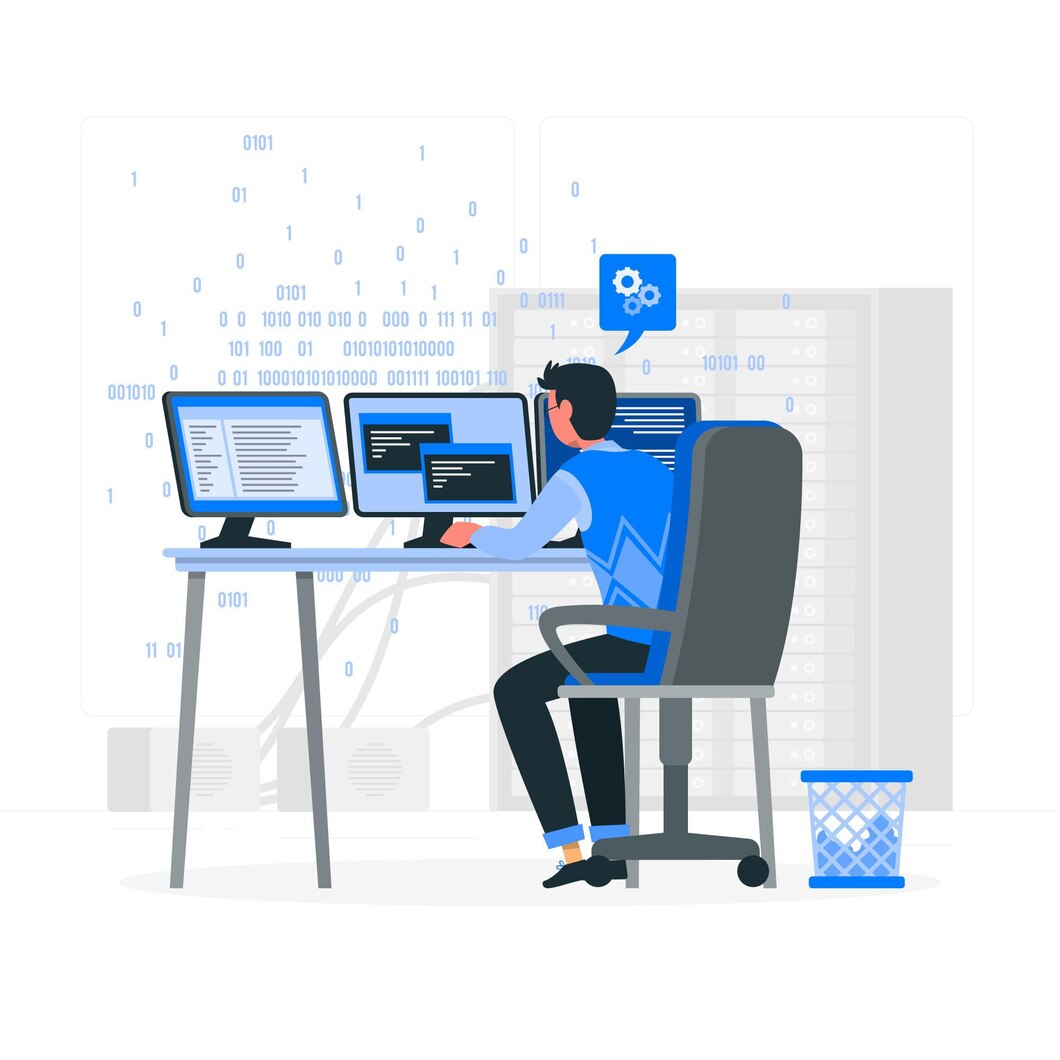
- 12th Dec 2023
- 22:54 pm
- Admin
Time Series Analysis in R includes the study, modelling, and forecasting of time-varying data. It is frequently utilised in a variety of sectors including finance, economics, environmental science, and others. R has a rich ecosystem of packages and functions that are specifically developed for time series analysis, making it a popular choice for time series researchers and analysts.
Important Steps in Time Series Analysis with R:
- Data Exploration: Begin by loading the time series data into R and investigating its properties. Look for trends, seasonality, and any obvious patterns. To get insights, use visualisations such as line graphs and seasonal decompositions.
- Time Series Decomposition: Divide the time series into its constituents, typically trend, seasonality, and remainder (residuals). R's 'decompose()' function and packages such as 'forecast' can be used for this.
- Testing for Stationarity: Many time series models are based on stationarity, which means that statistical features such as mean and variance remain constant across time. To examine and transform the data, run stationarity tests with routines like 'adf.test()' or 'kpss.test()'.
- Model Identification: Select a suitable model based on the time series characteristics. Autoregressive Integrated Moving Average (ARIMA), Seasonal-Trend decomposition using LOESS (STL), and Exponential Smoothing State Space Models (ETS) are examples of popular models.
- Model Fitting: Use routines such as 'auto.arima()' or 'ets()' to automatically pick and fit the selected model to time series data. Model diagnostics and goodness-of-fit tests aid in evaluating model performance.
- Forecasting: Use the fitted model to generate forecasts. Point forecasts and prediction intervals are provided via functions such as 'forecast()'. Visualisation techniques such as 'autoplot()' make it simple to compare anticipated numbers to past data.
- Model Evaluation: Determine the forecast's accuracy by comparing it to actual data. Model performance can be evaluated using metrics such as Mean Absolute Error (MAE), Mean Squared Error (MSE), or predicted error distributions.
- Time Series Visualisation: R provides several time series visualisation tools, such as interactive charts, seasonal decomposition plots, and time series heatmaps. Libraries such as 'ggplot2' and 'dygraphs' make it easier to create visually beautiful and useful visualisations.
Popular R Time Series Analysis Packages:
R offers a complete set of tools for time series analysis, allowing analysts and researchers to successfully analyse, model, and forecast temporal data. R is a versatile and favoured language for time series analysis jobs due to its extensive packages, statistical models, and visualisation features.
- 'forecast': Offers time series forecasting services such as automatic ARIMA modelling, ETS models, and numerous forecast evaluation measures.
- 'xts' and 'zoo': Provide specialised data structures for processing time series data, making manipulation and analysis easier.
- 'TSA': Uses time series analysis techniques such as ARIMA and seasonal decomposition.
- 'ggplot2' and 'dygraphs': This software is widely used to create visually beautiful and interactive time series plots.
Use cases of Time Series Analysis in R Programming
Time Series Analysis in R is used for a variety of purposes across industries and areas. Here are a few major scenarios in which R is regularly used for time series analysis:
- Financial Prediction: R is often used in finance to forecast stock prices, currency exchange rates, and other financial indices. ARIMA and GARCH time series models assist financial analysts in making informed decisions based on past market data.
- Economic Indicators: Time series analysis is critical for tracking and forecasting economic indicators like GDP growth, inflation rates, and unemployment rates. R is used by economists to create models that capture trends and cyclical patterns in economic time series data.
- Energy Consumption and Production: Energy analysts analyse time series data related to energy consumption and production using R. Predicting power demand, optimising energy usage, and measuring the impact of renewable energy sources on the system are all part of this.
- Healthcare and Epidemiology: In healthcare, time series analysis is used to predict patient admission rates, track disease outbreaks, and assess the impact of interventions. R is useful for developing models that estimate patient loads and efficiently allocate resources.
- Climate and Environmental Science: Environmental scientists use R to analyse time series data from climate systems such as temperature patterns, rainfall trends, and sea-level fluctuations. This study helps to comprehend long-term climate fluctuations and forecast future environmental situations.
- Sales and Demand Forecasting: Companies use time series analysis to forecast sales and demand patterns, allowing for better inventory management and supply chain optimisation. R forecasting tools like 'forecast' and 'prophet' are popular for these applications.
- Analytics for Web and Social Media: In online analytics and social media monitoring, time series analysis is critical. Analysts use R to analyse website traffic, user engagement, and social media patterns over time, assisting organisations in making data-driven marketing decisions.
- Production and Quality Control: R is used in manufacturing to monitor and regulate production operations. Time series analysis aids in the identification of patterns, detection of abnormalities, and optimisation of production schedules to assure product quality.
- Telecom: Telecommunications businesses utilise time series analysis to estimate network traffic, diagnose network breakdowns or disturbances, and optimise resource allocation. R makes it easier to create models for effective network management.
- Human Resources: In human resources, time series analysis is used to estimate labour need, analyse employee performance trends, and predict attrition rates. This assists HR professionals in making strategic talent management decisions.
These examples demonstrate the adaptability of time series analysis in R across a wide range of topics. Analysts and data scientists may extract useful insights from temporal data by leveraging R's broad ecosystem of packages and tools, contributing to better decision-making and more effective planning in a variety of industries.
Step-by-step method to perform Time Series Analysis in R Programming
Time series analysis in R entails numerous critical processes, ranging from data exploration to model construction and evaluation. A step-by-step approach with a simple sample dataset is provided below. We'll utilise the built-in 'AirPassengers' dataset in this example, which contains monthly airline passenger counts.
- Step 1: Load the necessary libraries and datasets
```
# Load required libraries
library(tidyverse)
library(forecast)
# Load the AirPassengers dataset
data("AirPassengers")
```
- Step 2: Explore the Dataset
```
# View the first few rows of the dataset
head(AirPassengers)
# Check the structure of the dataset
str(AirPassengers)
# Plot the time series data
plot(AirPassengers, main = "Airline Passenger Counts Over Time", xlab = "Year", ylab = "Passenger Count")
```
- Step 3: Time Series Decomposition
Decompose the time series into its components (trend, seasonality, and residuals).
```
# Decompose the time series
decomp_result <- decompose(AirPassengers)
# Plot the decomposition
plot(decomp_result)
```
- Step 4: Stationarity Testing
Check for stationarity using statistical tests. In this example, we'll use the Augmented Dickey-Fuller test.
```
# Augmented Dickey-Fuller test for stationarity
adf_test <- adf.test(AirPassengers)
print(adf_test)
```
- Step 5: Differencing (if needed)
If the time series is not stationary, apply differencing to make it stationary.
```
# Differencing to achieve stationarity
diff_series <- diff(AirPassengers)
# Plot differenced series
plot(diff_series, main = "Differenced Time Series", xlab = "Year", ylab = "Differenced Passenger Count")
```
- Step 6: Model Identification and Fitting
Identify an appropriate time series model (e.g., ARIMA) based on the characteristics of the data and fit the model.
```
# Automatic ARIMA model selection and fitting
arima_model <- auto.arima(AirPassengers)
print(arima_model)
```
- Step 7: Model Diagnostics
Check the diagnostics of the fitted model to ensure its adequacy.
```
# Model diagnostics
checkresiduals(arima_model)
```
Step 8: Forecasting
Generate forecasts using the fitted model.
```
# Forecasting with the ARIMA model
forecast_result <- forecast(arima_model, h = 12) # Forecasting for the next 12 months
print(forecast_result)
# Plot the forecast
autoplot(forecast_result, main = "ARIMA Forecast for Airline Passenger Counts")
```
- Step 9: Model Evaluation
Evaluate the accuracy of the forecast by comparing it to actual values.
```
# Calculate forecast accuracy metrics
accuracy(forecast_result)
```
These steps lay the groundwork for time series analysis in R. Additional strategies, such as seasonal adjustment, model tuning, and more advanced forecasting algorithms, may be used depending on the data properties. Time series analysis in R is a vibrant subject with numerous packages and tools to meet a variety of modelling requirements.